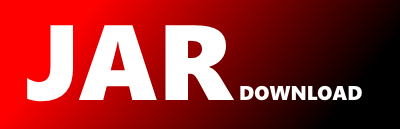
com.norconex.commons.lang.encrypt.EncryptionUtil Maven / Gradle / Ivy
Show all versions of norconex-commons-lang Show documentation
/* Copyright 2015-2016 Norconex Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.norconex.commons.lang.encrypt;
import java.io.PrintStream;
import java.nio.charset.StandardCharsets;
import java.security.spec.AlgorithmParameterSpec;
import java.security.spec.KeySpec;
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.PBEKeySpec;
import javax.crypto.spec.PBEParameterSpec;
import javax.xml.bind.DatatypeConverter;
import com.norconex.commons.lang.encrypt.EncryptionKey.Source;
/**
* Simplified encryption and decryption methods using the
* "PBEWithMD5AndDES" algorithm with a supplied encryption key (which you
* can also think of as a passphrase, or password).
* The "salt" and iteration count used by this class are hard-coded. To have
* more control and ensure a more secure approach, you should rely on another
* implementation or create your own.
*
*
* To use on the command prompt, use the following command to print usage
* options:
*
*
* java -cp norconex-commons-lang-[version].jar com.norconex.commons.lang.encrypt.EncryptionUtil
*
*
* For example, to use a encryption key store in a file to encrypt some text,
* add the following arguments to the above command:
*
*
* <above_command> encrypt -f "/path/to/key.txt" "Encrypt this text"
*
*
* @author Pascal Essiembre
* @since 1.9.0
*/
public class EncryptionUtil {
private EncryptionUtil() {
super();
}
public static void main(String[] args) {
if (args.length != 4) {
printUsage();
}
String cmdArg = args[0];
String typeArg = args[1];
String keyArg = args[2];
String textArg = args[3];
Source type = null;
if ("-k".equalsIgnoreCase(typeArg)) {
type = Source.KEY;
} else if ("-f".equalsIgnoreCase(typeArg)) {
type = Source.FILE;
} else if ("-e".equalsIgnoreCase(typeArg)) {
type = Source.ENVIRONMENT;
} else if ("-p".equalsIgnoreCase(typeArg)) {
type = Source.PROPERTY;
} else {
System.err.println("Unsupported type of key: " + type);
printUsage();
}
EncryptionKey key = new EncryptionKey(keyArg, type);
if ("encrypt".equalsIgnoreCase(cmdArg)) {
System.out.println(encrypt(textArg, key));
} else if ("decrypt".equalsIgnoreCase(cmdArg)) {
System.out.println(decrypt(textArg, key));
} else {
System.err.println("Unsupported command: " + cmdArg);
printUsage();
}
System.exit(0);
}
private static void printUsage() {
PrintStream out = System.out;
out.println(" encrypt|decrypt -k|-f|-e key text");
out.println();
out.println("Where:");
out.println(" encrypt encrypt the text with the given key");
out.println(" decrypt decrypt the text with the given key");
out.println(" -k key is the encryption key");
out.println(" -f key is the file containing the encryption key");
out.println(" -e key is the environment variable holding the "
+ "encryption key");
out.println(" -p key is the system property holding the "
+ "encryption key");
out.println(" key the encryption key (or file, or env. "
+ "variable, etc.)");
out.println(" text text to encrypt or decrypt");
System.exit(-1);
}
/**
* Encrypts the given text with the encryption key supplied. If the
* encryption key is null
or resolves to blank key,
* the text to encrypt will be returned unmodified.
* @param textToEncrypt text to be encrypted
* @param encryptionKey encryption key which must resolve to the same
* value to encrypt and decrypt the supplied text.
* @return encrypted text or null
if
* textToEncrypt
is null
.
*/
public static String encrypt(
String textToEncrypt, EncryptionKey encryptionKey) {
if (textToEncrypt == null) {
return null;
}
if (encryptionKey == null) {
return textToEncrypt;
}
String key = encryptionKey.resolve();
if (key == null) {
return textToEncrypt;
}
// 8-byte Salt
byte[] salt = {
(byte)0xE3, (byte)0x03, (byte)0x9B, (byte)0xA9,
(byte)0xC8, (byte)0x16, (byte)0x35, (byte)0x56
};
// Iteration count
int iterationCount = 19;
Cipher ecipher;
try {
// Create the key
KeySpec keySpec = new PBEKeySpec(
key.trim().toCharArray(), salt, iterationCount);
SecretKey secretKey = SecretKeyFactory.getInstance(
"PBEWithMD5AndDES").generateSecret(keySpec);
ecipher = Cipher.getInstance(secretKey.getAlgorithm());
// Prepare the parameter to the ciphers
AlgorithmParameterSpec paramSpec =
new PBEParameterSpec(salt, iterationCount);
// Create the ciphers
ecipher.init(Cipher.ENCRYPT_MODE, secretKey, paramSpec);
byte[] utf8 = textToEncrypt.trim().getBytes(StandardCharsets.UTF_8);
byte[] enc = ecipher.doFinal(utf8);
return DatatypeConverter.printBase64Binary(enc);
} catch (Exception e) {
throw new EncryptionException("Encryption failed.", e);
}
}
/**
* Decrypts the given encrypted text with the encryption key supplied.
*
* @param encryptedText text to be decrypted
* @param encryptionKey encryption key which must resolve to the same
* value to encrypt and decrypt the supplied text.
* @return decrypted text or null
if one of
* encryptedText
or key
is null
.
*/
public static String decrypt(
String encryptedText, EncryptionKey encryptionKey) {
if (encryptedText == null) {
return null;
}
if (encryptionKey == null) {
return encryptedText;
}
String key = encryptionKey.resolve();
if (key == null) {
return encryptedText;
}
// 8-byte Salt
byte[] salt = {
(byte)0xE3, (byte)0x03, (byte)0x9B, (byte)0xA9,
(byte)0xC8, (byte)0x16, (byte)0x35, (byte)0x56
};
// Iteration count
int iterationCount = 19;
Cipher dcipher;
try {
// Create the key
KeySpec keySpec = new PBEKeySpec(
key.trim().toCharArray(), salt, iterationCount);
SecretKey secretKey = SecretKeyFactory.getInstance(
"PBEWithMD5AndDES").generateSecret(keySpec);
dcipher = Cipher.getInstance(secretKey.getAlgorithm());
// Prepare the parameter to the ciphers
AlgorithmParameterSpec paramSpec =
new PBEParameterSpec(salt, iterationCount);
// Create the ciphers
dcipher.init(Cipher.DECRYPT_MODE, secretKey, paramSpec);
byte[] dec =
DatatypeConverter.parseBase64Binary(encryptedText.trim());
byte[] utf8 = dcipher.doFinal(dec);
return new String(utf8, StandardCharsets.UTF_8);
} catch (Exception e) {
throw new EncryptionException("Decryption failed.", e);
}
}
}