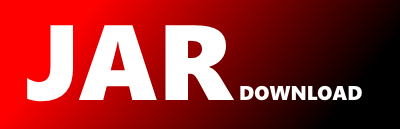
com.okta.sdk.resource.api.CustomPagesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.ErrorPage;
import com.okta.sdk.resource.model.HostedPage;
import com.okta.sdk.resource.model.PageRoot;
import com.okta.sdk.resource.model.SignInPage;
import java.net.URI;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class CustomPagesApi {
private ApiClient apiClient;
public CustomPagesApi() {
this(Configuration.getDefaultApiClient());
}
public CustomPagesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Delete the Customized Error Page Deletes the customized error page. As a result, the default error page appears
* in your live environment.
*
* @param brandId
* The ID of the brand (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deleteCustomizedErrorPage(String brandId) throws ApiException {
this.deleteCustomizedErrorPage(brandId, Collections.emptyMap());
}
/**
* Delete the Customized Error Page Deletes the customized error page. As a result, the default error page appears
* in your live environment.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deleteCustomizedErrorPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling deleteCustomizedErrorPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/error/customized".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete the Customized Sign-in Page Deletes the customized sign-in page. As a result, the default sign-in page
* appears in your live environment.
*
* @param brandId
* The ID of the brand (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deleteCustomizedSignInPage(String brandId) throws ApiException {
this.deleteCustomizedSignInPage(brandId, Collections.emptyMap());
}
/**
* Delete the Customized Sign-in Page Deletes the customized sign-in page. As a result, the default sign-in page
* appears in your live environment.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deleteCustomizedSignInPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling deleteCustomizedSignInPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in/customized".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete the Preview Error Page Deletes the preview error page. The preview error page contains unpublished changes
* and isn't shown in your live environment. Preview it at `${yourOktaDomain}/error/preview`.
*
* @param brandId
* The ID of the brand (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deletePreviewErrorPage(String brandId) throws ApiException {
this.deletePreviewErrorPage(brandId, Collections.emptyMap());
}
/**
* Delete the Preview Error Page Deletes the preview error page. The preview error page contains unpublished changes
* and isn't shown in your live environment. Preview it at `${yourOktaDomain}/error/preview`.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deletePreviewErrorPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling deletePreviewErrorPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/error/preview".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete the Preview Sign-in Page Deletes the preview sign-in page. The preview sign-in page contains unpublished
* changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/login/preview`.
*
* @param brandId
* The ID of the brand (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deletePreviewSignInPage(String brandId) throws ApiException {
this.deletePreviewSignInPage(brandId, Collections.emptyMap());
}
/**
* Delete the Preview Sign-in Page Deletes the preview sign-in page. The preview sign-in page contains unpublished
* changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/login/preview`.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deletePreviewSignInPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling deletePreviewSignInPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in/preview".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Retrieve the Customized Error Page Retrieves the customized error page. The customized error page appears in your
* live environment.
*
* @param brandId
* The ID of the brand (required)
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage getCustomizedErrorPage(String brandId) throws ApiException {
return this.getCustomizedErrorPage(brandId, Collections.emptyMap());
}
/**
* Retrieve the Customized Error Page Retrieves the customized error page. The customized error page appears in your
* live environment.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage getCustomizedErrorPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getCustomizedErrorPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/error/customized".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Customized Sign-in Page Retrieves the customized sign-in page. The customized sign-in page appears
* in your live environment.
*
* @param brandId
* The ID of the brand (required)
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage getCustomizedSignInPage(String brandId) throws ApiException {
return this.getCustomizedSignInPage(brandId, Collections.emptyMap());
}
/**
* Retrieve the Customized Sign-in Page Retrieves the customized sign-in page. The customized sign-in page appears
* in your live environment.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage getCustomizedSignInPage(String brandId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling getCustomizedSignInPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in/customized".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Default Error Page Retrieves the default error page. The default error page appears when no
* customized error page exists.
*
* @param brandId
* The ID of the brand (required)
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage getDefaultErrorPage(String brandId) throws ApiException {
return this.getDefaultErrorPage(brandId, Collections.emptyMap());
}
/**
* Retrieve the Default Error Page Retrieves the default error page. The default error page appears when no
* customized error page exists.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage getDefaultErrorPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getDefaultErrorPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/error/default".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Default Sign-in Page Retrieves the default sign-in page. The default sign-in page appears when no
* customized sign-in page exists.
*
* @param brandId
* The ID of the brand (required)
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage getDefaultSignInPage(String brandId) throws ApiException {
return this.getDefaultSignInPage(brandId, Collections.emptyMap());
}
/**
* Retrieve the Default Sign-in Page Retrieves the default sign-in page. The default sign-in page appears when no
* customized sign-in page exists.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage getDefaultSignInPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getDefaultSignInPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in/default".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Error Page Sub-Resources Retrieves the error page sub-resources. The `expand` query
* parameter specifies which sub-resources to include in the response.
*
* @param brandId
* The ID of the brand (required)
* @param expand
* Specifies additional metadata to be included in the response (optional
*
* @return PageRoot
*
* @throws ApiException
* if fails to make API call
*/
public PageRoot getErrorPage(String brandId, List expand) throws ApiException {
return this.getErrorPage(brandId, expand, Collections.emptyMap());
}
/**
* Retrieve the Error Page Sub-Resources Retrieves the error page sub-resources. The `expand` query
* parameter specifies which sub-resources to include in the response.
*
* @param brandId
* The ID of the brand (required)
* @param expand
* Specifies additional metadata to be included in the response (optional
* @param additionalHeaders
* additionalHeaders for this call
*
* @return PageRoot
*
* @throws ApiException
* if fails to make API call
*/
public PageRoot getErrorPage(String brandId, List expand, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getErrorPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/error".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("csv", "expand", expand));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Preview Error Page Preview Retrieves the preview error page. The preview error page contains
* unpublished changes and isn't shown in your live environment. Preview it at
* `${yourOktaDomain}/error/preview`.
*
* @param brandId
* The ID of the brand (required)
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage getPreviewErrorPage(String brandId) throws ApiException {
return this.getPreviewErrorPage(brandId, Collections.emptyMap());
}
/**
* Retrieve the Preview Error Page Preview Retrieves the preview error page. The preview error page contains
* unpublished changes and isn't shown in your live environment. Preview it at
* `${yourOktaDomain}/error/preview`.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage getPreviewErrorPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getPreviewErrorPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/error/preview".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Preview Sign-in Page Preview Retrieves the preview sign-in page. The preview sign-in page contains
* unpublished changes and isn't shown in your live environment. Preview it at
* `${yourOktaDomain}/login/preview`.
*
* @param brandId
* The ID of the brand (required)
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage getPreviewSignInPage(String brandId) throws ApiException {
return this.getPreviewSignInPage(brandId, Collections.emptyMap());
}
/**
* Retrieve the Preview Sign-in Page Preview Retrieves the preview sign-in page. The preview sign-in page contains
* unpublished changes and isn't shown in your live environment. Preview it at
* `${yourOktaDomain}/login/preview`.
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage getPreviewSignInPage(String brandId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getPreviewSignInPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in/preview".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Sign-in Page Sub-Resources Retrieves the sign-in page sub-resources. The `expand` query
* parameter specifies which sub-resources to include in the response.
*
* @param brandId
* The ID of the brand (required)
* @param expand
* Specifies additional metadata to be included in the response (optional
*
* @return PageRoot
*
* @throws ApiException
* if fails to make API call
*/
public PageRoot getSignInPage(String brandId, List expand) throws ApiException {
return this.getSignInPage(brandId, expand, Collections.emptyMap());
}
/**
* Retrieve the Sign-in Page Sub-Resources Retrieves the sign-in page sub-resources. The `expand` query
* parameter specifies which sub-resources to include in the response.
*
* @param brandId
* The ID of the brand (required)
* @param expand
* Specifies additional metadata to be included in the response (optional
* @param additionalHeaders
* additionalHeaders for this call
*
* @return PageRoot
*
* @throws ApiException
* if fails to make API call
*/
public PageRoot getSignInPage(String brandId, List expand, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getSignInPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("csv", "expand", expand));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Sign-out Page Settings Retrieves the sign-out page settings
*
* @param brandId
* The ID of the brand (required)
*
* @return HostedPage
*
* @throws ApiException
* if fails to make API call
*/
public HostedPage getSignOutPageSettings(String brandId) throws ApiException {
return this.getSignOutPageSettings(brandId, Collections.emptyMap());
}
/**
* Retrieve the Sign-out Page Settings Retrieves the sign-out page settings
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return HostedPage
*
* @throws ApiException
* if fails to make API call
*/
public HostedPage getSignOutPageSettings(String brandId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getSignOutPageSettings");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-out/customized".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Sign-in Widget Versions Lists all sign-in widget versions supported by the current org
*
* @param brandId
* The ID of the brand (required)
*
* @return List<String>
*
* @throws ApiException
* if fails to make API call
*/
public List listAllSignInWidgetVersions(String brandId) throws ApiException {
return this.listAllSignInWidgetVersions(brandId, Collections.emptyMap());
}
/**
* List all Sign-in Widget Versions Lists all sign-in widget versions supported by the current org
*
* @param brandId
* The ID of the brand (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<String>
*
* @throws ApiException
* if fails to make API call
*/
public List listAllSignInWidgetVersions(String brandId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling listAllSignInWidgetVersions");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in/widget-versions"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace the Customized Error Page Replaces the customized error page. The customized error page appears in your
* live environment.
*
* @param brandId
* The ID of the brand (required)
* @param errorPage
* (required)
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage replaceCustomizedErrorPage(String brandId, ErrorPage errorPage) throws ApiException {
return this.replaceCustomizedErrorPage(brandId, errorPage, Collections.emptyMap());
}
/**
* Replace the Customized Error Page Replaces the customized error page. The customized error page appears in your
* live environment.
*
* @param brandId
* The ID of the brand (required)
* @param errorPage
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage replaceCustomizedErrorPage(String brandId, ErrorPage errorPage,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = errorPage;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling replaceCustomizedErrorPage");
}
// verify the required parameter 'errorPage' is set
if (errorPage == null) {
throw new ApiException(400,
"Missing the required parameter 'errorPage' when calling replaceCustomizedErrorPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/error/customized".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace the Customized Sign-in Page Replaces the customized sign-in page. The customized sign-in page appears in
* your live environment.
*
* @param brandId
* The ID of the brand (required)
* @param signInPage
* (required)
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage replaceCustomizedSignInPage(String brandId, SignInPage signInPage) throws ApiException {
return this.replaceCustomizedSignInPage(brandId, signInPage, Collections.emptyMap());
}
/**
* Replace the Customized Sign-in Page Replaces the customized sign-in page. The customized sign-in page appears in
* your live environment.
*
* @param brandId
* The ID of the brand (required)
* @param signInPage
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage replaceCustomizedSignInPage(String brandId, SignInPage signInPage,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = signInPage;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling replaceCustomizedSignInPage");
}
// verify the required parameter 'signInPage' is set
if (signInPage == null) {
throw new ApiException(400,
"Missing the required parameter 'signInPage' when calling replaceCustomizedSignInPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in/customized".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace the Preview Error Page Replaces the preview error page. The preview error page contains unpublished
* changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/error/preview`.
*
* @param brandId
* The ID of the brand (required)
* @param errorPage
* (required)
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage replacePreviewErrorPage(String brandId, ErrorPage errorPage) throws ApiException {
return this.replacePreviewErrorPage(brandId, errorPage, Collections.emptyMap());
}
/**
* Replace the Preview Error Page Replaces the preview error page. The preview error page contains unpublished
* changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/error/preview`.
*
* @param brandId
* The ID of the brand (required)
* @param errorPage
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return ErrorPage
*
* @throws ApiException
* if fails to make API call
*/
public ErrorPage replacePreviewErrorPage(String brandId, ErrorPage errorPage, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = errorPage;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling replacePreviewErrorPage");
}
// verify the required parameter 'errorPage' is set
if (errorPage == null) {
throw new ApiException(400,
"Missing the required parameter 'errorPage' when calling replacePreviewErrorPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/error/preview".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace the Preview Sign-in Page Replaces the preview sign-in page. The preview sign-in page contains unpublished
* changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/login/preview`.
*
* @param brandId
* The ID of the brand (required)
* @param signInPage
* (required)
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage replacePreviewSignInPage(String brandId, SignInPage signInPage) throws ApiException {
return this.replacePreviewSignInPage(brandId, signInPage, Collections.emptyMap());
}
/**
* Replace the Preview Sign-in Page Replaces the preview sign-in page. The preview sign-in page contains unpublished
* changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/login/preview`.
*
* @param brandId
* The ID of the brand (required)
* @param signInPage
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return SignInPage
*
* @throws ApiException
* if fails to make API call
*/
public SignInPage replacePreviewSignInPage(String brandId, SignInPage signInPage,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = signInPage;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling replacePreviewSignInPage");
}
// verify the required parameter 'signInPage' is set
if (signInPage == null) {
throw new ApiException(400,
"Missing the required parameter 'signInPage' when calling replacePreviewSignInPage");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-in/preview".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace the Sign-out Page Settings Replaces the sign-out page settings
*
* @param brandId
* The ID of the brand (required)
* @param hostedPage
* (required)
*
* @return HostedPage
*
* @throws ApiException
* if fails to make API call
*/
public HostedPage replaceSignOutPageSettings(String brandId, HostedPage hostedPage) throws ApiException {
return this.replaceSignOutPageSettings(brandId, hostedPage, Collections.emptyMap());
}
/**
* Replace the Sign-out Page Settings Replaces the sign-out page settings
*
* @param brandId
* The ID of the brand (required)
* @param hostedPage
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return HostedPage
*
* @throws ApiException
* if fails to make API call
*/
public HostedPage replaceSignOutPageSettings(String brandId, HostedPage hostedPage,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = hostedPage;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling replaceSignOutPageSettings");
}
// verify the required parameter 'hostedPage' is set
if (hostedPage == null) {
throw new ApiException(400,
"Missing the required parameter 'hostedPage' when calling replaceSignOutPageSettings");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/pages/sign-out/customized".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy