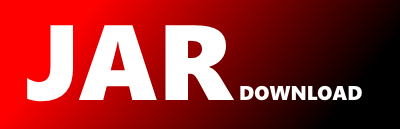
com.okta.sdk.resource.api.PolicyApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.Application;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.Policy;
import com.okta.sdk.resource.model.PolicyMapping;
import com.okta.sdk.resource.model.PolicyMappingRequest;
import com.okta.sdk.resource.model.PolicyRule;
import com.okta.sdk.resource.model.SimulatePolicyBody;
import com.okta.sdk.resource.model.SimulatePolicyEvaluations;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class PolicyApi {
private ApiClient apiClient;
public PolicyApi() {
this(Configuration.getDefaultApiClient());
}
public PolicyApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Activate a Policy Activates a policy
*
* @param policyId
* `id` of the Policy (required)
*
* @throws ApiException
* if fails to make API call
*/
public void activatePolicy(String policyId) throws ApiException {
this.activatePolicy(policyId, Collections.emptyMap());
}
/**
* Activate a Policy Activates a policy
*
* @param policyId
* `id` of the Policy (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void activatePolicy(String policyId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling activatePolicy");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/lifecycle/activate".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Activate a Policy Rule Activates a Policy Rule identified by `policyId` and `ruleId`
*
* @param policyId
* `id` of the Policy (required)
* @param ruleId
* `id` of the Policy Rule (required)
*
* @throws ApiException
* if fails to make API call
*/
public void activatePolicyRule(String policyId, String ruleId) throws ApiException {
this.activatePolicyRule(policyId, ruleId, Collections.emptyMap());
}
/**
* Activate a Policy Rule Activates a Policy Rule identified by `policyId` and `ruleId`
*
* @param policyId
* `id` of the Policy (required)
* @param ruleId
* `id` of the Policy Rule (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void activatePolicyRule(String policyId, String ruleId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling activatePolicyRule");
}
// verify the required parameter 'ruleId' is set
if (ruleId == null) {
throw new ApiException(400, "Missing the required parameter 'ruleId' when calling activatePolicyRule");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/rules/{ruleId}/lifecycle/activate"
.replaceAll("\\{" + "policyId" + "\\}", apiClient.escapeString(policyId.toString()))
.replaceAll("\\{" + "ruleId" + "\\}", apiClient.escapeString(ruleId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Clone an existing Policy Clones an existing policy
*
* @param policyId
* `id` of the Policy (required)
*
* @return Policy
*
* @throws ApiException
* if fails to make API call
*/
public Policy clonePolicy(String policyId) throws ApiException {
return this.clonePolicy(policyId, Collections.emptyMap());
}
/**
* Clone an existing Policy Clones an existing policy
*
* @param policyId
* `id` of the Policy (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return Policy
*
* @throws ApiException
* if fails to make API call
*/
public Policy clonePolicy(String policyId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling clonePolicy");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/clone".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a Policy Creates a policy. There are many types of policies that you can create. See
* [Policies](https://developer.okta.com/docs/concepts/policies/) for an overview of the types of policies available
* and then links to more indepth information.
*
* @param policy
* (required)
* @param activate
* This query parameter is only valid for Classic Engine orgs. (optional, default to true)
*
* @return Policy
*
* @throws ApiException
* if fails to make API call
*/
public Policy createPolicy(Policy policy, Boolean activate) throws ApiException {
return this.createPolicy(policy, activate, Collections.emptyMap());
}
/**
* Create a Policy Creates a policy. There are many types of policies that you can create. See
* [Policies](https://developer.okta.com/docs/concepts/policies/) for an overview of the types of policies available
* and then links to more indepth information.
*
* @param policy
* (required)
* @param activate
* This query parameter is only valid for Classic Engine orgs. (optional, default to true)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return Policy
*
* @throws ApiException
* if fails to make API call
*/
public Policy createPolicy(Policy policy, Boolean activate, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = policy;
// verify the required parameter 'policy' is set
if (policy == null) {
throw new ApiException(400, "Missing the required parameter 'policy' when calling createPolicy");
}
// create path and map variables
String localVarPath = "/api/v1/policies";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("activate", activate));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a Policy Rule Creates a policy rule > **Note:** You can't create additional rules for the
* `PROFILE_ENROLLMENT` or `POST_AUTH_SESSION` policies.
*
* @param policyId
* `id` of the Policy (required)
* @param policyRule
* (required)
* @param limit
* Defines the number of policy rules returned. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param activate
* Set this parameter to `false` to create an `INACTIVE` rule. (optional, default to
* true)
*
* @return PolicyRule
*
* @throws ApiException
* if fails to make API call
*/
public PolicyRule createPolicyRule(String policyId, PolicyRule policyRule, String limit, Boolean activate)
throws ApiException {
return this.createPolicyRule(policyId, policyRule, limit, activate, Collections.emptyMap());
}
/**
* Create a Policy Rule Creates a policy rule > **Note:** You can't create additional rules for the
* `PROFILE_ENROLLMENT` or `POST_AUTH_SESSION` policies.
*
* @param policyId
* `id` of the Policy (required)
* @param policyRule
* (required)
* @param limit
* Defines the number of policy rules returned. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param activate
* Set this parameter to `false` to create an `INACTIVE` rule. (optional, default to
* true)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return PolicyRule
*
* @throws ApiException
* if fails to make API call
*/
public PolicyRule createPolicyRule(String policyId, PolicyRule policyRule, String limit, Boolean activate,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = policyRule;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling createPolicyRule");
}
// verify the required parameter 'policyRule' is set
if (policyRule == null) {
throw new ApiException(400, "Missing the required parameter 'policyRule' when calling createPolicyRule");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/rules".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPair("activate", activate));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a Policy Simulation Creates a policy or policy rule simulation. The access simulation evaluates policy and
* policy rules based on the existing policy rule configuration. The evaluation result simulates what the real-world
* authentication flow is and what policy rules have been applied or matched to the authentication flow.
*
* @param simulatePolicy
* (required)
* @param expand
* Use `expand=EVALUATED` to include a list of evaluated but not matched policies and
* policy rules. Use `expand=RULE` to include details about why a rule condition
* wasn't matched. (optional)
*
* @return List<SimulatePolicyEvaluations>
*
* @throws ApiException
* if fails to make API call
*/
public List createPolicySimulation(List simulatePolicy,
String expand) throws ApiException {
return this.createPolicySimulation(simulatePolicy, expand, Collections.emptyMap());
}
/**
* Create a Policy Simulation Creates a policy or policy rule simulation. The access simulation evaluates policy and
* policy rules based on the existing policy rule configuration. The evaluation result simulates what the real-world
* authentication flow is and what policy rules have been applied or matched to the authentication flow.
*
* @param simulatePolicy
* (required)
* @param expand
* Use `expand=EVALUATED` to include a list of evaluated but not matched policies and
* policy rules. Use `expand=RULE` to include details about why a rule condition
* wasn't matched. (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<SimulatePolicyEvaluations>
*
* @throws ApiException
* if fails to make API call
*/
public List createPolicySimulation(List simulatePolicy,
String expand, Map additionalHeaders) throws ApiException {
Object localVarPostBody = simulatePolicy;
// verify the required parameter 'simulatePolicy' is set
if (simulatePolicy == null) {
throw new ApiException(400,
"Missing the required parameter 'simulatePolicy' when calling createPolicySimulation");
}
// create path and map variables
String localVarPath = "/api/v1/policies/simulate";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("expand", expand));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Deactivate a Policy Deactivates a policy
*
* @param policyId
* `id` of the Policy (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deactivatePolicy(String policyId) throws ApiException {
this.deactivatePolicy(policyId, Collections.emptyMap());
}
/**
* Deactivate a Policy Deactivates a policy
*
* @param policyId
* `id` of the Policy (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deactivatePolicy(String policyId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling deactivatePolicy");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/lifecycle/deactivate".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Deactivate a Policy Rule Deactivates a Policy Rule identified by `policyId` and `ruleId`
*
* @param policyId
* `id` of the Policy (required)
* @param ruleId
* `id` of the Policy Rule (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deactivatePolicyRule(String policyId, String ruleId) throws ApiException {
this.deactivatePolicyRule(policyId, ruleId, Collections.emptyMap());
}
/**
* Deactivate a Policy Rule Deactivates a Policy Rule identified by `policyId` and `ruleId`
*
* @param policyId
* `id` of the Policy (required)
* @param ruleId
* `id` of the Policy Rule (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deactivatePolicyRule(String policyId, String ruleId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling deactivatePolicyRule");
}
// verify the required parameter 'ruleId' is set
if (ruleId == null) {
throw new ApiException(400, "Missing the required parameter 'ruleId' when calling deactivatePolicyRule");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/rules/{ruleId}/lifecycle/deactivate"
.replaceAll("\\{" + "policyId" + "\\}", apiClient.escapeString(policyId.toString()))
.replaceAll("\\{" + "ruleId" + "\\}", apiClient.escapeString(ruleId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete a Policy Deletes a policy
*
* @param policyId
* `id` of the Policy (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deletePolicy(String policyId) throws ApiException {
this.deletePolicy(policyId, Collections.emptyMap());
}
/**
* Delete a Policy Deletes a policy
*
* @param policyId
* `id` of the Policy (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deletePolicy(String policyId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling deletePolicy");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete a policy resource Mapping Deletes the resource Mapping for a Policy identified by `policyId` and
* `mappingId`
*
* @param policyId
* `id` of the Policy (required)
* @param mappingId
* `id` of the policy resource Mapping (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deletePolicyResourceMapping(String policyId, String mappingId) throws ApiException {
this.deletePolicyResourceMapping(policyId, mappingId, Collections.emptyMap());
}
/**
* Delete a policy resource Mapping Deletes the resource Mapping for a Policy identified by `policyId` and
* `mappingId`
*
* @param policyId
* `id` of the Policy (required)
* @param mappingId
* `id` of the policy resource Mapping (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deletePolicyResourceMapping(String policyId, String mappingId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400,
"Missing the required parameter 'policyId' when calling deletePolicyResourceMapping");
}
// verify the required parameter 'mappingId' is set
if (mappingId == null) {
throw new ApiException(400,
"Missing the required parameter 'mappingId' when calling deletePolicyResourceMapping");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/mappings/{mappingId}"
.replaceAll("\\{" + "policyId" + "\\}", apiClient.escapeString(policyId.toString()))
.replaceAll("\\{" + "mappingId" + "\\}", apiClient.escapeString(mappingId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete a Policy Rule Deletes a Policy Rule identified by `policyId` and `ruleId`
*
* @param policyId
* `id` of the Policy (required)
* @param ruleId
* `id` of the Policy Rule (required)
*
* @throws ApiException
* if fails to make API call
*/
public void deletePolicyRule(String policyId, String ruleId) throws ApiException {
this.deletePolicyRule(policyId, ruleId, Collections.emptyMap());
}
/**
* Delete a Policy Rule Deletes a Policy Rule identified by `policyId` and `ruleId`
*
* @param policyId
* `id` of the Policy (required)
* @param ruleId
* `id` of the Policy Rule (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deletePolicyRule(String policyId, String ruleId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling deletePolicyRule");
}
// verify the required parameter 'ruleId' is set
if (ruleId == null) {
throw new ApiException(400, "Missing the required parameter 'ruleId' when calling deletePolicyRule");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/rules/{ruleId}"
.replaceAll("\\{" + "policyId" + "\\}", apiClient.escapeString(policyId.toString()))
.replaceAll("\\{" + "ruleId" + "\\}", apiClient.escapeString(ruleId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Retrieve a Policy Retrieves a policy
*
* @param policyId
* `id` of the Policy (required)
* @param expand
* (optional, default to )
*
* @return Policy
*
* @throws ApiException
* if fails to make API call
*/
public Policy getPolicy(String policyId, String expand) throws ApiException {
return this.getPolicy(policyId, expand, Collections.emptyMap());
}
/**
* Retrieve a Policy Retrieves a policy
*
* @param policyId
* `id` of the Policy (required)
* @param expand
* (optional, default to )
* @param additionalHeaders
* additionalHeaders for this call
*
* @return Policy
*
* @throws ApiException
* if fails to make API call
*/
public Policy getPolicy(String policyId, String expand, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling getPolicy");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("expand", expand));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve a policy resource Mapping Retrieves a resource Mapping for a Policy identified by `policyId`
* and `mappingId`
*
* @param policyId
* `id` of the Policy (required)
* @param mappingId
* `id` of the policy resource Mapping (required)
*
* @return PolicyMapping
*
* @throws ApiException
* if fails to make API call
*/
public PolicyMapping getPolicyMapping(String policyId, String mappingId) throws ApiException {
return this.getPolicyMapping(policyId, mappingId, Collections.emptyMap());
}
/**
* Retrieve a policy resource Mapping Retrieves a resource Mapping for a Policy identified by `policyId`
* and `mappingId`
*
* @param policyId
* `id` of the Policy (required)
* @param mappingId
* `id` of the policy resource Mapping (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return PolicyMapping
*
* @throws ApiException
* if fails to make API call
*/
public PolicyMapping getPolicyMapping(String policyId, String mappingId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling getPolicyMapping");
}
// verify the required parameter 'mappingId' is set
if (mappingId == null) {
throw new ApiException(400, "Missing the required parameter 'mappingId' when calling getPolicyMapping");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/mappings/{mappingId}"
.replaceAll("\\{" + "policyId" + "\\}", apiClient.escapeString(policyId.toString()))
.replaceAll("\\{" + "mappingId" + "\\}", apiClient.escapeString(mappingId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve a Policy Rule Retrieves a policy rule
*
* @param policyId
* `id` of the Policy (required)
* @param ruleId
* `id` of the Policy Rule (required)
*
* @return PolicyRule
*
* @throws ApiException
* if fails to make API call
*/
public PolicyRule getPolicyRule(String policyId, String ruleId) throws ApiException {
return this.getPolicyRule(policyId, ruleId, Collections.emptyMap());
}
/**
* Retrieve a Policy Rule Retrieves a policy rule
*
* @param policyId
* `id` of the Policy (required)
* @param ruleId
* `id` of the Policy Rule (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return PolicyRule
*
* @throws ApiException
* if fails to make API call
*/
public PolicyRule getPolicyRule(String policyId, String ruleId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling getPolicyRule");
}
// verify the required parameter 'ruleId' is set
if (ruleId == null) {
throw new ApiException(400, "Missing the required parameter 'ruleId' when calling getPolicyRule");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/rules/{ruleId}"
.replaceAll("\\{" + "policyId" + "\\}", apiClient.escapeString(policyId.toString()))
.replaceAll("\\{" + "ruleId" + "\\}", apiClient.escapeString(ruleId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Policies Lists all policies with the specified type
*
* @param type
* Specifies the type of policy to return. The following policy types are available only with the Okta
* Identity Engine - `ACCESS_POLICY`, `PROFILE_ENROLLMENT`,
* `POST_AUTH_SESSION`, and `ENTITY_RISK`. The `POST_AUTH_SESSION` and
* `ENTITY_RISK` policy types are in <x-lifecycle
* class=\"ea\"></x-lifecycle>. Contact your Okta account team to enable these
* features. (required)
* @param status
* Refines the query by the `status` of the policy - `ACTIVE` or `INACTIVE`
* (optional)
* @param q
* Refines the query by policy name prefix (startWith method) passed in as `q=string`
* (optional)
* @param expand
* (optional, default to )
* @param sortBy
* Refines the query by sorting on the policy `name` in ascending order (optional)
* @param limit
* Defines the number of policies returned, see
* [Pagination](https://developer.okta.com/docs/api/#pagination) (optional)
* @param resourceId
* Reference to the associated authorization server (optional)
* @param after
* End page cursor for pagination, see [Pagination](https://developer.okta.com/docs/api/#pagination)
* (optional)
*
* @return List<Policy>
*
* @throws ApiException
* if fails to make API call
*/
public List listPolicies(String type, String status, String q, String expand, String sortBy, String limit,
String resourceId, String after) throws ApiException {
return this.listPolicies(type, status, q, expand, sortBy, limit, resourceId, after, Collections.emptyMap());
}
/**
* List all Policies Lists all policies with the specified type
*
* @param type
* Specifies the type of policy to return. The following policy types are available only with the Okta
* Identity Engine - `ACCESS_POLICY`, `PROFILE_ENROLLMENT`,
* `POST_AUTH_SESSION`, and `ENTITY_RISK`. The `POST_AUTH_SESSION` and
* `ENTITY_RISK` policy types are in <x-lifecycle
* class=\"ea\"></x-lifecycle>. Contact your Okta account team to enable these
* features. (required)
* @param status
* Refines the query by the `status` of the policy - `ACTIVE` or `INACTIVE`
* (optional)
* @param q
* Refines the query by policy name prefix (startWith method) passed in as `q=string`
* (optional)
* @param expand
* (optional, default to )
* @param sortBy
* Refines the query by sorting on the policy `name` in ascending order (optional)
* @param limit
* Defines the number of policies returned, see
* [Pagination](https://developer.okta.com/docs/api/#pagination) (optional)
* @param resourceId
* Reference to the associated authorization server (optional)
* @param after
* End page cursor for pagination, see [Pagination](https://developer.okta.com/docs/api/#pagination)
* (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<Policy>
*
* @throws ApiException
* if fails to make API call
*/
public List listPolicies(String type, String status, String q, String expand, String sortBy, String limit,
String resourceId, String after, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException(400, "Missing the required parameter 'type' when calling listPolicies");
}
// create path and map variables
String localVarPath = "/api/v1/policies";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("type", type));
localVarQueryParams.addAll(apiClient.parameterToPair("status", status));
localVarQueryParams.addAll(apiClient.parameterToPair("q", q));
localVarQueryParams.addAll(apiClient.parameterToPair("expand", expand));
localVarQueryParams.addAll(apiClient.parameterToPair("sortBy", sortBy));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPair("resourceId", resourceId));
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Applications mapped to a Policy Lists all applications mapped to a policy identified by
* `policyId` > **Note:** Use [List all resources mapped to a
* Policy](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/Policy/#tag/Policy/operation/listPolicyMappings)
* to list all applications mapped to a policy.
*
* @param policyId
* `id` of the Policy (required)
*
* @return List<Application>
*
* @throws ApiException
* if fails to make API call
*
* @deprecated
*/
@Deprecated
public List listPolicyApps(String policyId) throws ApiException {
return this.listPolicyApps(policyId, Collections.emptyMap());
}
/**
* List all Applications mapped to a Policy Lists all applications mapped to a policy identified by
* `policyId` > **Note:** Use [List all resources mapped to a
* Policy](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/Policy/#tag/Policy/operation/listPolicyMappings)
* to list all applications mapped to a policy.
*
* @param policyId
* `id` of the Policy (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<Application>
*
* @throws ApiException
* if fails to make API call
*
* @deprecated
*/
@Deprecated
public List listPolicyApps(String policyId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling listPolicyApps");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/app".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all resources mapped to a Policy Lists all resources mapped to a Policy identified by `policyId`
*
* @param policyId
* `id` of the Policy (required)
*
* @return List<PolicyMapping>
*
* @throws ApiException
* if fails to make API call
*/
public List listPolicyMappings(String policyId) throws ApiException {
return this.listPolicyMappings(policyId, Collections.emptyMap());
}
/**
* List all resources mapped to a Policy Lists all resources mapped to a Policy identified by `policyId`
*
* @param policyId
* `id` of the Policy (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<PolicyMapping>
*
* @throws ApiException
* if fails to make API call
*/
public List listPolicyMappings(String policyId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling listPolicyMappings");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/mappings".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Policy Rules Lists all policy rules
*
* @param policyId
* `id` of the Policy (required)
* @param limit
* Defines the number of policy rules returned. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
*
* @return List<PolicyRule>
*
* @throws ApiException
* if fails to make API call
*/
public List listPolicyRules(String policyId, String limit) throws ApiException {
return this.listPolicyRules(policyId, limit, Collections.emptyMap());
}
/**
* List all Policy Rules Lists all policy rules
*
* @param policyId
* `id` of the Policy (required)
* @param limit
* Defines the number of policy rules returned. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<PolicyRule>
*
* @throws ApiException
* if fails to make API call
*/
public List listPolicyRules(String policyId, String limit, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling listPolicyRules");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/rules".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Map a resource to a Policy Maps a resource to a Policy identified by `policyId`
*
* @param policyId
* `id` of the Policy (required)
* @param policyMappingRequest
* (required)
*
* @return PolicyMapping
*
* @throws ApiException
* if fails to make API call
*/
public PolicyMapping mapResourceToPolicy(String policyId, PolicyMappingRequest policyMappingRequest)
throws ApiException {
return this.mapResourceToPolicy(policyId, policyMappingRequest, Collections.emptyMap());
}
/**
* Map a resource to a Policy Maps a resource to a Policy identified by `policyId`
*
* @param policyId
* `id` of the Policy (required)
* @param policyMappingRequest
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return PolicyMapping
*
* @throws ApiException
* if fails to make API call
*/
public PolicyMapping mapResourceToPolicy(String policyId, PolicyMappingRequest policyMappingRequest,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = policyMappingRequest;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling mapResourceToPolicy");
}
// verify the required parameter 'policyMappingRequest' is set
if (policyMappingRequest == null) {
throw new ApiException(400,
"Missing the required parameter 'policyMappingRequest' when calling mapResourceToPolicy");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}/mappings".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace a Policy Replaces the properties of a Policy identified by `policyId`
*
* @param policyId
* `id` of the Policy (required)
* @param policy
* (required)
*
* @return Policy
*
* @throws ApiException
* if fails to make API call
*/
public Policy replacePolicy(String policyId, Policy policy) throws ApiException {
return this.replacePolicy(policyId, policy, Collections.emptyMap());
}
/**
* Replace a Policy Replaces the properties of a Policy identified by `policyId`
*
* @param policyId
* `id` of the Policy (required)
* @param policy
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return Policy
*
* @throws ApiException
* if fails to make API call
*/
public Policy replacePolicy(String policyId, Policy policy, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = policy;
// verify the required parameter 'policyId' is set
if (policyId == null) {
throw new ApiException(400, "Missing the required parameter 'policyId' when calling replacePolicy");
}
// verify the required parameter 'policy' is set
if (policy == null) {
throw new ApiException(400, "Missing the required parameter 'policy' when calling replacePolicy");
}
// create path and map variables
String localVarPath = "/api/v1/policies/{policyId}".replaceAll("\\{" + "policyId" + "\\}",
apiClient.escapeString(policyId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map