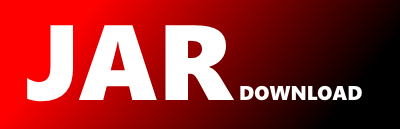
com.ociweb.iot.grove.adc.ADCTwig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of foglight Show documentation
Show all versions of foglight Show documentation
FogLight is a lightweight runtime that enables makers of all ages and skill levels to create highly
performant apps for embedded devices like Raspberry Pi's.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.ociweb.iot.grove.adc;
import com.ociweb.iot.hardware.I2CConnection;
import com.ociweb.iot.hardware.I2CIODevice;
import com.ociweb.iot.maker.FogCommandChannel;
/**
*
* @author huydo
*/
public enum ADCTwig {
;
public enum ADC implements I2CIODevice {
ReadConversionResult(){
@Override
public I2CConnection getI2CConnection() {
byte[] REG_ADDR = {ADC_Constants.REG_ADDR_RESULT};
byte I2C_ADDR = ADC_Constants.ADDR_ADC121;
byte BYTESTOREAD = 2;
byte REG_ID = ADC_Constants.REG_ADDR_RESULT; //just an identifier
return new I2CConnection(this, I2C_ADDR, REG_ADDR, BYTESTOREAD, REG_ID, null);
}
},
ReadAlertStatus(){
@Override
public I2CConnection getI2CConnection() {
byte[] REG_ADDR = {ADC_Constants.REG_ADDR_ALERT};
byte I2C_ADDR = ADC_Constants.ADDR_ADC121;
byte BYTESTOREAD = 1;
byte REG_ID = ADC_Constants.REG_ADDR_ALERT; //just an identifier
return new I2CConnection(this, I2C_ADDR, REG_ADDR, BYTESTOREAD, REG_ID, null);
}
};
@Override
public int defaultPullRateMS() {
return 1000;
}
@Override
public int pullResponseMinWaitNS() {
return 0;
}
@Override
public boolean isInput() {
return true;
}
@Override
public boolean isOutput() {
return false;
}
@Override
public boolean isPWM() {
return false;
}
@Override
public int range() {
return 256;
}
@Override
public boolean isValid(byte[] backing, int position, int length, int mask) {
return true;
}
@Override
public int pinsUsed() {
return 1;
}
@SuppressWarnings("unchecked")
@Override
public ADC_Transducer newTransducer(FogCommandChannel... ch) {
return new ADC_Transducer(ch[0]);
}
}
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy