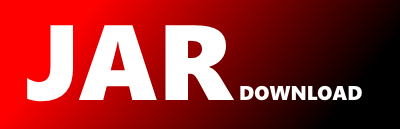
com.offbytwo.jenkins.model.BuildWithDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jenkins-client Show documentation
Show all versions of jenkins-client Show documentation
A Jenkins API client for Java
/*
* Copyright (c) 2013 Rising Oak LLC.
*
* Distributed under the MIT license: http://opensource.org/licenses/MIT
*/
package com.offbytwo.jenkins.model;
import com.google.common.base.Predicate;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static com.google.common.collect.Collections2.filter;
public class BuildWithDetails extends Build {
List actions;
boolean building;
String description;
int duration;
int estimatedDuration;
String fullDisplayName;
String id;
long timestamp;
BuildResult result;
List artifacts;
String consoleOutputText;
String consoleOutputHtml;
public List getArtifacts() {
return artifacts;
}
public boolean isBuilding() {
return building;
}
public String getDescription() {
return description;
}
public int getDuration() {
return duration;
}
public int getEstimatedDuration() {
return estimatedDuration;
}
public String getFullDisplayName() {
return fullDisplayName;
}
public String getId() {
return id;
}
public long getTimestamp() {
return timestamp;
}
public BuildResult getResult() {
return result;
}
public List getActions() {
return actions;
}
public Map getParameters() {
Collection parameters = filter(actions, new Predicate
© 2015 - 2024 Weber Informatics LLC | Privacy Policy