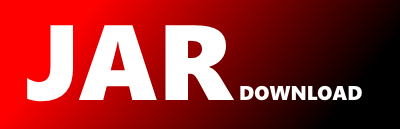
com.nordstrom.automation.junit.RunReflectiveCall Maven / Gradle / Ivy
package com.nordstrom.automation.junit;
import static com.nordstrom.automation.junit.LifecycleHooks.getFieldValue;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.ServiceLoader;
import java.util.concurrent.Callable;
import org.junit.After;
import org.junit.AfterClass;
import org.junit.Before;
import org.junit.BeforeClass;
import org.junit.Test;
import org.junit.runners.model.FrameworkMethod;
import com.nordstrom.common.base.UncheckedThrow;
import net.bytebuddy.implementation.bind.annotation.RuntimeType;
import net.bytebuddy.implementation.bind.annotation.SuperCall;
import net.bytebuddy.implementation.bind.annotation.This;
/**
* This class declares the interceptor for the {@link org.junit.internal.runners.model.ReflectiveCallable#runReflectiveCall
* runReflectiveCall} method.
*/
@SuppressWarnings("squid:S1118")
public class RunReflectiveCall {
private static final ServiceLoader methodWatcherLoader;
private static final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy