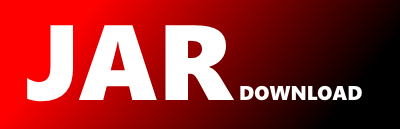
com.noenv.wiremongo.MemoryStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-wiremongo Show documentation
Show all versions of vertx-wiremongo Show documentation
Lightweight mongo mocking for Vert.x
package com.noenv.wiremongo;
import io.vertx.codegen.annotations.Nullable;
import io.vertx.core.Handler;
import io.vertx.core.streams.ReadStream;
import java.util.*;
import java.util.stream.Collectors;
// ToDo: proper support toFlowable() toObservable()
public class MemoryStream implements ReadStream {
private final Queue items = new LinkedList<>();
private final Throwable error;
private Handler endHandler;
private Handler dataHandler;
boolean flowingMode = true;
private MemoryStream(final Collection items, final Throwable error) {
this.items.addAll(items);
this.error = error;
}
public MemoryStream copy(final java.util.function.Function copyItem) {
return new MemoryStream<>(items.stream().map(copyItem).collect(Collectors.toList()), error);
}
@Override
public ReadStream exceptionHandler(Handler handler) {
if (error != null) {
handler.handle(error);
}
return this;
}
@Override
public ReadStream handler(@Nullable Handler handler) {
this.dataHandler = handler;
if (dataHandler != null && flowingMode) {
while (!items.isEmpty()) {
dataHandler.handle(items.remove());
}
if (endHandler != null) {
endHandler.handle(null);
}
}
return this;
}
@Override
public ReadStream pause() {
flowingMode = false;
return this;
}
@Override
public ReadStream resume() {
flowingMode = true;
fetch(Long.MAX_VALUE);
return this;
}
@Override
public ReadStream fetch(long amount) {
long counter = 0;
while (counter < amount && !this.items.isEmpty()) {
dataHandler.handle(this.items.remove());
counter++;
}
if (this.items.isEmpty() && endHandler != null) {
endHandler.handle(null);
}
return this;
}
@Override
public ReadStream endHandler(@Nullable Handler endHandler) {
this.endHandler = endHandler;
if (this.items.isEmpty()) {
endHandler.handle(null);
}
return this;
}
public static ReadStream of(T... items) {
return new MemoryStream<>(Arrays.asList(items), null);
}
public static ReadStream fromList(List list) {
return new MemoryStream<>(list, null);
}
public static ReadStream error(Throwable error) {
return new MemoryStream<>(Collections.emptyList(), error);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy