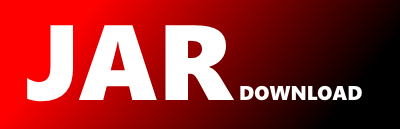
edu.uci.ics.jung.io.GraphMLWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jung-io Show documentation
Show all versions of jung-io Show documentation
IO support classes for JUNG
The newest version!
/*
* Created on June 16, 2008
*
* Copyright (c) 2008, The JUNG Authors
*
* All rights reserved.
*
* This software is open-source under the BSD license; see either
* "license.txt" or
* https://github.com/jrtom/jung/blob/master/LICENSE for a description.
*/
package edu.uci.ics.jung.io;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.Writer;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import com.google.common.base.Function;
import com.google.common.base.Functions;
import edu.uci.ics.jung.graph.Graph;
import edu.uci.ics.jung.graph.Hypergraph;
import edu.uci.ics.jung.graph.UndirectedGraph;
import edu.uci.ics.jung.graph.util.EdgeType;
import edu.uci.ics.jung.graph.util.Pair;
/**
* Writes graphs out in GraphML format.
*
* Current known issues:
*
* - Only supports one graph per output file.
*
- Does not indent lines for text-format readability.
*
*
*/
public class GraphMLWriter
{
protected Function super V, String> vertex_ids;
protected Function super E, String> edge_ids;
protected Map>> graph_data;
protected Map> vertex_data;
protected Map> edge_data;
protected Function super V, String> vertex_desc;
protected Function super E, String> edge_desc;
protected Function super Hypergraph, String> graph_desc;
protected boolean directed;
protected int nest_level;
public GraphMLWriter()
{
vertex_ids = new Function()
{
public String apply(V v)
{
return v.toString();
}
};
edge_ids = Functions.constant(null);
graph_data = Collections.emptyMap();
vertex_data = Collections.emptyMap();
edge_data = Collections.emptyMap();
vertex_desc = Functions.constant(null);
edge_desc = Functions.constant(null);
graph_desc = Functions.constant(null);
nest_level = 0;
}
/**
* Writes {@code graph} out using {@code w}.
* @param graph the graph to write out
* @param w the writer instance to which the graph data will be written out
* @throws IOException if writing the graph fails
*/
public void save(Hypergraph graph, Writer w) throws IOException
{
BufferedWriter bw = new BufferedWriter(w);
// write out boilerplate header
bw.write("\n");
bw.write("\n");
// write out data specifiers, including defaults
for (String key : graph_data.keySet())
writeKeySpecification(key, "graph", graph_data.get(key), bw);
for (String key : vertex_data.keySet())
writeKeySpecification(key, "node", vertex_data.get(key), bw);
for (String key : edge_data.keySet())
writeKeySpecification(key, "edge", edge_data.get(key), bw);
// write out graph-level information
// set edge default direction
bw.write("\n");
else
bw.write("undirected\">\n");
// write graph description, if any
String desc = graph_desc.apply(graph);
if (desc != null)
bw.write("" + desc + " \n");
// write graph data out if any
for (String key : graph_data.keySet())
{
Function, ?> t = graph_data.get(key).transformer;
Object value = t.apply(graph);
if (value != null)
bw.write(format("data", "key", key, value.toString()) + "\n");
}
// write vertex information
writeVertexData(graph, bw);
// write edge information
writeEdgeData(graph, bw);
// close graph
bw.write(" \n");
bw.write(" \n");
bw.flush();
bw.close();
}
// public boolean save(Collection> graphs, Writer w)
// {
// return true;
// }
protected void writeIndentedText(BufferedWriter w, String to_write) throws IOException
{
for (int i = 0; i < nest_level; i++)
w.write(" ");
w.write(to_write);
}
protected void writeVertexData(Hypergraph graph, BufferedWriter w) throws IOException
{
for (V v: graph.getVertices())
{
String v_string = String.format("\n");
closed = true;
w.write("" + desc + " \n");
}
// write data out if any
for (String key : vertex_data.keySet())
{
Function t = vertex_data.get(key).transformer;
if (t != null)
{
Object value = t.apply(v);
if (value != null)
{
if (!closed)
{
w.write(v_string + ">\n");
closed = true;
}
w.write(format("data", "key", key, value.toString()) + "\n");
}
}
}
if (!closed)
w.write(v_string + "/>\n"); // no contents; close the node with "/>"
else
w.write(" \n");
}
}
protected void writeEdgeData(Hypergraph g, Writer w) throws IOException
{
for (E e: g.getEdges())
{
Collection vertices = g.getIncidentVertices(e);
String id = edge_ids.apply(e);
String e_string;
boolean is_hyperedge = !(g instanceof Graph);
if (is_hyperedge)
{
e_string = " endpoints = new Pair(vertices);
V v1 = endpoints.getFirst();
V v2 = endpoints.getSecond();
e_string = "\n");
closed = true;
w.write("" + desc + " \n");
}
// write data out if any
for (String key : edge_data.keySet())
{
Function t = edge_data.get(key).transformer;
Object value = t.apply(e);
if (value != null)
{
if (!closed)
{
w.write(e_string + ">\n");
closed = true;
}
w.write(format("data", "key", key, value.toString()) + "\n");
}
}
// if this is a hyperedge, write endpoints out if any
if (is_hyperedge)
{
for (V v : vertices)
{
if (!closed)
{
w.write(e_string + ">\n");
closed = true;
}
w.write(" \n");
}
}
if (!closed)
w.write(e_string + "/>\n"); // no contents; close the edge with "/>"
else
if (is_hyperedge)
w.write(" \n");
else
w.write("\n");
}
}
protected void writeKeySpecification(String key, String type,
GraphMLMetadata> ds, BufferedWriter bw) throws IOException
{
bw.write("\n");
closed = true;
}
bw.write("" + desc + " \n");
}
// write out default if any
Object def = ds.default_value;
if (def != null)
{
if (!closed)
{
bw.write(">\n");
closed = true;
}
bw.write("" + def.toString() + " \n");
}
if (!closed)
bw.write("/>\n");
else
bw.write(" \n");
}
protected String format(String type, String attr, String value, String contents)
{
return String.format("<%s %s=\"%s\">%s%s>",
type, attr, value, contents, type);
}
/**
* Provides an ID that will be used to identify a vertex in the output file.
* If the vertex IDs are not set, the ID for each vertex will default to
* the output of toString
* (and thus not guaranteed to be unique).
*
* @param vertex_ids a mapping from vertex to ID
*/
public void setVertexIDs(Function vertex_ids)
{
this.vertex_ids = vertex_ids;
}
/**
* Provides an ID that will be used to identify an edge in the output file.
* If any edge ID is missing, no ID will be written out for the
* corresponding edge.
*
* @param edge_ids a mapping from edge to ID
*/
public void setEdgeIDs(Function edge_ids)
{
this.edge_ids = edge_ids;
}
/**
* Provides a map from data type name to graph data.
*
* @param graph_map map from data type name to graph data
*/
public void setGraphData(Map>> graph_map)
{
graph_data = graph_map;
}
/**
* Provides a map from data type name to vertex data.
*
* @param vertex_map map from data type name to vertex data
*/
public void setVertexData(Map> vertex_map)
{
vertex_data = vertex_map;
}
/**
* Provides a map from data type name to edge data.
*
* @param edge_map map from data type name to edge data
*/
public void setEdgeData(Map> edge_map)
{
edge_data = edge_map;
}
/**
* Adds a new graph data specification.
*
* @param id the ID of the data to add
* @param description a description of the data to add
* @param default_value a default value for the data type
* @param graph_transformer a mapping from graphs to their string representations
*/
public void addGraphData(String id, String description, String default_value,
Function, String> graph_transformer)
{
if (graph_data.equals(Collections.EMPTY_MAP))
graph_data = new HashMap>>();
graph_data.put(id, new GraphMLMetadata>(description,
default_value, graph_transformer));
}
/**
* Adds a new vertex data specification.
*
* @param id the ID of the data to add
* @param description a description of the data to add
* @param default_value a default value for the data type
* @param vertex_transformer a mapping from vertices to their string representations
*/
public void addVertexData(String id, String description, String default_value,
Function vertex_transformer)
{
if (vertex_data.equals(Collections.EMPTY_MAP))
vertex_data = new HashMap>();
vertex_data.put(id, new GraphMLMetadata(description, default_value,
vertex_transformer));
}
/**
* Adds a new edge data specification.
*
* @param id the ID of the data to add
* @param description a description of the data to add
* @param default_value a default value for the data type
* @param edge_transformer a mapping from edges to their string representations
*/
public void addEdgeData(String id, String description, String default_value,
Function edge_transformer)
{
if (edge_data.equals(Collections.EMPTY_MAP))
edge_data = new HashMap>();
edge_data.put(id, new GraphMLMetadata(description, default_value,
edge_transformer));
}
/**
* Provides vertex descriptions.
* @param vertex_desc a mapping from vertices to their descriptions
*/
public void setVertexDescriptions(Function vertex_desc)
{
this.vertex_desc = vertex_desc;
}
/**
* Provides edge descriptions.
* @param edge_desc a mapping from edges to their descriptions
*/
public void setEdgeDescriptions(Function edge_desc)
{
this.edge_desc = edge_desc;
}
/**
* Provides graph descriptions.
* @param graph_desc a mapping from graphs to their descriptions
*/
public void setGraphDescriptions(Function, String> graph_desc)
{
this.graph_desc = graph_desc;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy