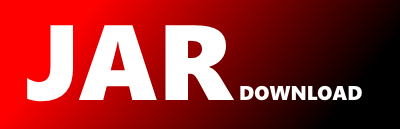
com.nutanix.dp1.aio.common.v1.config.KVPair Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aiops-java-client Show documentation
Show all versions of aiops-java-client Show documentation
Manage infrastructure on-premises and in the cloud seamlessly through AIOps features such as Analysis, Reporting, Capacity Planning, What if Analysis, VM Rightsizing, Troubleshooting, App Discovery, Broad Observability, and Ops Automation through Playbooks.
The newest version!
/*
* Generated file ..
*
* Product version: 4.0.3-alpha-2
*
* Part of the Nutanix Aiops Versioned APIs
*
* (c) 2023 Nutanix Inc. All rights reserved
*
*/
package com.nutanix.dp1.aio.common.v1.config;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.Setter;
import lombok.AccessLevel;
import com.nutanix.devplatform.models.PrettyModeViews.*;
import com.fasterxml.jackson.annotation.JsonView;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.nutanix.dp1.aio.deserializers.AioObjectTypeTypedObject;
import javax.validation.constraints.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static com.nutanix.dp1.aio.deserializers.AioDeserializerUtils.*;
/**
* A map describing a set of keys and their corresponding values.
*
*/
@Data
@lombok.extern.slf4j.Slf4j
public class KVPair implements java.io.Serializable, AioObjectTypeTypedObject {
public KVPair() {
this.$objectType = this.initialize$objectType();
this.$reserved = new java.util.LinkedHashMap<>();
this.$reserved.put("$fqObjectType", this.initialize$fqObjectType());
this.$unknownFields = new java.util.LinkedHashMap<>();
}
@lombok.Builder(builderMethodName = "KVPairBuilder")
public KVPair(String name, Object value) {
this.$objectType = this.initialize$objectType();
this.$reserved = new java.util.LinkedHashMap<>();
this.$reserved.put("$fqObjectType", this.initialize$fqObjectType());
this.$unknownFields = new java.util.LinkedHashMap<>();
this.setName(name);
this.setValueInWrapper(value);
}
protected String initialize$objectType() {
return "common.v1.config.KVPair";
}
protected String initialize$fqObjectType() {
return "common.v1.r0.a3.config.KVPair";
}
@JsonAnySetter
private void setUndeserializedFields(String name, String value) {
$unknownFields.put(name, value);
}
/**
* The key of this key-value pair
*/
@JsonProperty("name")
public String name = null;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
@Getter
private String $valueItemDiscriminator = null;
@Data
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(using = OneOfValueWrapper.OneOfValueWrapperJsonDeserializer.class)
public static class OneOfValueWrapper {
private static final java.util.List EMPTY_LIST = new java.util.ArrayList<>();
private static final java.util.Map EMPTY_MAP = java.util.Collections.emptyMap();
public OneOfValueWrapper() {
}
public OneOfValueWrapper(java.util.List data) {
if(data.isEmpty()) {
this.discriminator = "EMPTY_LIST";
this.$objectType = "EMPTY_LIST";
return;
}
Class> cls = data.get(0).getClass();
if(String.class.equals(cls)) {
this.oneOfType1005 = (java.util.List) data;
this.discriminator = "List";
this.$objectType = "List";
return;
}
throw new IllegalArgumentException("Attempting to set unsupported object type in OneOfValueWrapper:" + data.getClass().getName());
}
public OneOfValueWrapper(java.util.Map data) {
if(data.isEmpty()) {
this.discriminator = "EMPTY_MAP";
this.$objectType = "EMPTY_MAP";
return;
}
Class> cls = data.values().toArray()[0].getClass();
if(String.class.equals(cls)) {
this.oneOfType1006 = (java.util.Map) data;
this.discriminator = "Map";
this.$objectType = "Map";
return;
}
throw new IllegalArgumentException("Attempting to set unsupported object type in OneOfValueWrapper:" + data.getClass().getName());
}
public OneOfValueWrapper(String data) {
this.oneOfType1002 = data;
this.discriminator = "String";
this.$objectType = "String";
}
public OneOfValueWrapper(Integer data) {
this.oneOfType1003 = data;
this.discriminator = "Integer";
this.$objectType = "Integer";
}
public OneOfValueWrapper(Boolean data) {
this.oneOfType1004 = data;
this.discriminator = "Boolean";
this.$objectType = "Boolean";
}
@com.nutanix.dp1.aio.annotations.AioJsonDeserializer
@org.springframework.stereotype.Component("com.nutanix.dp1.aio.common.v1.config.KVPairJsonDeserializer")
private static class OneOfValueWrapperJsonDeserializer extends com.nutanix.dp1.aio.deserializers.AioOneOfDeserializer {
private static final com.fasterxml.jackson.databind.type.TypeFactory TYPE_FACTORY = com.fasterxml.jackson.databind.type.TypeFactory.defaultInstance();
private static final com.fasterxml.jackson.databind.JavaType ONE_OF_TYPE1002 = TYPE_FACTORY.constructType(String.class);
private static final com.fasterxml.jackson.databind.JavaType ONE_OF_TYPE1003 = TYPE_FACTORY.constructType(Integer.class);
private static final com.fasterxml.jackson.databind.JavaType ONE_OF_TYPE1004 = TYPE_FACTORY.constructType(Boolean.class);
private static final com.fasterxml.jackson.databind.JavaType ONE_OF_TYPE1005 = TYPE_FACTORY.constructCollectionType(java.util.List.class, String.class);
private static final com.fasterxml.jackson.databind.JavaType ONE_OF_TYPE1006 = TYPE_FACTORY.constructMapType(java.util.HashMap.class, String.class, String.class);
public OneOfValueWrapperJsonDeserializer() {
super(TYPE_FACTORY.constructType(OneOfValueWrapper.class));
}
@Override
protected void setDataObject(OneOfValueWrapper oneOfObject, Object nestedObject) {
if (oneOfObject == null) {
throw new IllegalArgumentException("Instance of OneOfValueWrapper provided is null");
}
if(ONE_OF_TYPE1002.getRawClass().isAssignableFrom(nestedObject.getClass())) {
oneOfObject.setValue(nestedObject);
}
else if(ONE_OF_TYPE1003.getRawClass().isAssignableFrom(nestedObject.getClass())) {
oneOfObject.setValue(nestedObject);
}
else if(ONE_OF_TYPE1004.getRawClass().isAssignableFrom(nestedObject.getClass())) {
oneOfObject.setValue(nestedObject);
}
else if(ONE_OF_TYPE1005.getRawClass().isAssignableFrom(nestedObject.getClass())) {
oneOfObject.setValue(nestedObject);
}
else if(ONE_OF_TYPE1006.getRawClass().isAssignableFrom(nestedObject.getClass())) {
oneOfObject.setValue(nestedObject);
}
else {
throw new IllegalArgumentException("Attempting to set unsupported object type in OneOfValueWrapper:" + nestedObject.getClass().getName());
}
}
public String getPackagePrefix() {
return "com.nutanix.dp1.aio";
}
}
@com.fasterxml.jackson.annotation.JsonIgnore
@lombok.Getter(lombok.AccessLevel.NONE)
@lombok.Setter(lombok.AccessLevel.NONE)
private String oneOfType1002;
@com.fasterxml.jackson.annotation.JsonIgnore
@lombok.Getter(lombok.AccessLevel.NONE)
@lombok.Setter(lombok.AccessLevel.NONE)
private Integer oneOfType1003;
@com.fasterxml.jackson.annotation.JsonIgnore
@lombok.Getter(lombok.AccessLevel.NONE)
@lombok.Setter(lombok.AccessLevel.NONE)
private Boolean oneOfType1004;
@com.fasterxml.jackson.annotation.JsonIgnore
@lombok.Getter(lombok.AccessLevel.NONE)
@lombok.Setter(lombok.AccessLevel.NONE)
private java.util.List oneOfType1005;
@com.fasterxml.jackson.annotation.JsonIgnore
@lombok.Getter(lombok.AccessLevel.NONE)
@lombok.Setter(lombok.AccessLevel.NONE)
private Map oneOfType1006;
@lombok.Setter(lombok.AccessLevel.NONE)
private String discriminator;
@com.fasterxml.jackson.annotation.JsonIgnore
@lombok.Setter(lombok.AccessLevel.NONE)
private String $objectType;
@com.fasterxml.jackson.annotation.JsonGetter
public Object getValue() {
if("EMPTY_LIST".equals(this.discriminator)) {
return EMPTY_LIST;
}
if("EMPTY_MAP".equals(this.discriminator)) {
return EMPTY_MAP;
}
if("String".equals(this.discriminator)) {
return this.oneOfType1002;
}
if("Integer".equals(this.discriminator)) {
return this.oneOfType1003;
}
if("Boolean".equals(this.discriminator)) {
return this.oneOfType1004;
}
if("List".equals(this.discriminator)) {
return this.oneOfType1005;
}
if("Map".equals(this.discriminator)) {
return this.oneOfType1006;
}
throw new IllegalArgumentException("Unrecognized discriminator:" + this.discriminator);
}
public void setValue(Object value) {
if(value == null) {
log.warn("null passed to setValue function. OneOf's value will not be set.");
return;
}
if(String.class.getName().equals(value.getClass().getName())) {
this.oneOfType1002 = (String) value;
this.discriminator = "String";
this.$objectType = "String";
return;
}
if(Integer.class.getName().equals(value.getClass().getName())) {
this.oneOfType1003 = (Integer) value;
this.discriminator = "Integer";
this.$objectType = "Integer";
return;
}
if(Boolean.class.getName().equals(value.getClass().getName())) {
this.oneOfType1004 = (Boolean) value;
this.discriminator = "Boolean";
this.$objectType = "Boolean";
return;
}
if(setOneOfType1005(value)) {
return;
}
if(setOneOfType1006(value)) {
return;
}
throw new IllegalArgumentException("Attempting to set unsupported object type in OneOfValueWrapper:" + value.getClass().getName());
}
private boolean setOneOfType1005(Object value) {
Class valueClass = String.class;
if(java.util.List.class.isAssignableFrom(value.getClass())) {
// list try to match types if list is not empty.
if (((java.util.List) value).size() > 0) {
// Do some extra type checking
if (valueClass.equals(((java.util.List) value).get(0).getClass())) {
this.discriminator = "List";
this.$objectType = "List";
this.oneOfType1005 = (java.util.List) value;
return true;
}
return false;
} else {
this.discriminator = "EMPTY_LIST";
this.$objectType = "EMPTY_LIST";
this.oneOfType1005 = (List) value;
return true;
}
}
return false;
}
private boolean setOneOfType1006(Object value) {
Class valueClass = String.class;
if(java.util.Map.class.isAssignableFrom(value.getClass())) {
// map try to match types if map is not empty.
if (!((java.util.Map) value).isEmpty()) {
// Do some extra type checking
if (valueClass.equals(((java.util.Map) value).values().toArray()[0].getClass())) {
this.discriminator = "Map";
this.$objectType = "Map";
this.oneOfType1006 = (Map) value;
return true;
}
return false;
} else {
this.discriminator = "EMPTY_MAP";
this.$objectType = "EMPTY_MAP";
this.oneOfType1006 = (Map) value;
return true;
}
}
return false;
}
}
private OneOfValueWrapper value = null;
/**
* @deprecated
* @param value one of wrapper instance
*/
public void setValue(OneOfValueWrapper value) {
log.warn("Deprecating this method, please use setDataInWrapper instead");
if (value == null) {
return;
}
this.value = value;
this.$valueItemDiscriminator = this.value.getDiscriminator();
}
/**
* @param value value of one of field value
*/
@com.fasterxml.jackson.annotation.JsonIgnore
public void setValueInWrapper(Object value) {
if (value == null) {
return;
}
if (this.value == null) {
this.value = new OneOfValueWrapper();
}
this.value.setValue(value);
this.$valueItemDiscriminator = this.value.getDiscriminator();
}
/**
* Get value in one of possible types :
*
* - EMPTY_LIST
* - String
* - Integer
* - Boolean
* - List(String)
*
* @return Object
*/
@com.fasterxml.jackson.databind.annotation.JsonSerialize(using = com.nutanix.dp1.aio.serializers.AioOneOfSerializer.class)
public Object getValue() {
if (this.value == null) {
log.debug("OneOf property value was never set. Returning null...");
return null;
}
return this.value.getValue();
}
@Getter
@JsonView({StandardView.class})
protected final Map $reserved;
@Getter
@JsonView({StandardView.class})
protected final String $objectType;
@Getter
@JsonView({StandardView.class})
protected final Map $unknownFields;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy