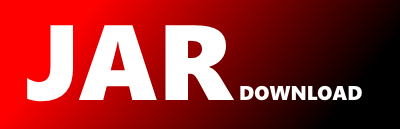
com.okta.sdk.resource.api.ApplicationUsersApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.AppUser;
import com.okta.sdk.resource.model.AppUserAssignRequest;
import com.okta.sdk.resource.model.AppUserUpdateRequest;
import com.okta.sdk.resource.model.Error;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ApplicationUsersApi {
private ApiClient apiClient;
public ApplicationUsersApi() {
this(Configuration.getDefaultApiClient());
}
public ApplicationUsersApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Assign an Application User Assigns a user to an app for: * SSO only<br> Assignments to SSO apps typically
* don't include a user profile. However, if your SSO app requires a profile but doesn't have provisioning
* enabled, you can add profile attributes in the request body. * SSO and provisioning<br> Assignments to SSO
* and provisioning apps typically include credentials and an app-specific profile. Profile mappings defined for the
* app are applied first before applying any profile properties that are specified in the request body. >
* **Notes:** > * When Universal Directory is enabled, you can only specify profile properties that aren't
* defined in profile mappings. > * Omit mapped properties during assignment to minimize assignment errors.
*
* @param appId
* Application ID (required)
* @param appUser
* (required)
*
* @return AppUser
*
* @throws ApiException
* if fails to make API call
*/
public AppUser assignUserToApplication(String appId, AppUserAssignRequest appUser) throws ApiException {
return this.assignUserToApplication(appId, appUser, Collections.emptyMap());
}
/**
* Assign an Application User Assigns a user to an app for: * SSO only<br> Assignments to SSO apps typically
* don't include a user profile. However, if your SSO app requires a profile but doesn't have provisioning
* enabled, you can add profile attributes in the request body. * SSO and provisioning<br> Assignments to SSO
* and provisioning apps typically include credentials and an app-specific profile. Profile mappings defined for the
* app are applied first before applying any profile properties that are specified in the request body. >
* **Notes:** > * When Universal Directory is enabled, you can only specify profile properties that aren't
* defined in profile mappings. > * Omit mapped properties during assignment to minimize assignment errors.
*
* @param appId
* Application ID (required)
* @param appUser
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AppUser
*
* @throws ApiException
* if fails to make API call
*/
public AppUser assignUserToApplication(String appId, AppUserAssignRequest appUser,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = appUser;
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(400, "Missing the required parameter 'appId' when calling assignUserToApplication");
}
// verify the required parameter 'appUser' is set
if (appUser == null) {
throw new ApiException(400,
"Missing the required parameter 'appUser' when calling assignUserToApplication");
}
// create path and map variables
String localVarPath = "/api/v1/apps/{appId}/users".replaceAll("\\{" + "appId" + "\\}",
apiClient.escapeString(appId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve an Application User Retrieves a specific user assignment for a specific app
*
* @param appId
* Application ID (required)
* @param userId
* ID of an existing Okta user (required)
* @param expand
* An optional query parameter to return the corresponding
* [User](/openapi/okta-management/management/tag/User/) object in the `_embedded` property.
* Valid value: `user` (optional)
*
* @return AppUser
*
* @throws ApiException
* if fails to make API call
*/
public AppUser getApplicationUser(String appId, String userId, String expand) throws ApiException {
return this.getApplicationUser(appId, userId, expand, Collections.emptyMap());
}
/**
* Retrieve an Application User Retrieves a specific user assignment for a specific app
*
* @param appId
* Application ID (required)
* @param userId
* ID of an existing Okta user (required)
* @param expand
* An optional query parameter to return the corresponding
* [User](/openapi/okta-management/management/tag/User/) object in the `_embedded` property.
* Valid value: `user` (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AppUser
*
* @throws ApiException
* if fails to make API call
*/
public AppUser getApplicationUser(String appId, String userId, String expand, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(400, "Missing the required parameter 'appId' when calling getApplicationUser");
}
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling getApplicationUser");
}
// create path and map variables
String localVarPath = "/api/v1/apps/{appId}/users/{userId}"
.replaceAll("\\{" + "appId" + "\\}", apiClient.escapeString(appId.toString()))
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("expand", expand));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Application Users Lists all assigned users for an app
*
* @param appId
* Application ID (required)
* @param after
* Specifies the pagination cursor for the next page of results. Treat this as an opaque value obtained
* through the next link relationship. See [Pagination](/#pagination). (optional)
* @param limit
* Specifies the number of objects to return per page. If there are multiple pages of results, the Link
* header contains a `next` link that you need to use as an opaque value (follow it, don't
* parse it). See [Pagination](/#pagination). (optional, default to 50)
* @param q
* Specifies a filter for the list of Application Users returned based on their profile attributes. The
* value of `q` is matched against the beginning of the following profile attributes:
* `userName`, `firstName`, `lastName`, and `email`. This filter
* only supports the `startsWith` operation that matches the `q` string against the
* beginning of the attribute values. > **Note:** For OIDC apps, user profiles don't contain the
* `firstName` or `lastName` attributes. Therefore, the query only matches against
* the `userName` or `email` attributes. (optional)
* @param expand
* An optional query parameter to return the corresponding
* [User](/openapi/okta-management/management/tag/User/) object in the `_embedded` property.
* Valid value: `user` (optional)
*
* @return List<AppUser>
*
* @throws ApiException
* if fails to make API call
*/
public List listApplicationUsers(String appId, String after, Integer limit, String q, String expand)
throws ApiException {
return this.listApplicationUsers(appId, after, limit, q, expand, Collections.emptyMap());
}
/**
* List all Application Users Lists all assigned users for an app
*
* @param appId
* Application ID (required)
* @param after
* Specifies the pagination cursor for the next page of results. Treat this as an opaque value obtained
* through the next link relationship. See [Pagination](/#pagination). (optional)
* @param limit
* Specifies the number of objects to return per page. If there are multiple pages of results, the Link
* header contains a `next` link that you need to use as an opaque value (follow it, don't
* parse it). See [Pagination](/#pagination). (optional, default to 50)
* @param q
* Specifies a filter for the list of Application Users returned based on their profile attributes. The
* value of `q` is matched against the beginning of the following profile attributes:
* `userName`, `firstName`, `lastName`, and `email`. This filter
* only supports the `startsWith` operation that matches the `q` string against the
* beginning of the attribute values. > **Note:** For OIDC apps, user profiles don't contain the
* `firstName` or `lastName` attributes. Therefore, the query only matches against
* the `userName` or `email` attributes. (optional)
* @param expand
* An optional query parameter to return the corresponding
* [User](/openapi/okta-management/management/tag/User/) object in the `_embedded` property.
* Valid value: `user` (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<AppUser>
*
* @throws ApiException
* if fails to make API call
*/
public List listApplicationUsers(String appId, String after, Integer limit, String q, String expand,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(400, "Missing the required parameter 'appId' when calling listApplicationUsers");
}
// create path and map variables
String localVarPath = "/api/v1/apps/{appId}/users".replaceAll("\\{" + "appId" + "\\}",
apiClient.escapeString(appId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPair("q", q));
localVarQueryParams.addAll(apiClient.parameterToPair("expand", expand));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Unassign an Application User Unassigns a user from an app For directories like Active Directory and LDAP, they
* act as the owner of the user's credential with Okta delegating authentication (DelAuth) to that directory. If
* this request is successful for a user when DelAuth is enabled, then the user is in a state with no password. You
* can then reset the user's password. > **Important:** This is a destructive operation. You can't
* recover the user's app profile. If the app is enabled for provisioning and configured to deactivate users,
* the user is also deactivated in the target app.
*
* @param appId
* Application ID (required)
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* Sends a deactivation email to the administrator if `true` (optional, default to false)
*
* @throws ApiException
* if fails to make API call
*/
public void unassignUserFromApplication(String appId, String userId, Boolean sendEmail) throws ApiException {
this.unassignUserFromApplication(appId, userId, sendEmail, Collections.emptyMap());
}
/**
* Unassign an Application User Unassigns a user from an app For directories like Active Directory and LDAP, they
* act as the owner of the user's credential with Okta delegating authentication (DelAuth) to that directory. If
* this request is successful for a user when DelAuth is enabled, then the user is in a state with no password. You
* can then reset the user's password. > **Important:** This is a destructive operation. You can't
* recover the user's app profile. If the app is enabled for provisioning and configured to deactivate users,
* the user is also deactivated in the target app.
*
* @param appId
* Application ID (required)
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* Sends a deactivation email to the administrator if `true` (optional, default to false)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void unassignUserFromApplication(String appId, String userId, Boolean sendEmail,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(400,
"Missing the required parameter 'appId' when calling unassignUserFromApplication");
}
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400,
"Missing the required parameter 'userId' when calling unassignUserFromApplication");
}
// create path and map variables
String localVarPath = "/api/v1/apps/{appId}/users/{userId}"
.replaceAll("\\{" + "appId" + "\\}", apiClient.escapeString(appId.toString()))
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("sendEmail", sendEmail));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Update an Application User Updates the profile or credentials of a user assigned to an app
*
* @param appId
* Application ID (required)
* @param userId
* ID of an existing Okta user (required)
* @param appUser
* (required)
*
* @return AppUser
*
* @throws ApiException
* if fails to make API call
*/
public AppUser updateApplicationUser(String appId, String userId, AppUserUpdateRequest appUser)
throws ApiException {
return this.updateApplicationUser(appId, userId, appUser, Collections.emptyMap());
}
/**
* Update an Application User Updates the profile or credentials of a user assigned to an app
*
* @param appId
* Application ID (required)
* @param userId
* ID of an existing Okta user (required)
* @param appUser
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return AppUser
*
* @throws ApiException
* if fails to make API call
*/
public AppUser updateApplicationUser(String appId, String userId, AppUserUpdateRequest appUser,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = appUser;
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(400, "Missing the required parameter 'appId' when calling updateApplicationUser");
}
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling updateApplicationUser");
}
// verify the required parameter 'appUser' is set
if (appUser == null) {
throw new ApiException(400, "Missing the required parameter 'appUser' when calling updateApplicationUser");
}
// create path and map variables
String localVarPath = "/api/v1/apps/{appId}/users/{userId}"
.replaceAll("\\{" + "appId" + "\\}", apiClient.escapeString(appId.toString()))
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy