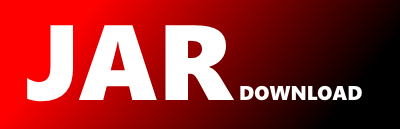
com.offbytwo.jenkins.model.ComputerWithDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jenkins-client Show documentation
Show all versions of jenkins-client Show documentation
A Jenkins API client for Java
/*
* Copyright (c) 2013 Rising Oak LLC.
*
* Distributed under the MIT license: http://opensource.org/licenses/MIT
*/
package com.offbytwo.jenkins.model;
import com.google.common.base.Function;
import com.google.common.collect.Lists;
import java.util.List;
import java.util.Map;
public class ComputerWithDetails extends Job {
String displayName;
List actions;
List executors;
Boolean idle;
Boolean jnlp;
Boolean launchSupported;
Map loadStatistics;
Boolean manualLaunchAllowed;
Map monitorData;
Integer numExecutors;
Boolean offline;
Object offlineCause;
String offlineReason;
List oneOffExecutors;
Boolean temporarilyOffline;
public String getDisplayName() {
return displayName;
}
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy