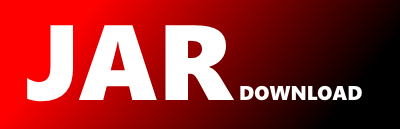
com.ocpsoft.pretty.faces.util.PrettyURLBuilder Maven / Gradle / Ivy
/*
* PrettyFaces is an OpenSource JSF library to create bookmarkable URLs.
* Copyright (C) 2009 - Lincoln Baxter, III This program
* is free software: you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version. This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details. You should have received a copy of the GNU Lesser General
* Public License along with this program. If not, see the file COPYING.LESSER
* or visit the GNU website at .
*/
package com.ocpsoft.pretty.faces.util;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import javax.faces.component.UIComponent;
import javax.faces.component.UIParameter;
import com.ocpsoft.pretty.PrettyException;
import com.ocpsoft.pretty.faces.config.mapping.QueryParameter;
import com.ocpsoft.pretty.faces.config.mapping.UrlMapping;
import com.ocpsoft.pretty.faces.url.QueryString;
import com.ocpsoft.pretty.faces.url.URLPatternParser;
/**
* A utility class for building Pretty URLs.
*
* @author Lincoln Baxter, III
*/
public class PrettyURLBuilder
{
/**
* Extract any {@link UIParameter} objects from a given component. These
* parameters are what PrettyFaces uses to communicate with the JSF component
* tree
*
* @param component
* @return A list of {@link UIParameter} objects, or an empty list if no
* {@link UIParameter}s were contained within the component children.
*/
public List extractParameters(final UIComponent component)
{
List results = new ArrayList();
for (UIComponent child : component.getChildren())
{
if (child instanceof UIParameter)
{
results.add((UIParameter) child);
}
}
return results;
}
/**
* Build a Pretty URL for the given UrlMapping and parameters.
*/
public String build(final UrlMapping mapping, final Map parameters)
{
List list = new ArrayList();
for (Entry e : parameters.entrySet())
{
UIParameter p = new UIParameter();
p.setName(e.getKey());
p.setValue(e.getValue());
list.add(p);
}
return build(mapping, list);
}
/**
* Build a Pretty URL for the given Mapping ID and parameters.
*/
public String build(final UrlMapping urlMapping, final List parameters)
{
if (urlMapping != null)
{
URLPatternParser parser = new URLPatternParser(urlMapping.getPattern());
List pathParams = new ArrayList();
if ((parameters.size() == 1) && ((parameters.get(0).getValue() instanceof List>) || (parameters.get(0).getValue() == null)))
{
return parser.getMappedUrl(parameters.get(0).getValue());
}
List queryParams = new ArrayList();
for (UIParameter parameter : parameters)
{
try
{
String name = parameter.getName();
Object value = parameter.getValue();
if ((name == null) && (value != null))
{
pathParams.add(URLEncoder.encode(value.toString(), "UTF-8"));
}
else
{
List> values = null;
if ((value != null) && value.getClass().isArray())
{
values = Arrays.asList((Object[]) value);
}
else if (value instanceof List>)
{
values = (List>) value;
}
else if (value != null)
{
values = Arrays.asList(value);
}
if (values != null)
{
for (Object object : values)
{
String tempValue = null;
if (object != null)
{
tempValue = object.toString();
}
queryParams.add(new QueryParameter(name, tempValue));
}
}
else
{
queryParams.add(new QueryParameter(name, null));
}
}
}
catch (UnsupportedEncodingException e)
{
throw new PrettyException(e);
}
}
return parser.getMappedUrl(pathParams.toArray()) + QueryString.build(queryParams).toQueryString();
}
return "";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy