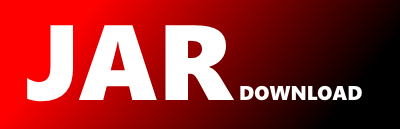
com.okta.sdk.resource.api.AgentPoolsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.AgentPool;
import com.okta.sdk.resource.model.AgentPoolUpdate;
import com.okta.sdk.resource.model.AgentPoolUpdateSetting;
import com.okta.sdk.resource.model.AgentType;
import com.okta.sdk.resource.model.Error;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class AgentPoolsApi {
private ApiClient apiClient;
public AgentPoolsApi() {
this(Configuration.getDefaultApiClient());
}
public AgentPoolsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Activate an Agent Pool update Activates scheduled Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate activateAgentPoolsUpdate(String poolId, String updateId) throws ApiException {
return this.activateAgentPoolsUpdate(poolId, updateId, Collections.emptyMap());
}
/**
* Activate an Agent Pool update Activates scheduled Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate activateAgentPoolsUpdate(String poolId, String updateId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400,
"Missing the required parameter 'poolId' when calling activateAgentPoolsUpdate");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400,
"Missing the required parameter 'updateId' when calling activateAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}/activate"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create an Agent Pool update Creates an Agent pool update \\n For user flow 2 manual update, starts the update
* immediately. \\n For user flow 3, schedules the update based on the configured update window and delay.
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param agentPoolUpdate
* (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate createAgentPoolsUpdate(String poolId, AgentPoolUpdate agentPoolUpdate) throws ApiException {
return this.createAgentPoolsUpdate(poolId, agentPoolUpdate, Collections.emptyMap());
}
/**
* Create an Agent Pool update Creates an Agent pool update \\n For user flow 2 manual update, starts the update
* immediately. \\n For user flow 3, schedules the update based on the configured update window and delay.
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param agentPoolUpdate
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate createAgentPoolsUpdate(String poolId, AgentPoolUpdate agentPoolUpdate,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = agentPoolUpdate;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling createAgentPoolsUpdate");
}
// verify the required parameter 'agentPoolUpdate' is set
if (agentPoolUpdate == null) {
throw new ApiException(400,
"Missing the required parameter 'agentPoolUpdate' when calling createAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates".replaceAll("\\{" + "poolId" + "\\}",
apiClient.escapeString(poolId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Deactivate an Agent Pool update Deactivates scheduled Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate deactivateAgentPoolsUpdate(String poolId, String updateId) throws ApiException {
return this.deactivateAgentPoolsUpdate(poolId, updateId, Collections.emptyMap());
}
/**
* Deactivate an Agent Pool update Deactivates scheduled Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate deactivateAgentPoolsUpdate(String poolId, String updateId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400,
"Missing the required parameter 'poolId' when calling deactivateAgentPoolsUpdate");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400,
"Missing the required parameter 'updateId' when calling deactivateAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}/deactivate"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Delete an Agent Pool update Deletes Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deleteAgentPoolsUpdate(String poolId, String updateId) throws ApiException {
this.deleteAgentPoolsUpdate(poolId, updateId, Collections.emptyMap());
}
/**
* Delete an Agent Pool update Deletes Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deleteAgentPoolsUpdate(String poolId, String updateId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling deleteAgentPoolsUpdate");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400,
"Missing the required parameter 'updateId' when calling deleteAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Retrieve an Agent Pool update by id Retrieves Agent pool update from updateId
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate getAgentPoolsUpdateInstance(String poolId, String updateId) throws ApiException {
return this.getAgentPoolsUpdateInstance(poolId, updateId, Collections.emptyMap());
}
/**
* Retrieve an Agent Pool update by id Retrieves Agent pool update from updateId
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate getAgentPoolsUpdateInstance(String poolId, String updateId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400,
"Missing the required parameter 'poolId' when calling getAgentPoolsUpdateInstance");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400,
"Missing the required parameter 'updateId' when calling getAgentPoolsUpdateInstance");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve an Agent Pool update's settings Retrieves the current state of the agent pool update instance
* settings
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
*
* @return AgentPoolUpdateSetting
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdateSetting getAgentPoolsUpdateSettings(String poolId) throws ApiException {
return this.getAgentPoolsUpdateSettings(poolId, Collections.emptyMap());
}
/**
* Retrieve an Agent Pool update's settings Retrieves the current state of the agent pool update instance
* settings
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdateSetting
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdateSetting getAgentPoolsUpdateSettings(String poolId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400,
"Missing the required parameter 'poolId' when calling getAgentPoolsUpdateSettings");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/settings".replaceAll("\\{" + "poolId" + "\\}",
apiClient.escapeString(poolId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Agent Pools Lists all agent pools with pagination support
*
* @param limitPerPoolType
* Maximum number of AgentPools being returned (optional, default to 5)
* @param poolType
* Agent type to search for (optional)
* @param after
* The cursor to use for pagination. It is an opaque string that specifies your current location in the
* list and is obtained from the `Link` response header. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
*
* @return List<AgentPool>
*
* @throws ApiException
* if fails to make API call
*/
public List listAgentPools(Integer limitPerPoolType, AgentType poolType, String after)
throws ApiException {
return this.listAgentPools(limitPerPoolType, poolType, after, Collections.emptyMap());
}
/**
* List all Agent Pools Lists all agent pools with pagination support
*
* @param limitPerPoolType
* Maximum number of AgentPools being returned (optional, default to 5)
* @param poolType
* Agent type to search for (optional)
* @param after
* The cursor to use for pagination. It is an opaque string that specifies your current location in the
* list and is obtained from the `Link` response header. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<AgentPool>
*
* @throws ApiException
* if fails to make API call
*/
public List listAgentPools(Integer limitPerPoolType, AgentType poolType, String after,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/agentPools";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("limitPerPoolType", limitPerPoolType));
localVarQueryParams.addAll(apiClient.parameterToPair("poolType", poolType));
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Agent Pool updates Lists all agent pool updates
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param scheduled
* Scope the list only to scheduled or ad-hoc updates. If the parameter is not provided we will return
* the whole list of updates. (optional)
*
* @return List<AgentPoolUpdate>
*
* @throws ApiException
* if fails to make API call
*/
public List listAgentPoolsUpdates(String poolId, Boolean scheduled) throws ApiException {
return this.listAgentPoolsUpdates(poolId, scheduled, Collections.emptyMap());
}
/**
* List all Agent Pool updates Lists all agent pool updates
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param scheduled
* Scope the list only to scheduled or ad-hoc updates. If the parameter is not provided we will return
* the whole list of updates. (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<AgentPoolUpdate>
*
* @throws ApiException
* if fails to make API call
*/
public List listAgentPoolsUpdates(String poolId, Boolean scheduled,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling listAgentPoolsUpdates");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates".replaceAll("\\{" + "poolId" + "\\}",
apiClient.escapeString(poolId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("scheduled", scheduled));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Pause an Agent Pool update Pauses running or queued Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate pauseAgentPoolsUpdate(String poolId, String updateId) throws ApiException {
return this.pauseAgentPoolsUpdate(poolId, updateId, Collections.emptyMap());
}
/**
* Pause an Agent Pool update Pauses running or queued Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate pauseAgentPoolsUpdate(String poolId, String updateId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling pauseAgentPoolsUpdate");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400, "Missing the required parameter 'updateId' when calling pauseAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}/pause"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Resume an Agent Pool update Resumes running or queued Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate resumeAgentPoolsUpdate(String poolId, String updateId) throws ApiException {
return this.resumeAgentPoolsUpdate(poolId, updateId, Collections.emptyMap());
}
/**
* Resume an Agent Pool update Resumes running or queued Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate resumeAgentPoolsUpdate(String poolId, String updateId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling resumeAgentPoolsUpdate");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400,
"Missing the required parameter 'updateId' when calling resumeAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}/resume"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retry an Agent Pool update Retries Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate retryAgentPoolsUpdate(String poolId, String updateId) throws ApiException {
return this.retryAgentPoolsUpdate(poolId, updateId, Collections.emptyMap());
}
/**
* Retry an Agent Pool update Retries Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate retryAgentPoolsUpdate(String poolId, String updateId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling retryAgentPoolsUpdate");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400, "Missing the required parameter 'updateId' when calling retryAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}/retry"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Stop an Agent Pool update Stops Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate stopAgentPoolsUpdate(String poolId, String updateId) throws ApiException {
return this.stopAgentPoolsUpdate(poolId, updateId, Collections.emptyMap());
}
/**
* Stop an Agent Pool update Stops Agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate stopAgentPoolsUpdate(String poolId, String updateId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling stopAgentPoolsUpdate");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400, "Missing the required parameter 'updateId' when calling stopAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}/stop"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Update an Agent Pool update by id Updates Agent pool update and return latest agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param agentPoolUpdate
* (required)
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate updateAgentPoolsUpdate(String poolId, String updateId, AgentPoolUpdate agentPoolUpdate)
throws ApiException {
return this.updateAgentPoolsUpdate(poolId, updateId, agentPoolUpdate, Collections.emptyMap());
}
/**
* Update an Agent Pool update by id Updates Agent pool update and return latest agent pool update
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param updateId
* Id of the update (required)
* @param agentPoolUpdate
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdate
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdate updateAgentPoolsUpdate(String poolId, String updateId, AgentPoolUpdate agentPoolUpdate,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = agentPoolUpdate;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling updateAgentPoolsUpdate");
}
// verify the required parameter 'updateId' is set
if (updateId == null) {
throw new ApiException(400,
"Missing the required parameter 'updateId' when calling updateAgentPoolsUpdate");
}
// verify the required parameter 'agentPoolUpdate' is set
if (agentPoolUpdate == null) {
throw new ApiException(400,
"Missing the required parameter 'agentPoolUpdate' when calling updateAgentPoolsUpdate");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/{updateId}"
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()))
.replaceAll("\\{" + "updateId" + "\\}", apiClient.escapeString(updateId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Update an Agent Pool update settings Updates an agent pool update settings
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param agentPoolUpdateSetting
* (required)
*
* @return AgentPoolUpdateSetting
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdateSetting updateAgentPoolsUpdateSettings(String poolId,
AgentPoolUpdateSetting agentPoolUpdateSetting) throws ApiException {
return this.updateAgentPoolsUpdateSettings(poolId, agentPoolUpdateSetting, Collections.emptyMap());
}
/**
* Update an Agent Pool update settings Updates an agent pool update settings
*
* @param poolId
* Id of the agent pool for which the settings will apply (required)
* @param agentPoolUpdateSetting
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AgentPoolUpdateSetting
*
* @throws ApiException
* if fails to make API call
*/
public AgentPoolUpdateSetting updateAgentPoolsUpdateSettings(String poolId,
AgentPoolUpdateSetting agentPoolUpdateSetting, Map additionalHeaders) throws ApiException {
Object localVarPostBody = agentPoolUpdateSetting;
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400,
"Missing the required parameter 'poolId' when calling updateAgentPoolsUpdateSettings");
}
// verify the required parameter 'agentPoolUpdateSetting' is set
if (agentPoolUpdateSetting == null) {
throw new ApiException(400,
"Missing the required parameter 'agentPoolUpdateSetting' when calling updateAgentPoolsUpdateSettings");
}
// create path and map variables
String localVarPath = "/api/v1/agentPools/{poolId}/updates/settings".replaceAll("\\{" + "poolId" + "\\}",
apiClient.escapeString(poolId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy