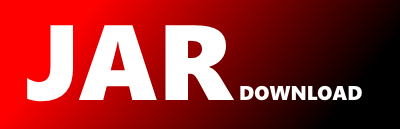
com.okta.sdk.resource.api.CustomTemplatesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.EmailCustomization;
import com.okta.sdk.resource.model.EmailDefaultContent;
import com.okta.sdk.resource.model.EmailPreview;
import com.okta.sdk.resource.model.EmailSettings;
import com.okta.sdk.resource.model.EmailSettingsResponse;
import com.okta.sdk.resource.model.EmailTemplateResponse;
import com.okta.sdk.resource.model.Error;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class CustomTemplatesApi {
private ApiClient apiClient;
public CustomTemplatesApi() {
this(Configuration.getDefaultApiClient());
}
public CustomTemplatesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Create an Email Customization Creates a new Email Customization <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is enabled, you
* can create a customization for any BCP47 language in addition to the Okta-supported languages.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param instance
* (optional)
*
* @return EmailCustomization
*
* @throws ApiException
* if fails to make API call
*/
public EmailCustomization createEmailCustomization(String brandId, String templateName, EmailCustomization instance)
throws ApiException {
return this.createEmailCustomization(brandId, templateName, instance, Collections.emptyMap());
}
/**
* Create an Email Customization Creates a new Email Customization <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is enabled, you
* can create a customization for any BCP47 language in addition to the Okta-supported languages.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param instance
* (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailCustomization
*
* @throws ApiException
* if fails to make API call
*/
public EmailCustomization createEmailCustomization(String brandId, String templateName, EmailCustomization instance,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = instance;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling createEmailCustomization");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling createEmailCustomization");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/customizations"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Delete all Email Customizations Deletes all customizations for an email template <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is enabled, all
* customizations are deleted, including customizations for additional languages. If disabled, only customizations
* in Okta-supported languages are deleted.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deleteAllCustomizations(String brandId, String templateName) throws ApiException {
this.deleteAllCustomizations(brandId, templateName, Collections.emptyMap());
}
/**
* Delete all Email Customizations Deletes all customizations for an email template <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is enabled, all
* customizations are deleted, including customizations for additional languages. If disabled, only customizations
* in Okta-supported languages are deleted.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deleteAllCustomizations(String brandId, String templateName, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling deleteAllCustomizations");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling deleteAllCustomizations");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/customizations"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete an Email Customization Deletes an Email Customization by its unique identifier <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is disabled,
* deletion of an existing additional language customization by ID doesn't register.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param customizationId
* The ID of the email customization (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deleteEmailCustomization(String brandId, String templateName, String customizationId)
throws ApiException {
this.deleteEmailCustomization(brandId, templateName, customizationId, Collections.emptyMap());
}
/**
* Delete an Email Customization Deletes an Email Customization by its unique identifier <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is disabled,
* deletion of an existing additional language customization by ID doesn't register.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param customizationId
* The ID of the email customization (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deleteEmailCustomization(String brandId, String templateName, String customizationId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling deleteEmailCustomization");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling deleteEmailCustomization");
}
// verify the required parameter 'customizationId' is set
if (customizationId == null) {
throw new ApiException(400,
"Missing the required parameter 'customizationId' when calling deleteEmailCustomization");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/customizations/{customizationId}"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()))
.replaceAll("\\{" + "customizationId" + "\\}", apiClient.escapeString(customizationId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Retrieve a Preview of an Email Customization Retrieves a Preview of an Email Customization. All variable
* references are populated from the current user's context. For example, `${user.profile.firstName}`.
* <x-lifecycle class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates
* is disabled, requests for the preview of an additional language customization by ID return a `404 Not
* Found` error response.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param customizationId
* The ID of the email customization (required)
*
* @return EmailPreview
*
* @throws ApiException
* if fails to make API call
*/
public EmailPreview getCustomizationPreview(String brandId, String templateName, String customizationId)
throws ApiException {
return this.getCustomizationPreview(brandId, templateName, customizationId, Collections.emptyMap());
}
/**
* Retrieve a Preview of an Email Customization Retrieves a Preview of an Email Customization. All variable
* references are populated from the current user's context. For example, `${user.profile.firstName}`.
* <x-lifecycle class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates
* is disabled, requests for the preview of an additional language customization by ID return a `404 Not
* Found` error response.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param customizationId
* The ID of the email customization (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailPreview
*
* @throws ApiException
* if fails to make API call
*/
public EmailPreview getCustomizationPreview(String brandId, String templateName, String customizationId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling getCustomizationPreview");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling getCustomizationPreview");
}
// verify the required parameter 'customizationId' is set
if (customizationId == null) {
throw new ApiException(400,
"Missing the required parameter 'customizationId' when calling getCustomizationPreview");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/customizations/{customizationId}/preview"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()))
.replaceAll("\\{" + "customizationId" + "\\}", apiClient.escapeString(customizationId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve an Email Customization Retrieves an email customization by its unique identifier <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is disabled,
* requests to retrieve an additional language customization by ID result in a `404 Not Found` error
* response.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param customizationId
* The ID of the email customization (required)
*
* @return EmailCustomization
*
* @throws ApiException
* if fails to make API call
*/
public EmailCustomization getEmailCustomization(String brandId, String templateName, String customizationId)
throws ApiException {
return this.getEmailCustomization(brandId, templateName, customizationId, Collections.emptyMap());
}
/**
* Retrieve an Email Customization Retrieves an email customization by its unique identifier <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is disabled,
* requests to retrieve an additional language customization by ID result in a `404 Not Found` error
* response.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param customizationId
* The ID of the email customization (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailCustomization
*
* @throws ApiException
* if fails to make API call
*/
public EmailCustomization getEmailCustomization(String brandId, String templateName, String customizationId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getEmailCustomization");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling getEmailCustomization");
}
// verify the required parameter 'customizationId' is set
if (customizationId == null) {
throw new ApiException(400,
"Missing the required parameter 'customizationId' when calling getEmailCustomization");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/customizations/{customizationId}"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()))
.replaceAll("\\{" + "customizationId" + "\\}", apiClient.escapeString(customizationId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve an Email Template Default Content Retrieves an email template's default content <x-lifecycle
* class=\"ea\"></x-lifecycle> Defaults to the current user's language given the
* following: - Custom languages for Okta Email Templates is enabled - An additional language is specified for the
* `language` parameter
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param language
* The language to use for the email. Defaults to the current user's language if unspecified.
* (optional)
*
* @return EmailDefaultContent
*
* @throws ApiException
* if fails to make API call
*/
public EmailDefaultContent getEmailDefaultContent(String brandId, String templateName, String language)
throws ApiException {
return this.getEmailDefaultContent(brandId, templateName, language, Collections.emptyMap());
}
/**
* Retrieve an Email Template Default Content Retrieves an email template's default content <x-lifecycle
* class=\"ea\"></x-lifecycle> Defaults to the current user's language given the
* following: - Custom languages for Okta Email Templates is enabled - An additional language is specified for the
* `language` parameter
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param language
* The language to use for the email. Defaults to the current user's language if unspecified.
* (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailDefaultContent
*
* @throws ApiException
* if fails to make API call
*/
public EmailDefaultContent getEmailDefaultContent(String brandId, String templateName, String language,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getEmailDefaultContent");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling getEmailDefaultContent");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/default-content"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("language", language));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve a Preview of the Email Template default content Retrieves a preview of an Email Template's default
* content. All variable references are populated using the current user's context. For example,
* `${user.profile.firstName}`. <x-lifecycle class=\"ea\"></x-lifecycle>
* Defaults to the current user's language given the following: - Custom languages for Okta Email Templates is
* enabled - An additional language is specified for the `language` parameter
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param language
* The language to use for the email. Defaults to the current user's language if unspecified.
* (optional)
*
* @return EmailPreview
*
* @throws ApiException
* if fails to make API call
*/
public EmailPreview getEmailDefaultPreview(String brandId, String templateName, String language)
throws ApiException {
return this.getEmailDefaultPreview(brandId, templateName, language, Collections.emptyMap());
}
/**
* Retrieve a Preview of the Email Template default content Retrieves a preview of an Email Template's default
* content. All variable references are populated using the current user's context. For example,
* `${user.profile.firstName}`. <x-lifecycle class=\"ea\"></x-lifecycle>
* Defaults to the current user's language given the following: - Custom languages for Okta Email Templates is
* enabled - An additional language is specified for the `language` parameter
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param language
* The language to use for the email. Defaults to the current user's language if unspecified.
* (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailPreview
*
* @throws ApiException
* if fails to make API call
*/
public EmailPreview getEmailDefaultPreview(String brandId, String templateName, String language,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getEmailDefaultPreview");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling getEmailDefaultPreview");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/default-content/preview"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("language", language));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the Email Template Settings Retrieves an email template's settings
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
*
* @return EmailSettingsResponse
*
* @throws ApiException
* if fails to make API call
*/
public EmailSettingsResponse getEmailSettings(String brandId, String templateName) throws ApiException {
return this.getEmailSettings(brandId, templateName, Collections.emptyMap());
}
/**
* Retrieve the Email Template Settings Retrieves an email template's settings
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailSettingsResponse
*
* @throws ApiException
* if fails to make API call
*/
public EmailSettingsResponse getEmailSettings(String brandId, String templateName,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getEmailSettings");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400, "Missing the required parameter 'templateName' when calling getEmailSettings");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/settings"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve an Email Template Retrieves the details of an email template by name
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param expand
* Specifies additional metadata to be included in the response (optional
*
* @return EmailTemplateResponse
*
* @throws ApiException
* if fails to make API call
*/
public EmailTemplateResponse getEmailTemplate(String brandId, String templateName, List expand)
throws ApiException {
return this.getEmailTemplate(brandId, templateName, expand, Collections.emptyMap());
}
/**
* Retrieve an Email Template Retrieves the details of an email template by name
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param expand
* Specifies additional metadata to be included in the response (optional
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailTemplateResponse
*
* @throws ApiException
* if fails to make API call
*/
public EmailTemplateResponse getEmailTemplate(String brandId, String templateName, List expand,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling getEmailTemplate");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400, "Missing the required parameter 'templateName' when calling getEmailTemplate");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("csv", "expand", expand));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Email Customizations Lists all customizations of an email template <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is enabled, all
* existing customizations are retrieved, including customizations for additional languages. If disabled, only
* customizations for Okta-supported languages are returned.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param after
* The cursor to use for pagination. It is an opaque string that specifies your current location in the
* list and is obtained from the `Link` response header. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param limit
* A limit on the number of objects to return (optional, default to 20)
*
* @return List<EmailCustomization>
*
* @throws ApiException
* if fails to make API call
*/
public List listEmailCustomizations(String brandId, String templateName, String after,
Integer limit) throws ApiException {
return this.listEmailCustomizations(brandId, templateName, after, limit, Collections.emptyMap());
}
/**
* List all Email Customizations Lists all customizations of an email template <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is enabled, all
* existing customizations are retrieved, including customizations for additional languages. If disabled, only
* customizations for Okta-supported languages are returned.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param after
* The cursor to use for pagination. It is an opaque string that specifies your current location in the
* list and is obtained from the `Link` response header. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param limit
* A limit on the number of objects to return (optional, default to 20)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<EmailCustomization>
*
* @throws ApiException
* if fails to make API call
*/
public List listEmailCustomizations(String brandId, String templateName, String after,
Integer limit, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling listEmailCustomizations");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling listEmailCustomizations");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/customizations"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Email Templates Lists all supported email templates
*
* @param brandId
* The ID of the brand (required)
* @param after
* The cursor to use for pagination. It is an opaque string that specifies your current location in the
* list and is obtained from the `Link` response header. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param limit
* A limit on the number of objects to return (optional, default to 20)
* @param expand
* Specifies additional metadata to be included in the response (optional
*
* @return List<EmailTemplateResponse>
*
* @throws ApiException
* if fails to make API call
*/
public List listEmailTemplates(String brandId, String after, Integer limit,
List expand) throws ApiException {
return this.listEmailTemplates(brandId, after, limit, expand, Collections.emptyMap());
}
/**
* List all Email Templates Lists all supported email templates
*
* @param brandId
* The ID of the brand (required)
* @param after
* The cursor to use for pagination. It is an opaque string that specifies your current location in the
* list and is obtained from the `Link` response header. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param limit
* A limit on the number of objects to return (optional, default to 20)
* @param expand
* Specifies additional metadata to be included in the response (optional
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<EmailTemplateResponse>
*
* @throws ApiException
* if fails to make API call
*/
public List listEmailTemplates(String brandId, String after, Integer limit,
List expand, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling listEmailTemplates");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email".replaceAll("\\{" + "brandId" + "\\}",
apiClient.escapeString(brandId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("csv", "expand", expand));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace an Email Customization Replaces an email customization using property values <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is disabled,
* requests to update a customization for an additional language return a `404 Not Found` error response.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param customizationId
* The ID of the email customization (required)
* @param instance
* Request (optional)
*
* @return EmailCustomization
*
* @throws ApiException
* if fails to make API call
*/
public EmailCustomization replaceEmailCustomization(String brandId, String templateName, String customizationId,
EmailCustomization instance) throws ApiException {
return this.replaceEmailCustomization(brandId, templateName, customizationId, instance, Collections.emptyMap());
}
/**
* Replace an Email Customization Replaces an email customization using property values <x-lifecycle
* class=\"ea\"></x-lifecycle> If Custom languages for Okta Email Templates is disabled,
* requests to update a customization for an additional language return a `404 Not Found` error response.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param customizationId
* The ID of the email customization (required)
* @param instance
* Request (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailCustomization
*
* @throws ApiException
* if fails to make API call
*/
public EmailCustomization replaceEmailCustomization(String brandId, String templateName, String customizationId,
EmailCustomization instance, Map additionalHeaders) throws ApiException {
Object localVarPostBody = instance;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400,
"Missing the required parameter 'brandId' when calling replaceEmailCustomization");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling replaceEmailCustomization");
}
// verify the required parameter 'customizationId' is set
if (customizationId == null) {
throw new ApiException(400,
"Missing the required parameter 'customizationId' when calling replaceEmailCustomization");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/customizations/{customizationId}"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()))
.replaceAll("\\{" + "customizationId" + "\\}", apiClient.escapeString(customizationId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace the Email Template Settings Replaces an email template's settings
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param emailSettings
* (optional)
*
* @return EmailSettings
*
* @throws ApiException
* if fails to make API call
*/
public EmailSettings replaceEmailSettings(String brandId, String templateName, EmailSettings emailSettings)
throws ApiException {
return this.replaceEmailSettings(brandId, templateName, emailSettings, Collections.emptyMap());
}
/**
* Replace the Email Template Settings Replaces an email template's settings
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param emailSettings
* (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return EmailSettings
*
* @throws ApiException
* if fails to make API call
*/
public EmailSettings replaceEmailSettings(String brandId, String templateName, EmailSettings emailSettings,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = emailSettings;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling replaceEmailSettings");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400,
"Missing the required parameter 'templateName' when calling replaceEmailSettings");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/settings"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Send a Test Email Sends a test email to the current user’s primary and secondary email addresses. The email
* content is selected based on the following priority: 1. The email customization for the language specified in the
* `language` query parameter. <x-lifecycle class=\"ea\"></x-lifecycle> If
* Custom languages for Okta Email Templates is enabled and the `language` parameter is an additional
* language, the test email uses the customization corresponding to the language. 2. The email template's
* default customization. 3. The email template’s default content, translated to the current user's language.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param language
* The language to use for the email. Defaults to the current user's language if unspecified.
* (optional)
*
* @throws ApiException
* if fails to make API call
*/
public void sendTestEmail(String brandId, String templateName, String language) throws ApiException {
this.sendTestEmail(brandId, templateName, language, Collections.emptyMap());
}
/**
* Send a Test Email Sends a test email to the current user’s primary and secondary email addresses. The email
* content is selected based on the following priority: 1. The email customization for the language specified in the
* `language` query parameter. <x-lifecycle class=\"ea\"></x-lifecycle> If
* Custom languages for Okta Email Templates is enabled and the `language` parameter is an additional
* language, the test email uses the customization corresponding to the language. 2. The email template's
* default customization. 3. The email template’s default content, translated to the current user's language.
*
* @param brandId
* The ID of the brand (required)
* @param templateName
* The name of the email template (required)
* @param language
* The language to use for the email. Defaults to the current user's language if unspecified.
* (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void sendTestEmail(String brandId, String templateName, String language,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'brandId' is set
if (brandId == null) {
throw new ApiException(400, "Missing the required parameter 'brandId' when calling sendTestEmail");
}
// verify the required parameter 'templateName' is set
if (templateName == null) {
throw new ApiException(400, "Missing the required parameter 'templateName' when calling sendTestEmail");
}
// create path and map variables
String localVarPath = "/api/v1/brands/{brandId}/templates/email/{templateName}/test"
.replaceAll("\\{" + "brandId" + "\\}", apiClient.escapeString(brandId.toString()))
.replaceAll("\\{" + "templateName" + "\\}", apiClient.escapeString(templateName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("language", language));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy