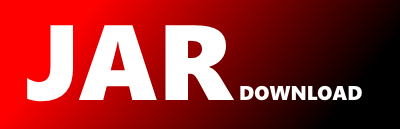
com.nitorcreations.willow.metrics.SimpleMetric Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of willow-servers Show documentation
Show all versions of willow-servers Show documentation
Willow operational servlets and servers
The newest version!
package com.nitorcreations.willow.metrics;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.search.SearchHit;
import org.elasticsearch.search.SearchHitField;
import com.nitorcreations.willow.messages.metrics.MetricConfig;
import com.nitorcreations.willow.messages.metrics.TimePoint;
public abstract class SimpleMetric extends AbstractMetric {
protected SortedMap rawData = new TreeMap();
private Map fields;
@Override
public abstract String getType();
public abstract String[] requiresFields();
protected void readResponse(SearchResponse response) {
for (SearchHit next : response.getHits().getHits()) {
fields = next.getFields();
Long timestamp = fields.get("timestamp").value();
List nextData = new ArrayList<>();
for (String nextField : requiresFields()) {
if (fields.get(nextField) == null) {
break;
}
nextData.add((T) fields.get(nextField).value());
}
if (nextData.size() == requiresFields().length) {
rawData.put(timestamp, getValue(nextData));
}
}
}
@SuppressWarnings("unchecked")
protected L getValue(List arr) {
return (L) arr.get(0);
}
@Override
public List> calculateMetric(MetricConfig conf) {
List reqFields = new ArrayList();
reqFields.add("timestamp");
reqFields.addAll(Arrays.asList(requiresFields()));
SearchResponse response = executeQuery(client, conf, getType(), reqFields);
readResponse(response);
int len = (int) ((conf.getStop() - conf.getStart()) / conf.getStep()) + 1;
List> ret = new ArrayList>();
if (rawData.isEmpty()) {
return ret;
}
List retTimes = new ArrayList();
long curr = conf.getStart();
for (int i = 0; i < len; i++) {
retTimes.add(Long.valueOf(curr));
curr += conf.getStep();
}
for (Long nextTime : retTimes) {
long afterNextTime = nextTime + 1;
Collection preceeding = rawData.headMap(afterNextTime).values();
rawData = rawData.tailMap(afterNextTime);
List tmplist = new ArrayList(preceeding);
if (tmplist.isEmpty()) {
ret.add(new TimePoint(nextTime.longValue(), fillMissingValue()));
continue;
}
ret.add(new TimePoint(nextTime.longValue(), estimateValue(tmplist, nextTime, conf.getStep(), conf)));
}
return ret;
}
protected L estimateValue(List preceeding, long stepTime, long stepLen, MetricConfig conf) {
return preceeding.get(preceeding.size() - 1);
}
protected abstract L fillMissingValue();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy