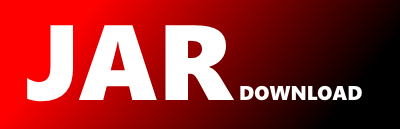
com.nimbusds.jose.jwk.loader.X509CertExpirationComparator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nimbus-jwkset-loader Show documentation
Show all versions of nimbus-jwkset-loader Show documentation
JWK set loader with PKCS#11 support for Hardware Security Modules (HSM)
and smart cards.
package com.nimbusds.jose.jwk.loader;
import java.security.cert.X509Certificate;
import java.util.Comparator;
import java.util.Date;
import com.nimbusds.jose.jwk.JWK;
import com.nimbusds.jose.util.Base64;
import com.nimbusds.jose.util.X509CertUtils;
import net.jcip.annotations.ThreadSafe;
/**
* Comparator of X.509 certificates by their expiration time.
*
*
* exp now < exp now + 1
* JWK without x5c < JWK with x5c
*
*/
@ThreadSafe
public class X509CertExpirationComparator implements Comparator {
@Override
public int compare(final JWK a, final JWK b) {
// Extract 1st cert from chain
final Base64 certDerEncoded_a;
if (a.getX509CertChain() != null && a.getX509CertChain().size() >= 1) {
certDerEncoded_a = a.getX509CertChain().get(0);
} else {
certDerEncoded_a = null;
}
final Base64 certDerEncoded_b;
if (b.getX509CertChain() != null && b.getX509CertChain().size() >= 1) {
certDerEncoded_b = b.getX509CertChain().get(0);
} else {
certDerEncoded_b = null;
}
if (certDerEncoded_a == null && certDerEncoded_b == null) {
// No certs -> a == b
return 0;
}
if (certDerEncoded_a == null && certDerEncoded_b != null) {
// a < b
return -1;
}
if (certDerEncoded_a != null && certDerEncoded_b == null) {
// a > b
return 1;
}
// Compare cert issue dates
X509Certificate cert_a = X509CertUtils.parse(certDerEncoded_a.decode());
if (cert_a == null) {
throw new IllegalArgumentException("Invalid X.509 certificate for JWK with ID " + a.getKeyID());
}
X509Certificate cert_b = X509CertUtils.parse(certDerEncoded_b.decode());
if (cert_b == null) {
throw new IllegalArgumentException("Invalid X.509 certificate for JWK with ID " + b.getKeyID());
}
Date exp_a = cert_a.getNotAfter();
if (exp_a == null) {
throw new IllegalArgumentException("Missing expiration date in X.509 certificate for JWK with ID " + a.getKeyID());
}
Date exp_b = cert_b.getNotAfter();
if (exp_b == null) {
throw new IllegalArgumentException("Missing expiration date in X.509 certificate for JWK with ID " + b.getKeyID());
}
// a == b: 0
// a > b: 1
// a < b: 0
return exp_a.compareTo(exp_b);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy