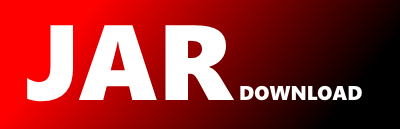
com.nitorcreations.matchers.ComponentMatchers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wicket-matchers Show documentation
Show all versions of wicket-matchers Show documentation
Useful Hamcrest 1.3 matchers for Wicket 6 projects
The newest version!
package com.nitorcreations.matchers;
import java.util.List;
import org.hamcrest.Description;
import org.hamcrest.Factory;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeDiagnosingMatcher;
import org.apache.wicket.Component;
import org.apache.wicket.Page;
import org.apache.wicket.markup.html.basic.MultiLineLabel;
import org.apache.wicket.markup.html.link.BookmarkablePageLink;
import org.apache.wicket.markup.html.list.ListView;
import org.apache.wicket.request.mapper.parameter.PageParameters;
import static org.hamcrest.Matchers.allOf;
import static org.hamcrest.Matchers.equalTo;
import static org.hamcrest.Matchers.hasProperty;
import static org.hamcrest.Matchers.instanceOf;
import static org.hamcrest.Matchers.is;
public class ComponentMatchers {
@Factory
public static Matcher hasId(String id) {
return hasId(equalTo(id));
}
@Factory
public static Matcher hasId(Matcher idMatcher) {
return hasProperty("id", idMatcher);
}
/**
* Matches that the component is visible in hierarchy.
*
* @return matcher for component visibility
* @see {@link Component#isVisibleInHierarchy()}
*/
@Factory
public static Matcher visibleInHierarchy() {
return new TypeSafeDiagnosingMatcher() {
@Override
public void describeTo(Description description) {
description.appendText("visible");
}
@Override
protected boolean matchesSafely(T item, Description mismatchDescription) {
if (!item.isVisibleInHierarchy()) {
mismatchDescription.appendText("visible: ").appendValue(item.isVisibleInHierarchy());
return false;
}
return true;
}
};
}
/**
* Matches that the component is enabled in hierarchy.
*
* @return matcher for component enabled status
* @see {@link Component#isEnabledInHierarchy()}
*/
@Factory
public static Matcher enabledInHierarchy() {
return new TypeSafeDiagnosingMatcher() {
@Override
protected boolean matchesSafely(T item, Description mismatchDescription) {
if (!item.isEnabledInHierarchy()) {
mismatchDescription.appendText("enabled: ").appendValue(item.isEnabledInHierarchy());
return false;
}
return true;
}
@Override
public void describeTo(Description description) {
description.appendText("enabled");
}
};
}
/**
* Matches a {@link org.apache.wicket.markup.html.basic.Label} with the given model object.
*
* @param value
* the matcher for the model object
* @return matcher for label
*/
@Factory
public static Matcher label(final Matcher> value) {
return new TypeSafeDiagnosingMatcher() {
@Override
protected boolean matchesSafely(T item, Description mismatchDescription) {
if (!value.matches(item.getDefaultModelObjectAsString())) {
mismatchDescription.appendText("label ");
value.describeMismatch(item.getDefaultModelObjectAsString(), mismatchDescription);
return false;
}
return true;
}
@Override
public void describeTo(Description description) {
description.appendText("label ").appendDescriptionOf(value);
}
};
}
/** @see #label(org.hamcrest.Matcher) */
@Factory
public static Matcher label(String value) {
return label(is(value));
}
/**
* Matches a component whose {@link org.apache.wicket.Component#getDefaultModelObject()} matches
* the given matcher {@code m}.
* @param m the matcher to match the model object against.
* @return the matcher
*/
@Factory
public static Matcher hasModelObject(Matcher> m) {
return hasProperty("defaultModelObject", m);
}
/** @see #hasModelObject(org.hamcrest.Matcher) */
@Factory
public static Matcher hasModelObject(Object o) {
return hasModelObject(is(o));
}
/**
* Matches list view whose {@link org.apache.wicket.markup.html.list.ListView#getList()} matches the given value
* matcher.
*
* @param items
* the value matcher
* @return matcher for {@link org.apache.wicket.markup.html.list.ListView}
*/
@SuppressWarnings("unchecked")
@Factory
public static Matcher listViewThat(final Matcher> items) {
return (Matcher) new TypeSafeDiagnosingMatcher>() {
@Override
protected boolean matchesSafely(ListView> item, Description mismatchDescription) {
List> list = item.getList();
items.describeMismatch(list, mismatchDescription);
return items.matches(list);
}
@Override
public void describeTo(Description description) {
description.appendText("ListView with items ").appendDescriptionOf(items);
}
};
}
/**
* Matches a {@link org.apache.wicket.markup.html.basic.MultiLineLabel} with the given
* default model object.
* @param value the value to match
*/
@SuppressWarnings("unchecked")
@Factory
public static Matcher multiLineLabel(final Matcher value) {
return (Matcher) new TypeSafeDiagnosingMatcher() {
@Override
protected boolean matchesSafely(MultiLineLabel item, Description mismatchDescription) {
value.describeMismatch(item, mismatchDescription);
return value.matches(item.getDefaultModelObjectAsString());
}
@Override
public void describeTo(Description description) {
description.appendText("multiline label: ").appendDescriptionOf(value);
}
};
}
/** @see #multiLineLabel(org.hamcrest.Matcher) */
@Factory
public static Matcher multiLineLabel(final String value) {
return multiLineLabel(is(value));
}
@Factory
public static Matcher required() {
return allOf(instanceOf(Component.class), hasProperty("required", is(true)));
}
@Factory
public static Matcher bookmarkableLink(Class clazz) {
return allOf(
instanceOf(BookmarkablePageLink.class),
hasProperty("pageClass", equalTo(clazz))
);
}
@Factory
public static Matcher bookmarkableLink(Class clazz, PageParameters params) {
return allOf(
instanceOf(BookmarkablePageLink.class),
hasProperty("pageClass", equalTo(clazz)),
hasProperty("pageParameters", equalTo(params))
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy