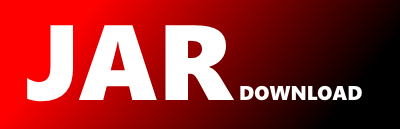
com.oceanprotocol.squid.models.DID Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of squid Show documentation
Show all versions of squid Show documentation
Squid facilitate the interaction of java clients with the Ocean Protocol network
/*
* Copyright 2018 Ocean Protocol Foundation
* SPDX-License-Identifier: Apache-2.0
*/
package com.oceanprotocol.squid.models;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.oceanprotocol.squid.exceptions.DIDFormatException;
import java.util.UUID;
@JsonIgnoreProperties(ignoreUnknown = true)
public class DID {
@JsonProperty
public String did;
public static final String PREFIX = "did:op:";
public DID() {
this.setEmptyDID();
}
public DID(String did) throws DIDFormatException {
setDid(did);
}
public static DID builder() throws DIDFormatException {
return new DID(generateRandomToken());
}
public static DID getFromHash(String hash) throws DIDFormatException {
return new DID(PREFIX + hash);
}
public String getDid() {
return did;
}
public String getHash() {
return did.substring(PREFIX.length());
}
public static String generateRandomToken() {
String token = PREFIX + UUID.randomUUID().toString()
+ UUID.randomUUID().toString();
return token.replaceAll("-", "");
}
@Override
public String toString() {
return did;
}
public DID setEmptyDID() {
this.did = "";
return this;
}
public DID setDid(String did) throws DIDFormatException {
if (!did.startsWith(PREFIX))
throw new DIDFormatException("Invalid DID Format, it should starts by " + PREFIX);
this.did = did;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy