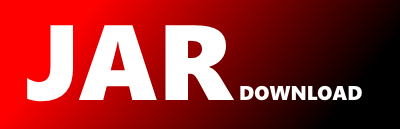
org.ietf.ldap.LDAPResponseQueue Maven / Gradle / Ivy
/* **************************************************************************
* $OpenLDAP: pkg/jldap/org/ietf/ldap/LDAPResponseQueue.java,v 1.2 2004/01/16 04:55:00 sunilk Exp $
*
* Copyright (C) 1999, 2000, 2001 Novell, Inc. All Rights Reserved.
*
* THIS WORK IS SUBJECT TO U.S. AND INTERNATIONAL COPYRIGHT LAWS AND
* TREATIES. USE, MODIFICATION, AND REDISTRIBUTION OF THIS WORK IS SUBJECT
* TO VERSION 2.0.1 OF THE OPENLDAP PUBLIC LICENSE, A COPY OF WHICH IS
* AVAILABLE AT HTTP://WWW.OPENLDAP.ORG/LICENSE.HTML OR IN THE FILE "LICENSE"
* IN THE TOP-LEVEL DIRECTORY OF THE DISTRIBUTION. ANY USE OR EXPLOITATION
* OF THIS WORK OTHER THAN AS AUTHORIZED IN VERSION 2.0.1 OF THE OPENLDAP
* PUBLIC LICENSE, OR OTHER PRIOR WRITTEN CONSENT FROM NOVELL, COULD SUBJECT
* THE PERPETRATOR TO CRIMINAL AND CIVIL LIABILITY.
******************************************************************************/
package org.ietf.ldap;
/**
* Encapsulates a low-level mechanism for processing asynchronous messages
* received from a server.
*
* @see
com.novell.ldap.LDAPResponseQueue
*/
public class LDAPResponseQueue implements LDAPMessageQueue
{
private com.novell.ldap.LDAPResponseQueue queue;
/**
* Constructs a response queue from com.novell.ldap.LDAPResponseQueue
*/
/* package */
LDAPResponseQueue(com.novell.ldap.LDAPResponseQueue queue)
{
this.queue = queue;
return;
}
/**
* Returns the com.novell.ldap.LDAPResponseQueue object
*/
/* package */
com.novell.ldap.LDAPResponseQueue getWrappedObject()
{
return queue;
}
/**
* Returns the message IDs for all outstanding requests.
*
* @see
com.novell.ldap.LDAPResponse.getMessageIDs()
*/
public int[] getMessageIDs()
{
return queue.getMessageIDs();
}
/**
* Reports whether a response has been received from the server.
*
* @see
com.novell.ldap.LDAPResponse.isResponseReceived()
*/
public boolean isResponseReceived()
{
return queue.isResponseReceived();
}
/**
* Reports whether a response has been received from the server for a
* particular message id.
*
* @see
com.novell.ldap.LDAPResponse.isResponseReceived(int)
*/
public boolean isResponseReceived(int msgid)
{
return queue.isResponseReceived( msgid);
}
/**
* Merges two response queues by moving the contents from another
* queue to this one.
*
* @see
com.novell.ldap.LDAPResponse.merge(LDAPMessageQueue)
*/
public void merge(LDAPMessageQueue queue2)
{
if( queue2 == null) {
queue.merge( (com.novell.ldap.LDAPMessageQueue)null);
}
if( queue2 instanceof LDAPResponseQueue) {
queue.merge( ((LDAPResponseQueue)queue2).getWrappedObject() );
} else {
queue.merge( ((LDAPSearchQueue)queue2).getWrappedObject() );
}
return;
}
/**
* Returns the response.
*
* @see
com.novell.ldap.LDAPResponse.getResponse()
*/
public LDAPMessage getResponse()
throws LDAPException
{
try {
return new LDAPMessage(queue.getResponse());
} catch( com.novell.ldap.LDAPException ex) {
if( ex instanceof com.novell.ldap.LDAPReferralException) {
throw new LDAPReferralException(
(com.novell.ldap.LDAPReferralException)ex);
} else {
throw new LDAPException( ex);
}
}
}
/**
* Returns the response for a particular message id.
*
* @see
com.novell.ldap.LDAPResponse.getResponse(int)
*/
public LDAPMessage getResponse(int msgid)
throws LDAPException
{
try {
return new LDAPMessage(queue.getResponse(msgid));
} catch( com.novell.ldap.LDAPException ex) {
if( ex instanceof com.novell.ldap.LDAPReferralException) {
throw new LDAPReferralException(
(com.novell.ldap.LDAPReferralException)ex);
} else {
throw new LDAPException( ex);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy