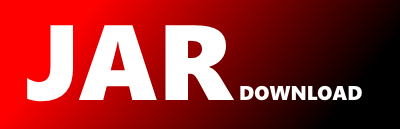
qunar.tc.qconfig.common.support.json.JsonMapper Maven / Gradle / Ivy
The newest version!
package qunar.tc.qconfig.common.support.json;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.Preconditions;
import java.io.IOException;
import java.io.Reader;
import java.io.Writer;
/**
* @author miao.yang [email protected]
* @since 14-4-24.
*/
@SuppressWarnings("unchecked")
public class JsonMapper {
private final ObjectMapper mapper;
public JsonMapper(ObjectMapper mapper) {
this.mapper = mapper;
}
public void writeValue(Writer writer, Object obj) throws IOException {
Preconditions.checkNotNull(writer);
try {
mapper.writeValue(writer, obj);
} catch (JsonProcessingException e) {
throw new RuntimeException("jackson format error: " + obj.getClass(), e);
}
}
public String writeValueAsString(Object obj) {
try {
return mapper.writeValueAsString(obj);
} catch (JsonProcessingException e) {
throw new RuntimeException("jackson format error: " + obj.getClass(), e);
}
}
public T readValue(String json, Class type) {
try {
return mapper.readValue(json, type);
} catch (IOException e) {
throw new RuntimeException("jackson parse error :" + json.substring(0, Math.min(100, json.length())), e);
}
}
public T readValue(Reader reader, Class type) throws IOException {
Preconditions.checkNotNull(reader);
try {
return mapper.readValue(reader, type);
} catch (JsonProcessingException e) {
throw new RuntimeException("jackson parse error.", e);
}
}
public T readValue(String json, TypeReference type) {
try {
return (T) mapper.readValue(json, type);
} catch (Exception e) {
throw new RuntimeException("jackson parse error.", e);
}
}
public T readValue(Reader reader, TypeReference type) throws IOException {
Preconditions.checkNotNull(reader);
try {
return (T) mapper.readValue(reader, type);
} catch (JsonProcessingException e) {
throw new RuntimeException("jackson parse error.", e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy