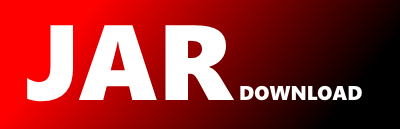
com.ning.compress.lzf.LZFOutputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compress-lzf Show documentation
Show all versions of compress-lzf Show documentation
Compression codec for LZF encoding for particularly encoding/decoding, with reasonable compression.
Compressor is basic Lempel-Ziv codec, without Huffman (deflate/gzip) or statistical post-encoding.
See "http://oldhome.schmorp.de/marc/liblzf.html" for more on original LZF package.
package com.ning.compress.lzf;
import java.io.IOException;
import java.io.OutputStream;
public class LZFOutputStream extends OutputStream
{
private static int OUTPUT_BUFFER_SIZE = LZFChunk.MAX_CHUNK_LEN;
protected final OutputStream outputStream;
protected byte[] outputBuffer = new byte[OUTPUT_BUFFER_SIZE];
protected int position = 0;
public LZFOutputStream(final OutputStream outputStream)
{
this.outputStream = outputStream;
}
@Override
public void write(final int singleByte) throws IOException
{
if(position >= outputBuffer.length) {
writeCompressedBlock();
}
outputBuffer[position++] = (byte) singleByte;
}
@Override
public void write(final byte[] buffer, final int offset, final int length) throws IOException
{
int inputCursor = offset;
int remainingBytes = length;
while(remainingBytes > 0) {
if(position >= outputBuffer.length) {
writeCompressedBlock();
}
int chunkLength = (remainingBytes > (outputBuffer.length - position))?outputBuffer.length - position: remainingBytes;
System.arraycopy(buffer, inputCursor, outputBuffer, position, chunkLength);
position += chunkLength;
remainingBytes -= chunkLength;
inputCursor += chunkLength;
}
}
@Override
public void flush() throws IOException
{
writeCompressedBlock();
outputStream.flush();
}
@Override
public void close() throws IOException
{
try {
flush();
} finally {
outputStream.close();
}
}
/**
* Compress and write the current block to the OutputStream
*/
private void writeCompressedBlock() throws IOException
{
if (position > 0) {
final byte[] compressedBytes = LZFEncoder.encode(outputBuffer, position);
outputStream.write(compressedBytes);
position = 0;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy