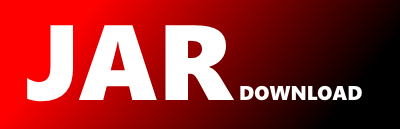
com.nitorcreations.willow.metrics.FullMessageMultiseriesMetric Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of willow-servers Show documentation
Show all versions of willow-servers Show documentation
Willow operational servlets and servers
The newest version!
package com.nitorcreations.willow.metrics;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import com.nitorcreations.willow.messages.AbstractMessage;
import com.nitorcreations.willow.messages.metrics.MetricConfig;
public abstract class FullMessageMultiseriesMetric, Y extends Comparable> extends FullMessageMetric>>{
@Override
public Collection> processData(long start, long stop, int step, MetricConfig conf) {
int len = (int) ((stop - start) / step) + 1;
Map> ret = new LinkedHashMap>();
if (rawData.isEmpty()) {
return ret.values();
}
List retTimes = new ArrayList();
long curr = start;
for (int i = 0; i < len; i++) {
retTimes.add(curr);
curr += step;
}
for (Long nextTime : retTimes) {
long afterNextTime = nextTime + 1;
Collection preceeding = rawData.headMap(afterNextTime).values();
rawData = rawData.tailMap(afterNextTime);
List tmplist = new ArrayList(preceeding);
addValue(ret, tmplist, nextTime.longValue(), step, conf);
}
fillMissingValues(ret, retTimes, step);
return ret.values();
}
protected abstract void addValue(Map> values, List preeceding, long stepTime, int stepLen, MetricConfig conf);
@SuppressWarnings("unchecked")
protected void fillMissingValues(Map> ret, List retTimes, int stepLen) {
for (SeriesData nextValues : ret.values()) {
Map valueMap = nextValues.pointsAsMap();
List addX = new ArrayList<>();
for (Long nextStep : retTimes) {
if (!valueMap.containsKey(nextStep)) {
addX.add(nextStep);
}
}
Object zero = 0;
for (Long nextAdd : addX) {
for (int i = 0; i < nextValues.values.size(); i++) {
if (nextValues.values.get(i).x.compareTo((X) nextAdd) > 0) {
Point toAdd = new Point<>();
toAdd.x = (X) nextAdd;
toAdd.y = (Y) zero;
nextValues.values.add(i, toAdd);
break;
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy