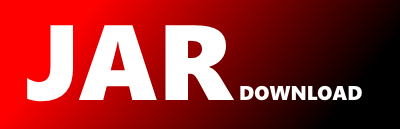
com.nu.art.http.HttpResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-module Show documentation
Show all versions of http-module Show documentation
A collection of core tools I use
package com.nu.art.http;
import com.nu.art.http.headers.ContentType;
import com.nu.art.http.headers.EncodingType;
import com.nu.art.http.interfaces.HeaderType;
import com.nu.art.core.interfaces.ILogger;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.zip.GZIPInputStream;
/**
* Created by TacB0sS on 08-Mar 2017.
*/
public class HttpResponse {
public int responseCode;
public Throwable exception;
Map> headers;
InputStream inputStream;
String responseAsString;
private int responseSize;
public Map> getHeaders() {
if (headers == null)
headers = new HashMap<>();
return headers;
}
public List getHeader(String key) {
List headers = getHeaders().get(key);
if (headers == null)
this.headers.put(key, headers = new ArrayList<>());
return headers;
}
private boolean hasFailed() {
return responseCode >= 300;
}
final boolean processFailure(HttpURLConnection connection)
throws IOException {
if (!hasFailed())
return false;
InputStream responseStream = connection.getErrorStream();
if (responseStream == null) {
throw new HttpException(this, "Got an error response without Error Body");
}
inputStream = connection.getErrorStream();
if (hasEncodingType(EncodingType.GZip))
inputStream = new GZIPInputStream(inputStream);
return true;
}
@SuppressWarnings("unchecked")
final void processSuccess(HttpURLConnection connection)
throws IOException {
inputStream = connection.getInputStream();
if (inputStream == null)
return;
if (hasEncodingType(EncodingType.GZip))
inputStream = new GZIPInputStream(inputStream);
List header = getHeader("content-length");
if (header != null && header.size() == 1)
responseSize = Integer.valueOf(header.get(0));
else if (inputStream != null)
responseSize = inputStream.available();
}
final void printResponse(ILogger logger) {
logger.logInfo("+-- Response Code: " + responseCode);
if (hasFailed()) {
if (responseAsString != null)
logger.logError("+-- Response: " + responseAsString);
else if (responseSize > 0)
logger.logError("+-- Error Response Length: " + responseSize);
} else {
if (responseAsString != null)
logger.logVerbose("+-- Response: " + responseAsString);
else if (responseSize > 0)
logger.logInfo("+-- Response Length: " + responseSize);
}
logger.logVerbose("+-- Response Headers: ");
Map> headers = getHeaders();
for (String key : headers.keySet()) {
for (String value : headers.get(key)) {
logger.logVerbose("+---- " + key + ": " + value);
}
}
}
/*
* Utility functions
*/
private boolean hasEncodingType(EncodingType encodingType) {
return hasMatchingHeader(encodingType);
}
protected final boolean isContentType(ContentType header) {
return hasMatchingHeader(header);
}
protected final boolean hasMatchingHeader(HeaderType header) {
List contentTypes = headers.get(header.getHeader().key);
if (contentTypes == null || contentTypes.size() == 0)
return false;
for (String contentType : contentTypes) {
if (!contentType.contains(header.getHeader().value))
continue;
return true;
}
return false;
}
final void close() {
try {
if (inputStream != null)
inputStream.close();
} catch (IOException ignore) {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy