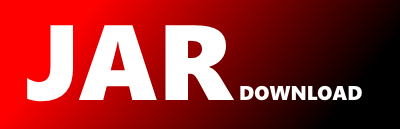
com.northernwall.hadrian.db.DataAccess Maven / Gradle / Ivy
/*
* Copyright 2014 Richard Thurston.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.northernwall.hadrian.db;
import com.northernwall.hadrian.domain.Audit;
import com.northernwall.hadrian.domain.CustomFunction;
import com.northernwall.hadrian.domain.DataStore;
import com.northernwall.hadrian.domain.Vip;
import com.northernwall.hadrian.domain.Host;
import com.northernwall.hadrian.domain.Module;
import com.northernwall.hadrian.domain.ModuleFile;
import com.northernwall.hadrian.domain.Service;
import com.northernwall.hadrian.domain.ModuleRef;
import com.northernwall.hadrian.domain.Team;
import com.northernwall.hadrian.domain.WorkItem;
import com.northernwall.hadrian.handlers.utility.HealthWriter;
import java.io.IOException;
import java.util.List;
/**
*
* @author Richard Thurston
*/
public interface DataAccess {
String getVersion();
void setVersion(String version);
void getHealth(HealthWriter writer) throws IOException;
List getTeams();
Team getTeam(String teamId);
void saveTeam(Team team);
void updateTeam(Team team);
List getAllServices();
List getActiveServices();
Service getService(String serviceId);
void saveService(Service service);
void updateService(Service service);
List getHosts(String serviceId);
Host getHost(String serviceId, String hostId);
void saveHost(Host host);
void updateHost(Host host);
void deleteHost(Host host);
List getVips(String serviceId);
Vip getVip(String serviceId, String vipId);
void saveVip(Vip vip);
void updateVip(Vip vip);
void deleteVip(String serviceId, String vipId);
List getModuleRefs();
List getModuleRefsByClient(String clientServiceId, String clientModuleId);
List getModuleRefsByServer(String serverServiceId, String serverModuleId);
void saveModuleRef(ModuleRef moduleRef);
void deleteModuleRef(String clientServiceId, String clientModuleId, String serverServiceId, String serverModuleId);
List getCustomFunctions(String serviceId);
CustomFunction getCustomFunction(String serviceId, String customFunctionId);
void saveCustomFunction(CustomFunction customFunction);
void updateCustomFunction(CustomFunction customFunction);
void deleteCustomFunction(String serviceId, String customFunctionId);
List getModules(String serviceId);
Module getModule(String serviceId, String moduleId);
void saveModule(Module module);
void updateModule(Module module);
void deleteModule(String serviceId, String moduleId);
List getModuleFiles(String serviceId, String moduleId, String environment);
ModuleFile getModuleFile(String serviceId, String moduleId, String environment, String name);
void saveModuleFile(ModuleFile moduleFile);
void updateModuleFile(ModuleFile moduleFile);
void deleteModuleFile(String serviceId, String moduleId, String environment, String name);
List getDataStores(String serviceId);
DataStore getDataStore(String serviceId, String dataStoreId);
void saveDataStore(DataStore dataStore);
void updateDataStore(DataStore dataStore);
void deleteDataStore(String serviceId, String dataStoreId);
List getWorkItems();
WorkItem getWorkItem(String id);
void saveWorkItem(WorkItem workItem);
void deleteWorkItem(String id);
int getWorkItemStatus(String id);
void saveWorkItemStatus(String id, int i);
SearchResult doSearch(String searchSpace, String searchText);
void insertSearch(String searchSpace, String searchText, String serviceId, String moduleId, String hostId);
void deleteSearch(String searchSpace, String searchText);
void updateStatus(String id, boolean busy, String status, String statusCode);
void saveAudit(Audit audit, String output);
List getAudit(String serviceId, int year, int month, int startDay, int endDay);
String getAuditOutput(String serviceId, String auditId);
boolean getAvailability();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy