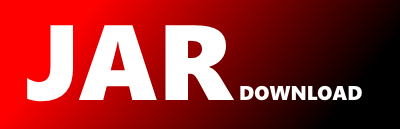
com.nimbusds.openid.connect.provider.spi.reg.statement.ScopeRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of software-statement-verifier Show documentation
Show all versions of software-statement-verifier Show documentation
Software statement verifier for the Connect2id server
package com.nimbusds.openid.connect.provider.spi.reg.statement;
import java.util.Collection;
import java.util.StringJoiner;
import com.jayway.jsonpath.JsonPath;
import org.json.JSONArray;
import com.nimbusds.oauth2.sdk.Scope;
/**
* Scope rule.
*/
public class ScopeRule {
/**
* Filters a requested scope for registration according to a set of
* rules.
*
* @param requestedScope The requested scope, {@code null} if not
* specified.
* @param scopeRules The scope rules, empty set if none.
* @param json The software statement claims JSON. Must not
* be {@code null}.
*
* @return The filtered allowed scopes, empty if none or not specified.
*/
public static Scope filter(final Scope requestedScope,
final Collection scopeRules,
final String json) {
if (requestedScope == null || requestedScope.isEmpty()) {
return new Scope();
}
var allowedScopes = new Scope();
for (ScopeRule rule: scopeRules) {
if (rule.matches(json)) {
allowedScopes.addAll(rule.getScope());
}
}
var out = new Scope(requestedScope);
out.retainAll(allowedScopes);
return out;
}
/**
* The scope values.
*/
private final Scope scope;
/**
* The matching JSON path for the scope.
*/
private final String jsonPath;
/**
* Creates a new scope setting rule.
*
* @param scope The scope values. Must not be empty or {@code null}.
* @param jsonPath The matching JSON path for the scope values. Must
* not be {@code null} or blank.
*/
public ScopeRule(final Scope scope, final String jsonPath) {
if (scope == null || scope.isEmpty()) {
throw new IllegalArgumentException("The scope must not empty or null");
}
this.scope = scope;
if (jsonPath == null || jsonPath.isBlank()) {
throw new IllegalArgumentException("The JSON path must not be blank or null");
}
this.jsonPath = jsonPath;
}
/**
* Returns the scope values.
*
* @return The scope values.
*/
public Scope getScope() {
return scope;
}
/**
* Returns the matching JSON path.
*
* @return The matching JSON path.
*/
public String getJSONPath() {
return jsonPath;
}
/**
* Returns {@code true} if the JSON path query returns one or more
* items for the specified JSON.
*
* @param json The JSON. Must not be {@code null}.
*
* @return {@code true} if the JSON path matches, else {@code false}.
*/
public boolean matches(final String json) {
JSONArray matches = JsonPath.read(json, jsonPath);
return ! matches.isEmpty();
}
@Override
public String toString() {
return new StringJoiner(", ", "[", "]")
.add("scope='" + scope + "'")
.add("jsonPath='" + jsonPath + "'")
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy