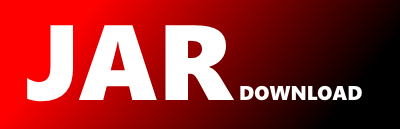
com.nostra13.universalimageloader.core.DisplayImageOptions Maven / Gradle / Ivy
package com.nostra13.universalimageloader.core;
import android.graphics.Bitmap;
import com.nostra13.universalimageloader.core.assist.ImageScaleType;
import com.nostra13.universalimageloader.core.display.BitmapDisplayer;
import com.nostra13.universalimageloader.core.display.SimpleBitmapDisplayer;
/**
* Contains options for image display. Defines:
*
* - whether stub image will be displayed in {@link android.widget.ImageView ImageView} during image loading
* - whether stub image will be displayed in {@link android.widget.ImageView ImageView} if empty URI is passed
* - whether {@link android.widget.ImageView ImageView} should be reset before image loading start
* - whether loaded image will be cached in memory
* - whether loaded image will be cached on disc
* - image scale type
* - bitmap decoding configuration
* - delay before loading of image
* - how decoded {@link Bitmap} will be displayed
*
*
* You can create instance:
*
* - with {@link Builder}:
* i.e. :
* new {@link DisplayImageOptions}.{@link Builder#Builder() Builder()}.{@link Builder#cacheInMemory() cacheInMemory()}.
* {@link Builder#showStubImage(int) showStubImage()}.{@link Builder#build() build()}
*
* - or by static method: {@link #createSimple()}
*
* @author Sergey Tarasevich (nostra13[at]gmail[dot]com)
*/
public final class DisplayImageOptions {
private final int stubImage;
private final int imageForEmptyUri;
private final boolean resetViewBeforeLoading;
private final boolean cacheInMemory;
private final boolean cacheOnDisc;
private final ImageScaleType imageScaleType;
private final Bitmap.Config bitmapConfig;
private final int delayBeforeLoading;
private final BitmapDisplayer displayer;
private DisplayImageOptions(Builder builder) {
stubImage = builder.stubImage;
imageForEmptyUri = builder.imageForEmptyUri;
resetViewBeforeLoading = builder.resetViewBeforeLoading;
cacheInMemory = builder.cacheInMemory;
cacheOnDisc = builder.cacheOnDisc;
imageScaleType = builder.imageScaleType;
bitmapConfig = builder.bitmapConfig;
delayBeforeLoading = builder.delayBeforeLoading;
displayer = builder.displayer;
}
boolean isShowStubImage() {
return stubImage != 0;
}
boolean isShowImageForEmptyUri() {
return imageForEmptyUri != 0;
}
Integer getStubImage() {
return stubImage;
}
Integer getImageForEmptyUri() {
return imageForEmptyUri;
}
boolean isResetViewBeforeLoading() {
return resetViewBeforeLoading;
}
boolean isCacheInMemory() {
return cacheInMemory;
}
boolean isCacheOnDisc() {
return cacheOnDisc;
}
ImageScaleType getImageScaleType() {
return imageScaleType;
}
Bitmap.Config getBitmapConfig() {
return bitmapConfig;
}
boolean isDelayBeforeLoading() {
return delayBeforeLoading > 0;
}
int getDelayBeforeLoading() {
return delayBeforeLoading;
}
BitmapDisplayer getDisplayer() {
return displayer;
}
/**
* Builder for {@link DisplayImageOptions}
*
* @author Sergey Tarasevich (nostra13[at]gmail[dot]com)
*/
public static class Builder {
private int stubImage = 0;
private int imageForEmptyUri = 0;
private boolean resetViewBeforeLoading = false;
private boolean cacheInMemory = false;
private boolean cacheOnDisc = false;
private ImageScaleType imageScaleType = ImageScaleType.IN_SAMPLE_POWER_OF_2;
private Bitmap.Config bitmapConfig = Bitmap.Config.ARGB_8888;
private int delayBeforeLoading = 0;
private BitmapDisplayer displayer = DefaultConfigurationFactory.createBitmapDisplayer();
/**
* Stub image will be displayed in {@link android.widget.ImageView ImageView} during image loading
*
* @param stubImageRes Stub image resource
*/
public Builder showStubImage(int stubImageRes) {
stubImage = stubImageRes;
return this;
}
/**
* Image will be displayed in {@link android.widget.ImageView ImageView} if empty URI (null or empty string)
* will be passed to ImageLoader.displayImage(...) method.
*
* @param imageRes Image resource
*/
public Builder showImageForEmptyUri(int imageRes) {
imageForEmptyUri = imageRes;
return this;
}
/** {@link android.widget.ImageView ImageView} will be reset (set null) before image loading start */
public Builder resetViewBeforeLoading() {
resetViewBeforeLoading = true;
return this;
}
/** Loaded image will be cached in memory */
public Builder cacheInMemory() {
cacheInMemory = true;
return this;
}
/** Loaded image will be cached on disc */
public Builder cacheOnDisc() {
cacheOnDisc = true;
return this;
}
/**
* Sets {@link ImageScaleType decoding type} for image loading task. Default value -
* {@link ImageScaleType#POWER_OF_2}
*/
public Builder imageScaleType(ImageScaleType imageScaleType) {
this.imageScaleType = imageScaleType;
return this;
}
/** Sets {@link Bitmap.Config bitmap config} for image decoding. Default value - {@link Bitmap.Config#ARGB_8888} */
public Builder bitmapConfig(Bitmap.Config bitmapConfig) {
this.bitmapConfig = bitmapConfig;
return this;
}
/** Sets delay time before starting loading task. Default - no delay. */
public Builder delayBeforeLoading(int delayInMillis) {
this.delayBeforeLoading = delayInMillis;
return this;
}
/**
* Sets custom {@link BitmapDisplayer displayer} for image loading task. Default value -
* {@link DefaultConfigurationFactory#createBitmapDisplayer()}
*/
public Builder displayer(BitmapDisplayer displayer) {
this.displayer = displayer;
return this;
}
/** Sets all options equal to incoming options */
public Builder cloneFrom(DisplayImageOptions options) {
stubImage = options.stubImage;
imageForEmptyUri = options.imageForEmptyUri;
resetViewBeforeLoading = options.resetViewBeforeLoading;
cacheInMemory = options.cacheInMemory;
cacheOnDisc = options.cacheOnDisc;
imageScaleType = options.imageScaleType;
bitmapConfig = options.bitmapConfig;
delayBeforeLoading = options.delayBeforeLoading;
displayer = options.displayer;
return this;
}
/** Builds configured {@link DisplayImageOptions} object */
public DisplayImageOptions build() {
return new DisplayImageOptions(this);
}
}
/**
* Creates options appropriate for single displaying:
*
* - View will not be reset before loading
* - Loaded image will not be cached in memory
* - Loaded image will not be cached on disc
* - {@link ImageScaleType#IN_SAMPLE_POWER_OF_2} decoding type will be used
* - {@link Bitmap.Config#ARGB_8888} bitmap config will be used for image decoding
* - {@link SimpleBitmapDisplayer} will be used for image displaying
*
*
* These option are appropriate for simple single-use image (from drawables or from Internet) displaying.
*/
public static DisplayImageOptions createSimple() {
return new Builder().build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy