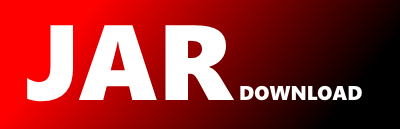
com.nimbusds.jose.jwk.loader.PKCS11Utils Maven / Gradle / Ivy
package com.nimbusds.jose.jwk.loader;
import java.security.KeyStore;
import java.security.Provider;
import com.nimbusds.jose.jwk.JWK;
import com.nimbusds.jose.jwk.JWKSet;
/**
* PKCS#11 utilities.
*/
public class PKCS11Utils {
/**
* Returns {@code true} if the specified JSON Web Key (JWK) is backed
* by a PKCS#11 key store.
*
* @param jwk The JWK. Must not be {@code null}.
*
* @return {@code true} if the JWK is backed by a PKCS#11 key store,
* else {@code false}.
*/
public static boolean isPKCS11Key(final JWK jwk) {
KeyStore keyStore = jwk.getKeyStore();
if (keyStore == null) {
return false;
}
Provider provider = keyStore.getProvider();
if (provider == null) {
return false;
}
return provider.getName().contains("PKCS11");
}
/**
* Returns {@code true} if the specified JSON Web Key (JWK) set
* contains at least one key backed by a PKCS#11 key store.
*
* @param jwkSet The JWK set. Must not be {@code null}.
*
* @return {@code true} if the JWK set contains at least one key that
* is backed by a PKCS#11 key store, else {@code false}.
*/
public static boolean hasPKCS11Key(final JWKSet jwkSet) {
for (JWK jwk: jwkSet.getKeys()) {
if (isPKCS11Key(jwk)) {
return true;
}
}
return false;
}
/**
* Prevents public instantiation.
*/
private PKCS11Utils() { }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy