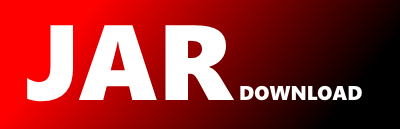
org.jruby.ir.util.DirectedGraph Maven / Gradle / Ivy
package org.jruby.ir.util;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
/**
* Meant to be single-threaded. More work on whole impl if not.
*/
public class DirectedGraph {
private Map> vertices = new HashMap>();
private Set> edges = new HashSet>();
private ArrayList inOrderVerticeData = new ArrayList();
int vertexIDCounter = 0;
public Collection> vertices() {
return vertices.values();
}
public Collection> edges() {
return edges;
}
public Iterable> edgesOfType(Object type) {
return new EdgeTypeIterable(edges, type);
}
public Collection allData() {
return vertices.keySet();
}
/**
* @return data in the order it was added to this graph.
*/
public Collection getInorderData() {
return inOrderVerticeData;
}
public void addEdge(T source, T destination, Object type) {
vertexFor(source).addEdgeTo(destination, type);
}
public void removeEdge(Edge edge) {
edge.getSource().removeEdgeTo(edge.getDestination());
}
public void removeEdge(T source, T destination) {
for (Edge edge: vertexFor(source).getOutgoingEdges()) {
if (edge.getDestination().getData() == destination) {
vertexFor(source).removeEdgeTo(edge.getDestination());
return;
}
}
}
public Vertex findVertexFor(T data) {
return vertices.get(data);
}
public Vertex vertexFor(T data) {
Vertex vertex = vertices.get(data);
if (vertex != null) return vertex;
vertex = new Vertex(this, data, vertexIDCounter++);
inOrderVerticeData.add(data);
vertices.put(data, vertex);
return vertex;
}
public void removeVertexFor(T data) {
Vertex vertex = vertexFor(data);
vertices.remove(data);
inOrderVerticeData.remove(data);
vertex.removeAllEdges();
}
/**
* @return the number of vertices in the graph.
*/
public int size() {
return allData().size();
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder();
ArrayList> verts = new ArrayList>(vertices.values());
Collections.sort(verts);
for (Vertex vertex: verts) {
buf.append(vertex);
}
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy