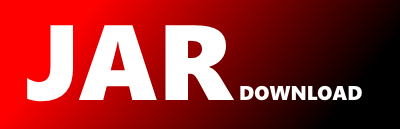
com.novell.ldap.events.edir.eventdata.BinderyObjectEventData Maven / Gradle / Ivy
/* **************************************************************************
* $OpenLDAP: pkg/jldap/com/novell/ldap/events/edir/eventdata/BinderyObjectEventData.java,v 1.3 2005/01/17 15:48:57 sunilk Exp $
*
* Copyright (C) 1999-2002 Novell, Inc. All Rights Reserved.
*
* THIS WORK IS SUBJECT TO U.S. AND INTERNATIONAL COPYRIGHT LAWS AND
* TREATIES. USE, MODIFICATION, AND REDISTRIBUTION OF THIS WORK IS SUBJECT
* TO VERSION 2.0.1 OF THE OPENLDAP PUBLIC LICENSE, A COPY OF WHICH IS
* AVAILABLE AT HTTP://WWW.OPENLDAP.ORG/LICENSE.HTML OR IN THE FILE "LICENSE"
* IN THE TOP-LEVEL DIRECTORY OF THE DISTRIBUTION. ANY USE OR EXPLOITATION
* OF THIS WORK OTHER THAN AS AUTHORIZED IN VERSION 2.0.1 OF THE OPENLDAP
* PUBLIC LICENSE, OR OTHER PRIOR WRITTEN CONSENT FROM NOVELL, COULD SUBJECT
* THE PERPETRATOR TO CRIMINAL AND CIVIL LIABILITY.
******************************************************************************/
package com.novell.ldap.events.edir.eventdata;
import com.novell.ldap.asn1.ASN1Integer;
import com.novell.ldap.asn1.ASN1Object;
import com.novell.ldap.asn1.ASN1OctetString;
import com.novell.ldap.asn1.LBERDecoder;
import com.novell.ldap.events.edir.EventResponseData;
import java.io.ByteArrayInputStream;
import java.io.IOException;
/**
* This class represents the data for Bindery Events. The event data has
* the following encoding:-
*
*
* BinderyObjectInfo [APPLICATION 5]::=
* SEQUENCE {
* entry LDAPDN,
* type INTEGER,
* emuObjFlags INTEGER,
* security INTEGER,
* name LDAPString (48)
* }
*
*
*
* Note: Please refer the JLDAP SDK Documentation at
*
* http://developer.novell.com/ndk/jldap.htm for details of all
* the properties.
*
*/
public class BinderyObjectEventData implements EventResponseData {
private final String entryDN;
private final int type;
private final int emuObjFlags;
private final int security;
private final String name;
/**
* Default Constructor
*
* @param message ASN1Object containing the encoded data as String.
*
* @throws IOException When decoding of message fails.
*/
public BinderyObjectEventData(final ASN1Object message)
throws IOException {
super();
byte[] data = ((ASN1OctetString) message).byteValue();
ByteArrayInputStream in = new ByteArrayInputStream(data);
LBERDecoder decode = new LBERDecoder();
int[] length = new int[1];
entryDN =
((ASN1OctetString) decode.decode(in, length)).stringValue();
type = ((ASN1Integer) decode.decode(in, length)).intValue();
emuObjFlags = ((ASN1Integer) decode.decode(in, length)).intValue();
security = ((ASN1Integer) decode.decode(in, length)).intValue();
name = ((ASN1OctetString) decode.decode(in, length)).stringValue();
}
/**
* Get the EmuObj Flag Property.
*
* @return Emu Flags as integer.
*/
public int getEmuObjFlags() {
return emuObjFlags;
}
/**
* Gets the EntryDn as this event.
*
* @return Entry DN as String.
*/
public String getEntryDN() {
return entryDN;
}
/**
* Returns the Name.
*
* @return Name as String.
*/
public String getName() {
return name;
}
/**
* Returns the security Flags.
*
* @return Security as integer.
*/
public int getSecurity() {
return security;
}
/**
* Returns the Event Type.
*
* @return event Type as integer.
*/
public int getType() {
return type;
}
/**
* Returns a string representation of the object.
*
* @return a string representation of the object.
*
* @see java.lang.Object#toString()
*/
public String toString() {
StringBuffer buf = new StringBuffer();
buf.append("[BinderyObjectEvent[EntryDn=" + getEntryDN() + "]");
buf.append("[Type=" + getType() + "]");
buf.append("[EnumOldFlags=" + getEmuObjFlags() + "]");
buf.append("[Secuirty=" + getSecurity() + "]");
buf.append("[Name=" + getName() + "]]");
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy