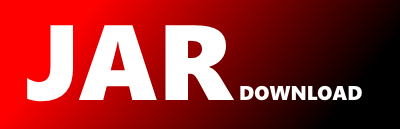
com.nutanix.dp1.sec.iam.v4.authn.User Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of security-java-client Show documentation
Show all versions of security-java-client Show documentation
Manage security features, such as encryption, certificates, or platform hardening.
The newest version!
/*
* Generated file ..
*
* Product version: 4.0.1-beta-1
*
* Part of the Nutanix Security APIs
*
* (c) 2024 Nutanix Inc. All rights reserved
*
*/
package com.nutanix.dp1.sec.iam.v4.authn;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.Setter;
import lombok.AccessLevel;
import com.nutanix.devplatform.models.PrettyModeViews.*;
import com.fasterxml.jackson.annotation.JsonView;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.stream.Collectors;
import com.nutanix.dp1.sec.deserializers.SecObjectTypeTypedObject;
import javax.validation.constraints.*;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import static com.nutanix.dp1.sec.deserializers.SecDeserializerUtils.*;
/**
* Information of the user.
*/
@EqualsAndHashCode(callSuper=true)
@Data
@lombok.extern.slf4j.Slf4j
public class User extends com.nutanix.dp1.sec.common.v1.response.ExternalizableAbstractModel implements java.io.Serializable, SecObjectTypeTypedObject {
public User() {
super();
}
@lombok.Builder(builderMethodName = "UserBuilder")
public User(String tenantId, String extId, java.util.List links, String username, com.nutanix.dp1.sec.iam.v4.authn.UserType userType, String idpId, String displayName, String firstName, String middleInitial, String lastName, String emailId, String locale, String region, String password, Boolean isForceResetPasswordEnabled, java.util.List additionalAttributes, com.nutanix.dp1.sec.iam.v4.authn.UserStatusType status, java.util.List bucketsAccessKeys, java.time.OffsetDateTime lastLoginTime, java.time.OffsetDateTime createdTime, java.time.OffsetDateTime lastUpdatedTime, String createdBy, String lastUpdatedBy, String description, com.nutanix.dp1.sec.iam.v4.authn.CreationType creationType) {
super(tenantId, extId, links);
this.setUsername(username);
this.setUserType(userType);
this.setIdpId(idpId);
this.setDisplayName(displayName);
this.setFirstName(firstName);
this.setMiddleInitial(middleInitial);
this.setLastName(lastName);
this.setEmailId(emailId);
this.setLocale(locale);
this.setRegion(region);
this.setPassword(password);
this.setIsForceResetPasswordEnabled(isForceResetPasswordEnabled);
this.setAdditionalAttributes(additionalAttributes);
this.setStatus(status);
this.setBucketsAccessKeys(bucketsAccessKeys);
this.setLastLoginTime(lastLoginTime);
this.setCreatedTime(createdTime);
this.setLastUpdatedTime(lastUpdatedTime);
this.setCreatedBy(createdBy);
this.setLastUpdatedBy(lastUpdatedBy);
this.setDescription(description);
this.setCreationType(creationType);
}
@Override
protected String initialize$objectType() {
return "iam.v4.authn.User";
}
@Override
protected String initialize$fv() {
return "v4.r0";
}
@javax.validation.constraints.Size(min = 0, max = 5)
private List bucketsAccessKeys = null;
/**
* Bucket access keys for the user.
*/
public void setBucketsAccessKeys(List bucketsAccessKeys) {
if (this.bucketsAccessKeys == null) {
this.bucketsAccessKeys = bucketsAccessKeys;
}
else {
log.warn("Read-only property bucketsAccessKeys already contains a non-null value and cannot be set again");
}
}
private OffsetDateTime lastLoginTime = null;
/**
* The last successful login time for the user.
*/
public void setLastLoginTime(OffsetDateTime lastLoginTime) {
if (this.lastLoginTime == null) {
this.lastLoginTime = lastLoginTime;
}
else {
log.warn("Read-only property lastLoginTime already contains a non-null value and cannot be set again");
}
}
private OffsetDateTime createdTime = null;
/**
* Creation time of the user.
*/
public void setCreatedTime(OffsetDateTime createdTime) {
if (this.createdTime == null) {
this.createdTime = createdTime;
}
else {
log.warn("Read-only property createdTime already contains a non-null value and cannot be set again");
}
}
private OffsetDateTime lastUpdatedTime = null;
/**
* The last updated time for the user.
*/
public void setLastUpdatedTime(OffsetDateTime lastUpdatedTime) {
if (this.lastUpdatedTime == null) {
this.lastUpdatedTime = lastUpdatedTime;
}
else {
log.warn("Read-only property lastUpdatedTime already contains a non-null value and cannot be set again");
}
}
@javax.validation.constraints.Pattern(regexp="^[a-fA-F0-9]{8}-[a-fA-F0-9]{4}-[a-fA-F0-9]{4}-[a-fA-F0-9]{4}-[a-fA-F0-9]{12}$")
private String createdBy = null;
/**
* User or Service who created the user.
*/
public void setCreatedBy(String createdBy) {
if (this.createdBy == null) {
this.createdBy = createdBy;
}
else {
log.warn("Read-only property createdBy already contains a non-null value and cannot be set again");
}
}
@javax.validation.constraints.Pattern(regexp="^[a-fA-F0-9]{8}-[a-fA-F0-9]{4}-[a-fA-F0-9]{4}-[a-fA-F0-9]{4}-[a-fA-F0-9]{12}$")
private String lastUpdatedBy = null;
/**
* Last updated by this user ID.
*/
public void setLastUpdatedBy(String lastUpdatedBy) {
if (this.lastUpdatedBy == null) {
this.lastUpdatedBy = lastUpdatedBy;
}
else {
log.warn("Read-only property lastUpdatedBy already contains a non-null value and cannot be set again");
}
}
/**
* Identifier of the user.
*/
@javax.validation.constraints.Pattern(regexp="^[^<>;'()&+%/\\\\\"`]*$")
@javax.validation.constraints.Size(min = 1, max = 255)
@JsonProperty("username")
public String username = null;
/**
*
*/
@JsonProperty("userType")
public com.nutanix.dp1.sec.iam.v4.authn.UserType userType = null;
/**
* Identifier of the IDP for the user.
*/
@javax.validation.constraints.Pattern(regexp="^[a-fA-F0-9]{8}-[a-fA-F0-9]{4}-[a-fA-F0-9]{4}-[a-fA-F0-9]{4}-[a-fA-F0-9]{12}$")
@JsonProperty("idpId")
public String idpId = null;
/**
* Display name of the user. For LDAP and SAML users, this is set from AD config.
*/
@javax.validation.constraints.Pattern(regexp="^[^<>;'()&+%/\\\\\"`]*$")
@javax.validation.constraints.Size(min = 1, max = 255)
@JsonProperty("displayName")
public String displayName = null;
/**
* First name of the user.
*/
@javax.validation.constraints.Pattern(regexp="^[^<>;'()&+%/\\\\\"`]*$")
@javax.validation.constraints.Size(min = 1, max = 255)
@JsonProperty("firstName")
public String firstName = null;
/**
* Middle name of the user.
*/
@javax.validation.constraints.Pattern(regexp="^[^<>;'()&+%/\\\\\"`]*$")
@javax.validation.constraints.Size(min = 1, max = 255)
@JsonProperty("middleInitial")
public String middleInitial = null;
/**
* Last name of the user.
*/
@javax.validation.constraints.Pattern(regexp="^[^<>;'()&+%/\\\\\"`]*$")
@javax.validation.constraints.Size(min = 1, max = 255)
@JsonProperty("lastName")
public String lastName = null;
/**
* Email ID of the user.
*/
@javax.validation.constraints.Pattern(regexp="^([a-zA-Z0-9_\\-\\.]+)@([a-zA-Z0-9_\\-\\.]+)\\.([a-zA-Z]{2,5})$")
@javax.validation.constraints.Size(max = 255)
@JsonProperty("emailId")
public String emailId = null;
/**
* Default locale of the user.
*/
@JsonProperty("locale")
public String locale = null;
/**
* Default region of the user.
*/
@JsonProperty("region")
public String region = null;
/**
* Password of the user.
*/
@JsonProperty("password")
public String password = null;
/**
* Flag to force the user to reset password.
*/
@JsonProperty("isForceResetPasswordEnabled")
public Boolean isForceResetPasswordEnabled = null;
/**
* Indicates additional attributes of the user.
*/
@javax.validation.constraints.Size(min = 0, max = 10)
@JsonProperty("additionalAttributes")
public List additionalAttributes = null;
/**
*
*/
@JsonProperty("status")
public com.nutanix.dp1.sec.iam.v4.authn.UserStatusType status = null;
/**
* Description of the user.
*/
@JsonProperty("description")
public String description = null;
/**
*
*/
@JsonProperty("creationType")
public com.nutanix.dp1.sec.iam.v4.authn.CreationType creationType = null;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy