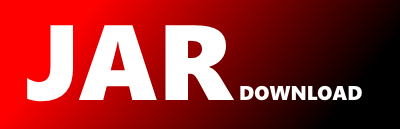
com.notronix.hibermate.impl.DefaultPersistenceManagerImpl Maven / Gradle / Ivy
package com.notronix.hibermate.impl;
import com.notronix.hibermate.api.*;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.boot.model.relational.Database;
import org.hibernate.jpa.TypedParameterValue;
import org.hibernate.query.NativeQuery;
import org.hibernate.resource.transaction.spi.TransactionStatus;
import org.hibernate.type.BasicTypeRegistry;
import org.hibernate.type.Type;
import java.io.Serializable;
import java.time.Instant;
import java.util.List;
import java.util.Map;
import static com.notronix.albacore.ContainerUtils.*;
import static com.notronix.albacore.NumberUtils.doubleValueOf;
import static com.notronix.albacore.NumberUtils.longValueOf;
import static java.util.Objects.requireNonNull;
import static org.apache.commons.lang3.StringUtils.isNotBlank;
public class DefaultPersistenceManagerImpl implements PersistenceManager
{
private final BasicTypeRegistry typeRegistry;
private final DatabaseIntegrator databaseIntegrator;
private final SessionFactory sessionFactory;
protected DefaultPersistenceManagerImpl(SessionFactory sessionFactory,
DatabaseIntegrator databaseIntegrator,
BasicTypeRegistry typeRegistry) {
this.sessionFactory = requireNonNull(sessionFactory);
this.databaseIntegrator = requireNonNull(databaseIntegrator);
this.typeRegistry = requireNonNull(typeRegistry);
}
@Override
public Session openSession() throws PersistenceException {
try {
return sessionFactory.openSession();
}
catch (Exception ex) {
throw new PersistenceException("An error occurred trying to open session.");
}
}
@Override
public Session getCurrentSession() throws PersistenceException {
try {
return sessionFactory.getCurrentSession();
}
catch (Exception ex) {
throw new PersistenceException("An error occurred trying to open session.");
}
}
@Override
public Database getDatabase() {
return databaseIntegrator.getDatabase();
}
@Override
public K makePersistent(PersistenceCapable object) throws PersistenceException {
Session session = openSession();
Transaction transaction = null;
K systemId;
try {
transaction = session.beginTransaction();
//noinspection unchecked
systemId = (K) session.save(object);
transaction.commit();
}
catch (Exception e) {
if (transaction != null && transaction.getStatus() == TransactionStatus.ACTIVE) {
transaction.rollback();
}
throw new PersistenceException("An error occurred trying to persist object.", e);
}
finally {
session.close();
}
return systemId;
}
@Override
public void deletePersistent(PersistenceCapable> object) throws PersistenceException {
Transaction transaction = null;
try (Session session = openSession()) {
@SuppressWarnings("unchecked")
Class> clazz = (Class>) object.getClass();
transaction = session.beginTransaction();
PersistenceCapable> alias = session.get(clazz, object.getSystemId());
session.delete(alias);
transaction.commit();
}
catch (Exception e) {
if (transaction != null && transaction.getStatus() == TransactionStatus.ACTIVE) {
transaction.rollback();
}
throw new PersistenceException("An error occurred trying to delete object.", e);
}
}
@Override
public void update(String recordId, String objectType, PersistenceCapable> object)
throws PersistenceException {
try {
if (object instanceof Syncable) {
((Syncable) object).setLastSynchronizedDate(Instant.now());
}
if (PersistenceManager.itIsAValid(object)) {
update(object);
}
else {
makePersistent(object);
}
}
catch (Exception ex) {
throw new PersistenceException(recordId + ": Failed storing " + objectType + ".", ex);
}
}
@Override
public > T update(T object) throws PersistenceException {
Session session = openSession();
Transaction transaction = null;
T alias;
try {
transaction = session.beginTransaction();
//noinspection unchecked
alias = (T) session.merge(object);
transaction.commit();
}
catch (Exception e) {
if (transaction != null && transaction.getStatus() == TransactionStatus.ACTIVE) {
transaction.rollback();
}
throw new PersistenceException("An error occurred trying to update object.", e);
}
finally {
session.close();
}
return alias;
}
@Override
public > T get(Class objectClass, K systemId)
throws PersistenceException {
if (systemId == null) {
throw new PersistenceException("systemId is null", null);
}
Session session = openSession();
Transaction transaction = null;
T alias;
try {
transaction = session.beginTransaction();
alias = session.get(objectClass, systemId);
transaction.commit();
}
catch (Exception e) {
if (transaction != null && transaction.getStatus() == TransactionStatus.ACTIVE) {
transaction.rollback();
}
throw new PersistenceException("An error occurred trying to get object with system id.", e);
}
finally {
session.close();
}
return alias;
}
@Override
public > T getFirst(Class objectClass) throws PersistenceException {
return getFirst(objectClass, null, null);
}
@Override
public > T getFirst(Class objectClass, String predicate)
throws PersistenceException {
return getFirst(objectClass, predicate, null);
}
@Override
public > T getFirst(Class objectClass, String
predicate, Map params)
throws PersistenceException {
return theFirst(getList(objectClass, predicate, params));
}
@Override
public > List getList(Class objectClass, String join, String
predicate, Map params)
throws PersistenceException {
String query = "from " + objectClass.getSimpleName();
if (isNotBlank(join)) {
query += " " + join;
}
if (isNotBlank(predicate)) {
query += " where " + predicate;
}
return getList(query, params, objectClass);
}
@Override
public > List getList(Class objectClass, String join, String predicate)
throws PersistenceException {
return getList(objectClass, join, predicate, null);
}
@Override
public > List getList(Class objectClass, String
predicate, Map params)
throws PersistenceException {
return getList(objectClass, null, predicate, params);
}
@Override
public > List getList(Class objectClass, String predicate)
throws PersistenceException {
return getList(objectClass, null, predicate, null);
}
@Override
public Long getLong(DBQuery query) throws PersistenceException {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy