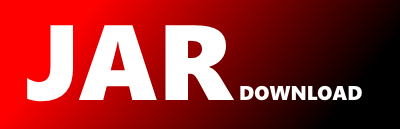
com.ocadotechnology.notification.CrossAppNotificationRouter Maven / Gradle / Ivy
/*
* Copyright © 2017-2023 Ocado (Ocava)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ocadotechnology.notification;
import java.util.function.BiPredicate;
import java.util.function.Consumer;
import java.util.function.Supplier;
import com.google.common.base.Preconditions;
import com.ocadotechnology.event.scheduling.EventScheduler;
import com.ocadotechnology.utils.RememberingSupplier;
public class CrossAppNotificationRouter implements NotificationRouter {
private static class SingletonHolder {
private static final CrossAppNotificationRouter instance = new CrossAppNotificationRouter();
}
public static CrossAppNotificationRouter get() {
return SingletonHolder.instance;
}
/** Singleton */
private CrossAppNotificationRouter(){}
private volatile Consumer eavesDropper = null;
private volatile NotificationLogger logger = null;
private volatile boolean disableBroadcast = false;
public void setEavesDropper(Consumer eavesDropper) {
Preconditions.checkState(this.eavesDropper == null || eavesDropper == null, "Attempting to override eavesdropper: old %s new %s", this.eavesDropper, eavesDropper);
this.eavesDropper = eavesDropper;
}
public void setLogger(NotificationLogger logger) {
Preconditions.checkState(this.logger == null || logger == null, "Attempting to override logger: old %s new %s", this.logger, logger);
this.logger = logger;
}
public void setShouldSuppressBroadcast(boolean disableBroadcast) {
this.disableBroadcast = disableBroadcast;
}
/**
* The defaultMode uses DefaultBus to send all notifications (no forwarding is provided)
* DefaultMode is required for all unit tests. It seems that it is
* the less destructive way of using this class in tests.
*
* DefaultMode is removed with first call to registerExecutionLayer
*
*/
@Override
public void broadcast(T notification) {
RememberingSupplier notificationHolder = new RememberingSupplier<>(notification);
broadcastImplementation(notificationHolder, notification.getClass(), Broadcaster::canHandleNotification);
}
@Override
public void broadcast(Supplier concreteMessageNotificationSupplier, Class notificationClass) {
RememberingSupplier rememberingSupplier = new RememberingSupplier<>(concreteMessageNotificationSupplier);
broadcastImplementation(rememberingSupplier, notificationClass, Broadcaster::isNotificationRegistered);
}
private void broadcastImplementation(
RememberingSupplier messageSupplier,
Class> notificationClass,
BiPredicate, Class>> shouldHandToBroadcaster) {
if (logger != null && logger.accepts(notificationClass)) {
logger.log(messageSupplier.get());
}
if (eavesDropper != null) {
eavesDropper.accept(messageSupplier.get());
}
if (disableBroadcast) {
return;
}
WithinAppNotificationRouter.get().broadcastImplementation(messageSupplier, notificationClass, shouldHandToBroadcaster);
}
public void broadcastWithinAppOnly(Notification message) {
WithinAppNotificationRouter.get().broadcast(message);
}
@Override
public void addHandler(Subscriber handler) {
WithinAppNotificationRouter.get().addHandler(handler);
}
@Override
public synchronized void registerExecutionLayer(EventScheduler scheduler, NotificationBus notificationBus) {
WithinAppNotificationRouter.get().registerExecutionLayer(scheduler, notificationBus);
}
@Override
public void registerExecutionLayer(Broadcaster newBroadcaster) {
WithinAppNotificationRouter.get().registerExecutionLayer(newBroadcaster);
}
@Override
public void clearAllHandlers() {
WithinAppNotificationRouter.get().clearAllHandlers();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy