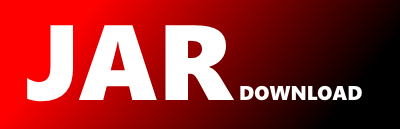
com.nutanix.sec.java.client.api.ApprovalPoliciesApi Maven / Gradle / Ivy
Show all versions of security-java-client Show documentation
package com.nutanix.sec.java.client.api;
import com.nutanix.sec.java.client.ApiClient;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.HashSet;
import java.util.Set;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.InputStreamResource;
import org.springframework.stereotype.Component;
import org.springframework.util.CollectionUtils;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.web.util.UriComponentsBuilder;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.*;
@lombok.extern.slf4j.Slf4j
@javax.annotation.Generated(value = "com.nutanix.swagger.codegen.generators.JavaClientSDKGenerator", date = "2024-12-05T00:55:59.712Z[Etc/UTC]")@Component("com.nutanix.sec.java.client.api.ApprovalPoliciesApi")
public class ApprovalPoliciesApi {
private ApiClient apiClient;
private final Set headersToSkip;
public ApprovalPoliciesApi() {
this(new ApiClient());
}
@Autowired
public ApprovalPoliciesApi(ApiClient apiClient) {
this.apiClient = apiClient;
this.headersToSkip = new HashSet<>(Arrays.asList("authorization", "cookie", "host", "user-agent"));
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Update the list of secured policies linked to an approval policy with particular external identifier.
* 202 - The request to update the list of secured policies is accepted.
*
4XX - Client error response
*
5XX - Server error response
* @param extId Approval policy external identifier.
* @param body The body parameter
* @param args Additional arguments
* @return com.nutanix.dp1.sec.security.v4.management.AssociatePoliciesApiResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public com.nutanix.dp1.sec.security.v4.management.AssociatePoliciesApiResponse associatePolicies(String extId, List body, Map ... args) throws RestClientException {
// Check for optional argument map
Map argMap = args.length > 0 ? args[0] : new HashMap();
Object postBody = body;
// verify the required parameter 'extId' is set
if (extId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'extId' when calling associatePolicies");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'body' when calling associatePolicies");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("extId", extId);
String uriPath = UriComponentsBuilder.fromPath("/api/security/v4.0.b1/management/approval-policies/{extId}/$action/associate-policies").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
argMap.forEach((key, value) -> {
if (!this.headersToSkip.contains(key.toLowerCase())) {
String stringValue = apiClient.parameterToString(value);
if (stringValue != null && !stringValue.trim().isEmpty()) {
headerParams.add(key, apiClient.parameterToString(value));
}
}
});
final String[] accepts = {
"application/json"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = { "application/json" };
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "apiKeyAuthScheme", "basicAuthScheme" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(uriPath, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
* Create an approval policy for secure snapshots.
* 202 - The request to create an approval policy is accepted.
*
4XX - Client error response
*
5XX - Server error response
* @param body Details used to create an approval policy.
* @param args Additional arguments
* @return com.nutanix.dp1.sec.security.v4.management.CreateApprovalPolicyApiResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public com.nutanix.dp1.sec.security.v4.management.CreateApprovalPolicyApiResponse createApprovalPolicy(com.nutanix.dp1.sec.security.v4.management.ApprovalPolicy body, Map ... args) throws RestClientException {
// Check for optional argument map
Map argMap = args.length > 0 ? args[0] : new HashMap();
Object postBody = body;
// verify the required parameter 'body' is set
if (body == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'body' when calling createApprovalPolicy");
}
String uriPath = UriComponentsBuilder.fromPath("/api/security/v4.0.b1/management/approval-policies").build().toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
argMap.forEach((key, value) -> {
if (!this.headersToSkip.contains(key.toLowerCase())) {
String stringValue = apiClient.parameterToString(value);
if (stringValue != null && !stringValue.trim().isEmpty()) {
headerParams.add(key, apiClient.parameterToString(value));
}
}
});
final String[] accepts = {
"application/json"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = { "application/json" };
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "apiKeyAuthScheme", "basicAuthScheme" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(uriPath, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
* Fetches the details of a specific approval policy by its external identifier.
* 200 - Approval policy details.
*
4XX - Client error response
*
5XX - Server error response
* @param extId Approval policy external identifier.
* @param $select A URL query parameter that allows clients to request a specific set of properties for each entity or complex type. Expression specified with the $select must conform to the [OData V4.01](https://docs.oasis-open.org/odata/odata/v4.01/odata-v4.01-part1-protocol.html) URL conventions. If a $select expression consists of a single select item that is an asterisk (i.e., *), then all properties on the matching resource will be returned.
- approverGroups
- description
- isUpdatePending
- name
- securedPolicies
* @param args Additional arguments
* @return com.nutanix.dp1.sec.security.v4.management.GetApprovalPolicyApiResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public com.nutanix.dp1.sec.security.v4.management.GetApprovalPolicyApiResponse getApprovalPolicyByExtId(String extId, String $select, Map ... args) throws RestClientException {
// Check for optional argument map
Map argMap = args.length > 0 ? args[0] : new HashMap();
Object postBody = null;
// verify the required parameter 'extId' is set
if (extId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'extId' when calling getApprovalPolicyByExtId");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("extId", extId);
String uriPath = UriComponentsBuilder.fromPath("/api/security/v4.0.b1/management/approval-policies/{extId}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "$select", $select));
argMap.forEach((key, value) -> {
if (!this.headersToSkip.contains(key.toLowerCase())) {
String stringValue = apiClient.parameterToString(value);
if (stringValue != null && !stringValue.trim().isEmpty()) {
headerParams.add(key, apiClient.parameterToString(value));
}
}
});
final String[] accepts = {
"application/json"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = { };
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "apiKeyAuthScheme", "basicAuthScheme" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(uriPath, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
* Fetches a list of approval policies for secure snapshots.
* 200 - Returns a list of approval policies.
*
4XX - Client error response
*
5XX - Server error response
* @param $select A URL query parameter that allows clients to request a specific set of properties for each entity or complex type. Expression specified with the $select must conform to the [OData V4.01](https://docs.oasis-open.org/odata/odata/v4.01/odata-v4.01-part1-protocol.html) URL conventions. If a $select expression consists of a single select item that is an asterisk (i.e., *), then all properties on the matching resource will be returned.
- approverGroups
- description
- isUpdatePending
- name
- securedPolicies
* @param args Additional arguments
* @return com.nutanix.dp1.sec.security.v4.management.ListApprovalPoliciesApiResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public com.nutanix.dp1.sec.security.v4.management.ListApprovalPoliciesApiResponse listApprovalPolicies(String $select, Map ... args) throws RestClientException {
// Check for optional argument map
Map argMap = args.length > 0 ? args[0] : new HashMap();
Object postBody = null;
String uriPath = UriComponentsBuilder.fromPath("/api/security/v4.0.b1/management/approval-policies").build().toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "$select", $select));
argMap.forEach((key, value) -> {
if (!this.headersToSkip.contains(key.toLowerCase())) {
String stringValue = apiClient.parameterToString(value);
if (stringValue != null && !stringValue.trim().isEmpty()) {
headerParams.add(key, apiClient.parameterToString(value));
}
}
});
final String[] accepts = {
"application/json"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = { };
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "apiKeyAuthScheme", "basicAuthScheme" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(uriPath, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
* Updates the details of a specific approval policy by its external identifier.
* 202 - The request to update an approval policy is accepted.
*
4XX - Client error response
*
5XX - Server error response
* @param extId Approval policy external identifier.
* @param body The body parameter
* @param args Additional arguments
* @return com.nutanix.dp1.sec.security.v4.management.UpdateApprovalPolicyApiResponse
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public com.nutanix.dp1.sec.security.v4.management.UpdateApprovalPolicyApiResponse updateApprovalPolicyByExtId(String extId, com.nutanix.dp1.sec.security.v4.management.ApprovalPolicy body, Map ... args) throws RestClientException {
// Check for optional argument map
Map argMap = args.length > 0 ? args[0] : new HashMap();
Object postBody = body;
// verify the required parameter 'extId' is set
if (extId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'extId' when calling updateApprovalPolicyByExtId");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'body' when calling updateApprovalPolicyByExtId");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("extId", extId);
String uriPath = UriComponentsBuilder.fromPath("/api/security/v4.0.b1/management/approval-policies/{extId}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
argMap.forEach((key, value) -> {
if (!this.headersToSkip.contains(key.toLowerCase())) {
String stringValue = apiClient.parameterToString(value);
if (stringValue != null && !stringValue.trim().isEmpty()) {
headerParams.add(key, apiClient.parameterToString(value));
}
}
});
final String[] accepts = {
"application/json"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = { "application/json" };
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "apiKeyAuthScheme", "basicAuthScheme" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(uriPath, HttpMethod.PUT, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
}