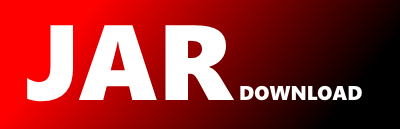
com.okta.sdk.resource.api.RiskEventApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.RiskEvent;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class RiskEventApi {
private ApiClient apiClient;
public RiskEventApi() {
this(Configuration.getDefaultApiClient());
}
public RiskEventApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Send multiple Risk Events Sends multiple IP risk events to Okta. This request is used by a third-party risk
* provider to send IP risk events to Okta. The third-party risk provider needs to be registered with Okta before
* they can send events to Okta. See [Risk Providers](/openapi/okta-management/management/tag/RiskProvider/). This
* API has a rate limit of 30 requests per minute. You can include multiple risk events (up to a maximum of 20
* events) in a single payload to reduce the number of API calls. Prioritize sending high risk signals if you have a
* burst of signals to send that would exceed the maximum request limits.
*
* @param instance
* (required)
*
* @throws ApiException
* if fails to make API call
*/
public void sendRiskEvents(List instance) throws ApiException {
this.sendRiskEvents(instance, Collections.emptyMap());
}
/**
* Send multiple Risk Events Sends multiple IP risk events to Okta. This request is used by a third-party risk
* provider to send IP risk events to Okta. The third-party risk provider needs to be registered with Okta before
* they can send events to Okta. See [Risk Providers](/openapi/okta-management/management/tag/RiskProvider/). This
* API has a rate limit of 30 requests per minute. You can include multiple risk events (up to a maximum of 20
* events) in a single payload to reduce the number of API calls. Prioritize sending high risk signals if you have a
* burst of signals to send that would exceed the maximum request limits.
*
* @param instance
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void sendRiskEvents(List instance, Map additionalHeaders) throws ApiException {
Object localVarPostBody = instance;
// verify the required parameter 'instance' is set
if (instance == null) {
throw new ApiException(400, "Missing the required parameter 'instance' when calling sendRiskEvents");
}
// create path and map variables
String localVarPath = "/api/v1/risk/events/ip";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy