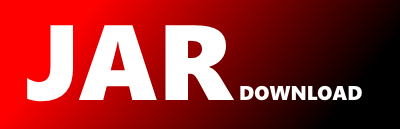
com.ocpsoft.pretty.faces.util.FacesMessagesUtils Maven / Gradle / Ivy
/*
* PrettyFaces is an OpenSource JSF library to create bookmarkable URLs.
* Copyright (C) 2010 - Lincoln Baxter, III This program
* is free software: you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version. This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details. You should have received a copy of the GNU Lesser General
* Public License along with this program. If not, see the file COPYING.LESSER
* or visit the GNU website at .
*/
package com.ocpsoft.pretty.faces.util;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.faces.application.FacesMessage;
import javax.faces.context.FacesContext;
/**
* @author Lincoln Baxter, III
*/
public class FacesMessagesUtils
{
private static final String token = "com.ocpsoft.pretty.SAVED_FACES_MESSAGES";
@SuppressWarnings("unchecked")
public int saveMessages(final FacesContext facesContext, final Map destination)
{
int restoredCount = 0;
if (FacesContext.getCurrentInstance() != null)
{
List messages = new ArrayList();
for (Iterator iter = facesContext.getMessages(null); iter.hasNext();)
{
messages.add(iter.next());
iter.remove();
}
if (messages.size() > 0)
{
List existingMessages = (List) destination.get(token);
if (existingMessages != null)
{
existingMessages.addAll(messages);
}
else
{
destination.put(token, messages);
}
restoredCount = messages.size();
}
}
return restoredCount;
}
@SuppressWarnings("unchecked")
public int restoreMessages(final FacesContext facesContext, final Map source)
{
int restoredCount = 0;
if (FacesContext.getCurrentInstance() != null)
{
List messages = (List) source.remove(token);
if (messages == null)
{
return 0;
}
restoredCount = messages.size();
for (Object element : messages)
{
facesContext.addMessage(null, (FacesMessage) element);
}
}
return restoredCount;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy