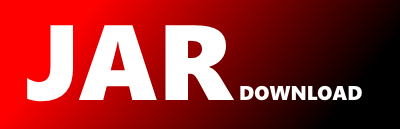
norswap.autumn.SideEffectingArrayStack Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of autumn Show documentation
Show all versions of autumn Show documentation
A parser combinator library
The newest version!
package norswap.autumn;
import norswap.autumn.util.ArrayStack;
import norswap.utils.data.wrappers.Slot;
import java.util.ArrayList;
import java.util.function.IntFunction;
/**
* A stack in which some mutating operations produce side-effecting results, namely:
*
*
* - {@link #push(Object)}
* - {@link #pop()}
* - {@link #pop(int)}
* - {@link #popFrom(int)}
*
*
* The stack should only be mutated through these operations, or it won't be safe
* to use during a parser!
*
*
A side-effecting operation is one where a {@link SideEffect.Applied} is pushed onto {@link
* Parse#log} to represent a state mutation, enabling it to be undone in case of parser
* backtracking.
*
*
Norswap's note: in the long run it would be good if we overrode every single mutating method
* of {@link ArrayStack} and {@link ArrayList} and made them side-effecting. For now, it will have
* to wait.
*/
public final class SideEffectingArrayStack extends ArrayStack
© 2015 - 2025 Weber Informatics LLC | Privacy Policy