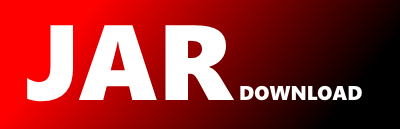
com.okta.sdk.resource.api.CaptchaApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.CAPTCHAInstance;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.OrgCAPTCHASettings;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class CaptchaApi {
private ApiClient apiClient;
public CaptchaApi() {
this(Configuration.getDefaultApiClient());
}
public CaptchaApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Create a CAPTCHA instance Creates a new CAPTCHA instance. Currently, an org can only configure a single CAPTCHA
* instance.
*
* @param instance
* (required)
*
* @return CAPTCHAInstance
*
* @throws ApiException
* if fails to make API call
*/
public CAPTCHAInstance createCaptchaInstance(CAPTCHAInstance instance) throws ApiException {
return this.createCaptchaInstance(instance, Collections.emptyMap());
}
/**
* Create a CAPTCHA instance Creates a new CAPTCHA instance. Currently, an org can only configure a single CAPTCHA
* instance.
*
* @param instance
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return CAPTCHAInstance
*
* @throws ApiException
* if fails to make API call
*/
public CAPTCHAInstance createCaptchaInstance(CAPTCHAInstance instance, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = instance;
// verify the required parameter 'instance' is set
if (instance == null) {
throw new ApiException(400, "Missing the required parameter 'instance' when calling createCaptchaInstance");
}
// create path and map variables
String localVarPath = "/api/v1/captchas";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Delete a CAPTCHA Instance Deletes a specified CAPTCHA instance > **Note:** If your CAPTCHA instance is still
* associated with your org, the request fails. You must first update your Org-wide CAPTCHA settings to remove the
* CAPTCHA instance.
*
* @param captchaId
* The unique key used to identify your CAPTCHA instance (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deleteCaptchaInstance(String captchaId) throws ApiException {
this.deleteCaptchaInstance(captchaId, Collections.emptyMap());
}
/**
* Delete a CAPTCHA Instance Deletes a specified CAPTCHA instance > **Note:** If your CAPTCHA instance is still
* associated with your org, the request fails. You must first update your Org-wide CAPTCHA settings to remove the
* CAPTCHA instance.
*
* @param captchaId
* The unique key used to identify your CAPTCHA instance (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deleteCaptchaInstance(String captchaId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'captchaId' is set
if (captchaId == null) {
throw new ApiException(400,
"Missing the required parameter 'captchaId' when calling deleteCaptchaInstance");
}
// create path and map variables
String localVarPath = "/api/v1/captchas/{captchaId}".replaceAll("\\{" + "captchaId" + "\\}",
apiClient.escapeString(captchaId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete the Org-wide CAPTCHA Settings Deletes the CAPTCHA settings object for your organization
*
* @throws ApiException
* if fails to make API call
*/
public void deleteOrgCaptchaSettings() throws ApiException {
this.deleteOrgCaptchaSettings(Collections.emptyMap());
}
/**
* Delete the Org-wide CAPTCHA Settings Deletes the CAPTCHA settings object for your organization
*
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deleteOrgCaptchaSettings(Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/org/captcha";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Retrieve a CAPTCHA Instance Retrieves the properties of a specified CAPTCHA instance
*
* @param captchaId
* The unique key used to identify your CAPTCHA instance (required)
*
* @return CAPTCHAInstance
*
* @throws ApiException
* if fails to make API call
*/
public CAPTCHAInstance getCaptchaInstance(String captchaId) throws ApiException {
return this.getCaptchaInstance(captchaId, Collections.emptyMap());
}
/**
* Retrieve a CAPTCHA Instance Retrieves the properties of a specified CAPTCHA instance
*
* @param captchaId
* The unique key used to identify your CAPTCHA instance (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return CAPTCHAInstance
*
* @throws ApiException
* if fails to make API call
*/
public CAPTCHAInstance getCaptchaInstance(String captchaId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'captchaId' is set
if (captchaId == null) {
throw new ApiException(400, "Missing the required parameter 'captchaId' when calling getCaptchaInstance");
}
// create path and map variables
String localVarPath = "/api/v1/captchas/{captchaId}".replaceAll("\\{" + "captchaId" + "\\}",
apiClient.escapeString(captchaId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Org-wide CAPTCHA Settings Retrieves the CAPTCHA settings object for your organization > **Note**:
* If the current organization hasn't configured CAPTCHA Settings, the request returns an empty object.
*
* @return OrgCAPTCHASettings
*
* @throws ApiException
* if fails to make API call
*/
public OrgCAPTCHASettings getOrgCaptchaSettings() throws ApiException {
return this.getOrgCaptchaSettings(Collections.emptyMap());
}
/**
* Retrieve the Org-wide CAPTCHA Settings Retrieves the CAPTCHA settings object for your organization > **Note**:
* If the current organization hasn't configured CAPTCHA Settings, the request returns an empty object.
*
* @param additionalHeaders
* additionalHeaders for this call
*
* @return OrgCAPTCHASettings
*
* @throws ApiException
* if fails to make API call
*/
public OrgCAPTCHASettings getOrgCaptchaSettings(Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/org/captcha";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all CAPTCHA Instances Lists all CAPTCHA instances with pagination support. A subset of CAPTCHA instances can
* be returned that match a supported filter expression or query.
*
* @return List<CAPTCHAInstance>
*
* @throws ApiException
* if fails to make API call
*/
public List listCaptchaInstances() throws ApiException {
return this.listCaptchaInstances(Collections.emptyMap());
}
/**
* List all CAPTCHA Instances Lists all CAPTCHA instances with pagination support. A subset of CAPTCHA instances can
* be returned that match a supported filter expression or query.
*
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<CAPTCHAInstance>
*
* @throws ApiException
* if fails to make API call
*/
public List listCaptchaInstances(Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/captchas";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace a CAPTCHA Instance Replaces the properties for a specified CAPTCHA instance
*
* @param captchaId
* The unique key used to identify your CAPTCHA instance (required)
* @param instance
* (required)
*
* @return CAPTCHAInstance
*
* @throws ApiException
* if fails to make API call
*/
public CAPTCHAInstance replaceCaptchaInstance(String captchaId, CAPTCHAInstance instance) throws ApiException {
return this.replaceCaptchaInstance(captchaId, instance, Collections.emptyMap());
}
/**
* Replace a CAPTCHA Instance Replaces the properties for a specified CAPTCHA instance
*
* @param captchaId
* The unique key used to identify your CAPTCHA instance (required)
* @param instance
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return CAPTCHAInstance
*
* @throws ApiException
* if fails to make API call
*/
public CAPTCHAInstance replaceCaptchaInstance(String captchaId, CAPTCHAInstance instance,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = instance;
// verify the required parameter 'captchaId' is set
if (captchaId == null) {
throw new ApiException(400,
"Missing the required parameter 'captchaId' when calling replaceCaptchaInstance");
}
// verify the required parameter 'instance' is set
if (instance == null) {
throw new ApiException(400,
"Missing the required parameter 'instance' when calling replaceCaptchaInstance");
}
// create path and map variables
String localVarPath = "/api/v1/captchas/{captchaId}".replaceAll("\\{" + "captchaId" + "\\}",
apiClient.escapeString(captchaId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace the Org-wide CAPTCHA Settings Replaces the CAPTCHA settings object for your organization > **Note**:
* You can disable CAPTCHA for your organization by setting `captchaId` and `enabledPages` to
* `null`.
*
* @param orgCAPTCHASettings
* (required)
*
* @return OrgCAPTCHASettings
*
* @throws ApiException
* if fails to make API call
*/
public OrgCAPTCHASettings replacesOrgCaptchaSettings(OrgCAPTCHASettings orgCAPTCHASettings) throws ApiException {
return this.replacesOrgCaptchaSettings(orgCAPTCHASettings, Collections.emptyMap());
}
/**
* Replace the Org-wide CAPTCHA Settings Replaces the CAPTCHA settings object for your organization > **Note**:
* You can disable CAPTCHA for your organization by setting `captchaId` and `enabledPages` to
* `null`.
*
* @param orgCAPTCHASettings
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return OrgCAPTCHASettings
*
* @throws ApiException
* if fails to make API call
*/
public OrgCAPTCHASettings replacesOrgCaptchaSettings(OrgCAPTCHASettings orgCAPTCHASettings,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = orgCAPTCHASettings;
// verify the required parameter 'orgCAPTCHASettings' is set
if (orgCAPTCHASettings == null) {
throw new ApiException(400,
"Missing the required parameter 'orgCAPTCHASettings' when calling replacesOrgCaptchaSettings");
}
// create path and map variables
String localVarPath = "/api/v1/org/captcha";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Update a CAPTCHA Instance Partially updates the properties of a specified CAPTCHA instance
*
* @param captchaId
* The unique key used to identify your CAPTCHA instance (required)
* @param instance
* (required)
*
* @return CAPTCHAInstance
*
* @throws ApiException
* if fails to make API call
*/
public CAPTCHAInstance updateCaptchaInstance(String captchaId, CAPTCHAInstance instance) throws ApiException {
return this.updateCaptchaInstance(captchaId, instance, Collections.emptyMap());
}
/**
* Update a CAPTCHA Instance Partially updates the properties of a specified CAPTCHA instance
*
* @param captchaId
* The unique key used to identify your CAPTCHA instance (required)
* @param instance
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return CAPTCHAInstance
*
* @throws ApiException
* if fails to make API call
*/
public CAPTCHAInstance updateCaptchaInstance(String captchaId, CAPTCHAInstance instance,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = instance;
// verify the required parameter 'captchaId' is set
if (captchaId == null) {
throw new ApiException(400,
"Missing the required parameter 'captchaId' when calling updateCaptchaInstance");
}
// verify the required parameter 'instance' is set
if (instance == null) {
throw new ApiException(400, "Missing the required parameter 'instance' when calling updateCaptchaInstance");
}
// create path and map variables
String localVarPath = "/api/v1/captchas/{captchaId}".replaceAll("\\{" + "captchaId" + "\\}",
apiClient.escapeString(captchaId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy