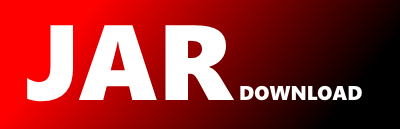
com.nordstrom.automation.junit.Run Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of junit-foundation Show documentation
Show all versions of junit-foundation Show documentation
This is the foundation framework for JUnit automation
package com.nordstrom.automation.junit;
import java.util.ArrayDeque;
import java.util.Deque;
import java.util.List;
import java.util.Map;
import java.util.ServiceLoader;
import java.util.Set;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.CopyOnWriteArraySet;
import org.junit.runner.Description;
import org.junit.runner.notification.RunListener;
import org.junit.runner.notification.RunNotifier;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.Optional;
import net.bytebuddy.implementation.bind.annotation.Argument;
import net.bytebuddy.implementation.bind.annotation.SuperCall;
import net.bytebuddy.implementation.bind.annotation.This;
/**
* This class declares the interceptor for the {@link org.junit.runners.ParentRunner#run run} method.
*/
@SuppressWarnings("squid:S1118")
public class Run {
private static final ThreadLocal> runnerStack;
private static final Set startNotified = new CopyOnWriteArraySet<>();
private static final Set finishNotified = new CopyOnWriteArraySet<>();
private static final ServiceLoader runListenerLoader;
private static final ServiceLoader runnerWatcherLoader;
private static final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy