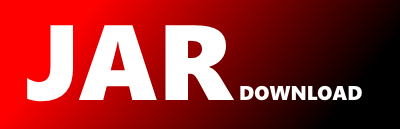
com.nordstrom.automation.junit.CreateTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of junit-foundation Show documentation
Show all versions of junit-foundation Show documentation
This is the foundation framework for JUnit automation
package com.nordstrom.automation.junit;
import java.util.Map;
import java.util.ServiceLoader;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentHashMap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.Optional;
import net.bytebuddy.implementation.bind.annotation.RuntimeType;
import net.bytebuddy.implementation.bind.annotation.SuperCall;
import net.bytebuddy.implementation.bind.annotation.This;
/**
* This class declares the interceptor for the {@link org.junit.runners.BlockJUnit4ClassRunner#createTest
* createTest} method.
*/
@SuppressWarnings("squid:S1118")
public class CreateTest {
private static final ServiceLoader objectWatcherLoader;
private static final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy