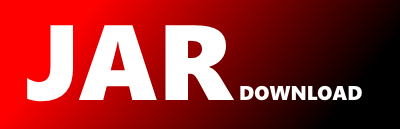
OktaJavaImpl.api.mustache Maven / Gradle / Ivy
{{!
Copyright 2017 Okta
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
}}
{{>licenseInfo}}
package {{package}};
import com.okta.sdk.cache.CacheManager;
import com.okta.sdk.client.AuthenticationScheme;
import com.okta.sdk.client.Proxy;
import com.okta.sdk.impl.api.ClientCredentialsResolver;
import com.okta.sdk.impl.http.authc.RequestAuthenticatorFactory;
import com.okta.sdk.impl.util.BaseUrlResolver;
import com.okta.sdk.impl.http.QueryString;
import com.okta.sdk.impl.config.ClientConfiguration;
import {{vendorExtensions.overrideApiPackage}}.*;
{{#imports}}import {{import}};
{{/imports}}
import com.okta.sdk.impl.ds.InternalDataStore;
import com.okta.sdk.impl.resource.DefaultVoidResource;
import java.util.LinkedHashMap;
{{^fullJavaUtil}}
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
{{/fullJavaUtil}}
import static com.okta.commons.lang.Assert.notNull;
import static com.okta.commons.lang.Assert.hasText;
{{#operations}}
@javax.annotation.Generated(
value = "{{generatorClass}}",
date = "{{generatedDate}}")
@SuppressWarnings("deprecation")
public class {{classname}} extends com.okta.sdk.impl.client.AbstractClient {
/**
* Instantiates a new Client instance that will communicate with the Okta REST API. See the class-level
* JavaDoc for a usage example.
*
* @param clientCredentialsResolver Okta API Key resolver
* @param baseUrlResolver Okta base URL resolver
* @param proxy the HTTP proxy to be used when communicating with the Okta API server (can be
* null)
* @param cacheManager the {@link CacheManager} that should be used to cache
* Okta REST resources (can be null)
* @param authenticationScheme the HTTP authentication scheme to be used when communicating with the Okta API
* @param requestAuthenticatorFactory
* @param connectionTimeout
*/
@Deprecated
public {{classname}}(ClientCredentialsResolver clientCredentialsResolver,
BaseUrlResolver baseUrlResolver,
Proxy proxy,
CacheManager cacheManager,
AuthenticationScheme authenticationScheme,
RequestAuthenticatorFactory requestAuthenticatorFactory,
int connectionTimeout) {
super(clientCredentialsResolver, baseUrlResolver, proxy, cacheManager, authenticationScheme, requestAuthenticatorFactory, connectionTimeout);
}
/**
* Instantiates a new Client instance that will communicate with the Okta REST API. See the class-level
* JavaDoc for a usage example.
*
* @param clientConfiguration the {@link ClientConfiguration} containing the connection information
* @param cacheManager the {@link CacheManager} that should be used to cache
*/
public {{classname}}(ClientConfiguration clientConfiguration, CacheManager cacheManager) {
super(clientConfiguration, cacheManager);
}
{{#operation}}{{^vendorExtensions.moved}}
/**
* {{summary}}
* {{notes}}
{{#allParams}}
* @param {{paramName}} {{description}}{{#required}} (required){{/required}}{{^required}} (optional{{#defaultValue}}, default to {{{.}}}{{/defaultValue}}){{/required}}
{{/allParams}}
{{#returnType}}
* @return {{returnType}}
{{/returnType}}
*/
@Override
@javax.annotation.Generated(
value = "{{generatorClass}}",
date = "{{generatedDate}}",
comments = "{{httpMethod}} - {{path}}")
public {{#returnType}}{{{returnType}}} {{/returnType}}{{^returnType}}void {{/returnType}}{{operationId}}({{#allParams}}{{{dataType}}} {{paramName}}{{#hasMore}}, {{/hasMore}}{{/allParams}}) {
{{>queryPartial}}
{{#vendorExtensions.isGet}}
{{>apiMethodGet}}
{{/vendorExtensions.isGet}}
{{#vendorExtensions.isPut}}
{{>apiMethodPut}}
{{/vendorExtensions.isPut}}
{{#vendorExtensions.isPost}}
{{>apiMethodPostClient}}
{{/vendorExtensions.isPost}}
{{#vendorExtensions.isDelete}}
{{>apiMethodDelete}}
{{/vendorExtensions.isDelete}}
}
{{#vendorExtensions.hasOptional}}
/**
* {{summary}}
* {{notes}}
{{#vendorExtensions.nonOptionalParams}}
* @param {{paramName}} {{description}} (required)
{{/vendorExtensions.nonOptionalParams}}
{{#returnType}}
* @return {{returnType}}
{{/returnType}}
*/
@Override
@javax.annotation.Generated(
value = "{{generatorClass}}",
date = "{{generatedDate}}",
comments = "{{httpMethod}} - {{path}}")
public {{#returnType}}{{{returnType}}} {{/returnType}}{{^returnType}}void {{/returnType}}{{operationId}}({{#vendorExtensions.nonOptionalParams}}{{{dataType}}} {{paramName}}{{#hasMore}}, {{/hasMore}}{{/vendorExtensions.nonOptionalParams}}) {
{{>queryPartialRequired}}
{{#vendorExtensions.isGet}}
{{>apiMethodGet}}
{{/vendorExtensions.isGet}}
{{#vendorExtensions.isPut}}
{{>apiMethodPut}}
{{/vendorExtensions.isPut}}
{{#vendorExtensions.isPost}}
{{#vendorExtensions.optionalBody}}
{{>apiMethodPostNoBody}}
{{/vendorExtensions.optionalBody}}
{{^vendorExtensions.optionalBody}}
{{>apiMethodPostClient}}
{{/vendorExtensions.optionalBody}}
{{/vendorExtensions.isPost}}
{{#vendorExtensions.isDelete}}
{{>apiMethodDelete}}
{{/vendorExtensions.isDelete}}
}
{{/vendorExtensions.hasOptional}}
{{#vendorExtensions.hasBackwardsCompatibleParams}}
/**
* {{summary}}
* {{notes}}
{{#vendorExtensions.onlyBackwardsCompatibleParams}}
* @param {{paramName}} {{description}} (required)
{{/vendorExtensions.onlyBackwardsCompatibleParams}}
{{#returnType}}
* @return {{returnType}}
{{/returnType}}
*/
@Override
@javax.annotation.Generated(
value = "{{generatorClass}}",
date = "{{generatedDate}}",
comments = "{{httpMethod}} - {{path}}")
public {{#returnType}}{{{returnType}}} {{/returnType}}{{^returnType}}void {{/returnType}}{{operationId}}({{#vendorExtensions.onlyBackwardsCompatibleParams}}{{{dataType}}} {{paramName}}{{#hasMore}}, {{/hasMore}}{{/vendorExtensions.onlyBackwardsCompatibleParams}}) {
{{>queryPartialBackwardsCompat}}
{{#vendorExtensions.isGet}}
{{>apiMethodGet}}
{{/vendorExtensions.isGet}}
{{#vendorExtensions.isPut}}
{{>apiMethodPut}}
{{/vendorExtensions.isPut}}
{{#vendorExtensions.isPost}}
{{>apiMethodPostClient}}
{{/vendorExtensions.isPost}}
{{#vendorExtensions.isDelete}}
{{>apiMethodDelete}}
{{/vendorExtensions.isDelete}}
}
{{/vendorExtensions.hasBackwardsCompatibleParams}}
{{/vendorExtensions.moved}}
{{/operation}}
}
{{/operations}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy