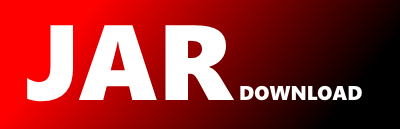
com.okta.sdk.resource.api.RoleBTargetBGroupApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.CatalogApplication;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.Group;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class RoleBTargetBGroupApi {
private ApiClient apiClient;
public RoleBTargetBGroupApi() {
this(Configuration.getDefaultApiClient());
}
public RoleBTargetBGroupApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Assign an Group Role Application Instance Target Assigns an app instance target to an `APP_ADMIN` Role
* Assignment to a Group. When you assign the first OIN app or app instance target, you reduce the scope of the Role
* Assignment. The Role no longer applies to all app targets, but applies only to the specified target. >
* **Note:** You can target a mixture of both OIN app and app instance targets, but you can't assign permissions
* to manage all instances of an OIN app and then assign a subset of permissions to the same app. For example, you
* can't specify that an admin has access to manage all instances of the Salesforce app and then also manage
* specific configurations of the Salesforce app.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param appName
* Application name for the app type (required)
* @param appId
* Application ID (required)
*
* @throws ApiException
* if fails to make API call
*/
public void assignAppInstanceTargetToAppAdminRoleForGroup(String groupId, String roleAssignmentId, String appName,
String appId) throws ApiException {
this.assignAppInstanceTargetToAppAdminRoleForGroup(groupId, roleAssignmentId, appName, appId,
Collections.emptyMap());
}
/**
* Assign an Group Role Application Instance Target Assigns an app instance target to an `APP_ADMIN` Role
* Assignment to a Group. When you assign the first OIN app or app instance target, you reduce the scope of the Role
* Assignment. The Role no longer applies to all app targets, but applies only to the specified target. >
* **Note:** You can target a mixture of both OIN app and app instance targets, but you can't assign permissions
* to manage all instances of an OIN app and then assign a subset of permissions to the same app. For example, you
* can't specify that an admin has access to manage all instances of the Salesforce app and then also manage
* specific configurations of the Salesforce app.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param appName
* Application name for the app type (required)
* @param appId
* Application ID (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void assignAppInstanceTargetToAppAdminRoleForGroup(String groupId, String roleAssignmentId, String appName,
String appId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException(400,
"Missing the required parameter 'groupId' when calling assignAppInstanceTargetToAppAdminRoleForGroup");
}
// verify the required parameter 'roleAssignmentId' is set
if (roleAssignmentId == null) {
throw new ApiException(400,
"Missing the required parameter 'roleAssignmentId' when calling assignAppInstanceTargetToAppAdminRoleForGroup");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException(400,
"Missing the required parameter 'appName' when calling assignAppInstanceTargetToAppAdminRoleForGroup");
}
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(400,
"Missing the required parameter 'appId' when calling assignAppInstanceTargetToAppAdminRoleForGroup");
}
// create path and map variables
String localVarPath = "/api/v1/groups/{groupId}/roles/{roleAssignmentId}/targets/catalog/apps/{appName}/{appId}"
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "roleAssignmentId" + "\\}", apiClient.escapeString(roleAssignmentId.toString()))
.replaceAll("\\{" + "appName" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "appId" + "\\}", apiClient.escapeString(appId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Assign an Group Role Application Target Assigns an OIN app target to an `APP_ADMIN` Role Assignment to
* a Group. When you assign the first OIN app target, you reduce the scope of the Role Assignment. The Role no
* longer applies to all app targets but applies only to the specified target. An OIN app target that's assigned
* to the Role overrides any existing instance targets of the OIN app. For example, if a user is assigned to
* administer a specific Facebook instance, a successful request to add an OIN app with `facebook` for
* `appName` makes that user the administrator for all Facebook instances.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param appName
* Application name for the app type (required)
*
* @throws ApiException
* if fails to make API call
*/
public void assignAppTargetToAdminRoleForGroup(String groupId, String roleAssignmentId, String appName)
throws ApiException {
this.assignAppTargetToAdminRoleForGroup(groupId, roleAssignmentId, appName, Collections.emptyMap());
}
/**
* Assign an Group Role Application Target Assigns an OIN app target to an `APP_ADMIN` Role Assignment to
* a Group. When you assign the first OIN app target, you reduce the scope of the Role Assignment. The Role no
* longer applies to all app targets but applies only to the specified target. An OIN app target that's assigned
* to the Role overrides any existing instance targets of the OIN app. For example, if a user is assigned to
* administer a specific Facebook instance, a successful request to add an OIN app with `facebook` for
* `appName` makes that user the administrator for all Facebook instances.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param appName
* Application name for the app type (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void assignAppTargetToAdminRoleForGroup(String groupId, String roleAssignmentId, String appName,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException(400,
"Missing the required parameter 'groupId' when calling assignAppTargetToAdminRoleForGroup");
}
// verify the required parameter 'roleAssignmentId' is set
if (roleAssignmentId == null) {
throw new ApiException(400,
"Missing the required parameter 'roleAssignmentId' when calling assignAppTargetToAdminRoleForGroup");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException(400,
"Missing the required parameter 'appName' when calling assignAppTargetToAdminRoleForGroup");
}
// create path and map variables
String localVarPath = "/api/v1/groups/{groupId}/roles/{roleAssignmentId}/targets/catalog/apps/{appName}"
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "roleAssignmentId" + "\\}", apiClient.escapeString(roleAssignmentId.toString()))
.replaceAll("\\{" + "appName" + "\\}", apiClient.escapeString(appName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Assign a Group Role Group Target Assigns a Group target to a `USER_ADMIN`, `HELP_DESK_ADMIN`,
* or `GROUP_MEMBERSHIP_ADMIN` Role Assignment to a Group. When you assign the first Group target, you
* reduce the scope of the Role Assignment. The Role no longer applies to all targets but applies only to the
* specified target.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param targetGroupId
* (required)
*
* @throws ApiException
* if fails to make API call
*/
public void assignGroupTargetToGroupAdminRole(String groupId, String roleAssignmentId, String targetGroupId)
throws ApiException {
this.assignGroupTargetToGroupAdminRole(groupId, roleAssignmentId, targetGroupId, Collections.emptyMap());
}
/**
* Assign a Group Role Group Target Assigns a Group target to a `USER_ADMIN`, `HELP_DESK_ADMIN`,
* or `GROUP_MEMBERSHIP_ADMIN` Role Assignment to a Group. When you assign the first Group target, you
* reduce the scope of the Role Assignment. The Role no longer applies to all targets but applies only to the
* specified target.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param targetGroupId
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void assignGroupTargetToGroupAdminRole(String groupId, String roleAssignmentId, String targetGroupId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException(400,
"Missing the required parameter 'groupId' when calling assignGroupTargetToGroupAdminRole");
}
// verify the required parameter 'roleAssignmentId' is set
if (roleAssignmentId == null) {
throw new ApiException(400,
"Missing the required parameter 'roleAssignmentId' when calling assignGroupTargetToGroupAdminRole");
}
// verify the required parameter 'targetGroupId' is set
if (targetGroupId == null) {
throw new ApiException(400,
"Missing the required parameter 'targetGroupId' when calling assignGroupTargetToGroupAdminRole");
}
// create path and map variables
String localVarPath = "/api/v1/groups/{groupId}/roles/{roleAssignmentId}/targets/groups/{targetGroupId}"
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "roleAssignmentId" + "\\}", apiClient.escapeString(roleAssignmentId.toString()))
.replaceAll("\\{" + "targetGroupId" + "\\}", apiClient.escapeString(targetGroupId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* List all Group Role Application Targets Lists all app targets for an `APP_ADMIN` Role Assignment to a
* Group. The response includes a list of OIN-cataloged apps or app instances. The response payload for an app
* instance contains the `id` property, but an OIN-cataloged app doesn't.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param after
* (optional)
* @param limit
* (optional, default to 20)
*
* @return List<CatalogApplication>
*
* @throws ApiException
* if fails to make API call
*/
public List listApplicationTargetsForApplicationAdministratorRoleForGroup(String groupId,
String roleAssignmentId, String after, Integer limit) throws ApiException {
return this.listApplicationTargetsForApplicationAdministratorRoleForGroup(groupId, roleAssignmentId, after,
limit, Collections.emptyMap());
}
/**
* List all Group Role Application Targets Lists all app targets for an `APP_ADMIN` Role Assignment to a
* Group. The response includes a list of OIN-cataloged apps or app instances. The response payload for an app
* instance contains the `id` property, but an OIN-cataloged app doesn't.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param after
* (optional)
* @param limit
* (optional, default to 20)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<CatalogApplication>
*
* @throws ApiException
* if fails to make API call
*/
public List listApplicationTargetsForApplicationAdministratorRoleForGroup(String groupId,
String roleAssignmentId, String after, Integer limit, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException(400,
"Missing the required parameter 'groupId' when calling listApplicationTargetsForApplicationAdministratorRoleForGroup");
}
// verify the required parameter 'roleAssignmentId' is set
if (roleAssignmentId == null) {
throw new ApiException(400,
"Missing the required parameter 'roleAssignmentId' when calling listApplicationTargetsForApplicationAdministratorRoleForGroup");
}
// create path and map variables
String localVarPath = "/api/v1/groups/{groupId}/roles/{roleAssignmentId}/targets/catalog/apps"
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "roleAssignmentId" + "\\}", apiClient.escapeString(roleAssignmentId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Group Role Group Targets Lists all Group targets for a `USER_ADMIN`,
* `HELP_DESK_ADMIN`, or `GROUP_MEMBERSHIP_ADMIN` Role Assignment to a Group. If the Role
* isn't scoped to specific Group targets, an empty array `[]` is returned.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param after
* (optional)
* @param limit
* (optional, default to 20)
*
* @return List<Group>
*
* @throws ApiException
* if fails to make API call
*/
public List listGroupTargetsForGroupRole(String groupId, String roleAssignmentId, String after,
Integer limit) throws ApiException {
return this.listGroupTargetsForGroupRole(groupId, roleAssignmentId, after, limit, Collections.emptyMap());
}
/**
* List all Group Role Group Targets Lists all Group targets for a `USER_ADMIN`,
* `HELP_DESK_ADMIN`, or `GROUP_MEMBERSHIP_ADMIN` Role Assignment to a Group. If the Role
* isn't scoped to specific Group targets, an empty array `[]` is returned.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param after
* (optional)
* @param limit
* (optional, default to 20)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<Group>
*
* @throws ApiException
* if fails to make API call
*/
public List listGroupTargetsForGroupRole(String groupId, String roleAssignmentId, String after,
Integer limit, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException(400,
"Missing the required parameter 'groupId' when calling listGroupTargetsForGroupRole");
}
// verify the required parameter 'roleAssignmentId' is set
if (roleAssignmentId == null) {
throw new ApiException(400,
"Missing the required parameter 'roleAssignmentId' when calling listGroupTargetsForGroupRole");
}
// create path and map variables
String localVarPath = "/api/v1/groups/{groupId}/roles/{roleAssignmentId}/targets/groups"
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "roleAssignmentId" + "\\}", apiClient.escapeString(roleAssignmentId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Unassign an Group Role Application Instance Target Unassigns an app instance target from an `APP_ADMIN`
* Role Assignment to a Group > **Note:** You can't remove the last app instance target from a Role
* Assignment since this causes an exception. > If you need a Role Assignment that applies to all apps, delete
* the `APP_ADMIN` Role Assignment and recreate a new one. See [Unassign a Group
* Role](/openapi/okta-management/management/tag/RoleAssignmentBGroup/#tag/RoleAssignmentBGroup/operation/unassignRoleFromGroup).
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param appName
* Application name for the app type (required)
* @param appId
* Application ID (required)
*
* @throws ApiException
* if fails to make API call
*/
public void unassignAppInstanceTargetToAppAdminRoleForGroup(String groupId, String roleAssignmentId, String appName,
String appId) throws ApiException {
this.unassignAppInstanceTargetToAppAdminRoleForGroup(groupId, roleAssignmentId, appName, appId,
Collections.emptyMap());
}
/**
* Unassign an Group Role Application Instance Target Unassigns an app instance target from an `APP_ADMIN`
* Role Assignment to a Group > **Note:** You can't remove the last app instance target from a Role
* Assignment since this causes an exception. > If you need a Role Assignment that applies to all apps, delete
* the `APP_ADMIN` Role Assignment and recreate a new one. See [Unassign a Group
* Role](/openapi/okta-management/management/tag/RoleAssignmentBGroup/#tag/RoleAssignmentBGroup/operation/unassignRoleFromGroup).
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param appName
* Application name for the app type (required)
* @param appId
* Application ID (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void unassignAppInstanceTargetToAppAdminRoleForGroup(String groupId, String roleAssignmentId, String appName,
String appId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException(400,
"Missing the required parameter 'groupId' when calling unassignAppInstanceTargetToAppAdminRoleForGroup");
}
// verify the required parameter 'roleAssignmentId' is set
if (roleAssignmentId == null) {
throw new ApiException(400,
"Missing the required parameter 'roleAssignmentId' when calling unassignAppInstanceTargetToAppAdminRoleForGroup");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException(400,
"Missing the required parameter 'appName' when calling unassignAppInstanceTargetToAppAdminRoleForGroup");
}
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(400,
"Missing the required parameter 'appId' when calling unassignAppInstanceTargetToAppAdminRoleForGroup");
}
// create path and map variables
String localVarPath = "/api/v1/groups/{groupId}/roles/{roleAssignmentId}/targets/catalog/apps/{appName}/{appId}"
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "roleAssignmentId" + "\\}", apiClient.escapeString(roleAssignmentId.toString()))
.replaceAll("\\{" + "appName" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "appId" + "\\}", apiClient.escapeString(appId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Unassign a Group Role Application Target Unassigns an OIN app target from an `APP_ADMIN` Role
* Assignment to a Group > **Note:** You can't remove the last app target from a Role Assignment, since this
* causes an exception. > If you need a Role Assignment that applies to all apps, delete the
* `APP_ADMIN` Role Assignment and recreate a new one. See [Unassign a Group
* Role](/openapi/okta-management/management/tag/RoleAssignmentBGroup/#tag/RoleAssignmentBGroup/operation/unassignRoleFromGroup).
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param appName
* Application name for the app type (required)
*
* @throws ApiException
* if fails to make API call
*/
public void unassignAppTargetToAdminRoleForGroup(String groupId, String roleAssignmentId, String appName)
throws ApiException {
this.unassignAppTargetToAdminRoleForGroup(groupId, roleAssignmentId, appName, Collections.emptyMap());
}
/**
* Unassign a Group Role Application Target Unassigns an OIN app target from an `APP_ADMIN` Role
* Assignment to a Group > **Note:** You can't remove the last app target from a Role Assignment, since this
* causes an exception. > If you need a Role Assignment that applies to all apps, delete the
* `APP_ADMIN` Role Assignment and recreate a new one. See [Unassign a Group
* Role](/openapi/okta-management/management/tag/RoleAssignmentBGroup/#tag/RoleAssignmentBGroup/operation/unassignRoleFromGroup).
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param appName
* Application name for the app type (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void unassignAppTargetToAdminRoleForGroup(String groupId, String roleAssignmentId, String appName,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException(400,
"Missing the required parameter 'groupId' when calling unassignAppTargetToAdminRoleForGroup");
}
// verify the required parameter 'roleAssignmentId' is set
if (roleAssignmentId == null) {
throw new ApiException(400,
"Missing the required parameter 'roleAssignmentId' when calling unassignAppTargetToAdminRoleForGroup");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException(400,
"Missing the required parameter 'appName' when calling unassignAppTargetToAdminRoleForGroup");
}
// create path and map variables
String localVarPath = "/api/v1/groups/{groupId}/roles/{roleAssignmentId}/targets/catalog/apps/{appName}"
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "roleAssignmentId" + "\\}", apiClient.escapeString(roleAssignmentId.toString()))
.replaceAll("\\{" + "appName" + "\\}", apiClient.escapeString(appName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Unassign a Group Role Group Target Unassigns a Group target from a `USER_ADMIN`,
* `HELP_DESK_ADMIN`, or `GROUP_MEMBERSHIP_ADMIN` Role Assignment to a Group.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param targetGroupId
* (required)
*
* @throws ApiException
* if fails to make API call
*/
public void unassignGroupTargetFromGroupAdminRole(String groupId, String roleAssignmentId, String targetGroupId)
throws ApiException {
this.unassignGroupTargetFromGroupAdminRole(groupId, roleAssignmentId, targetGroupId, Collections.emptyMap());
}
/**
* Unassign a Group Role Group Target Unassigns a Group target from a `USER_ADMIN`,
* `HELP_DESK_ADMIN`, or `GROUP_MEMBERSHIP_ADMIN` Role Assignment to a Group.
*
* @param groupId
* The `id` of the group (required)
* @param roleAssignmentId
* The `id` of the Role Assignment (required)
* @param targetGroupId
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void unassignGroupTargetFromGroupAdminRole(String groupId, String roleAssignmentId, String targetGroupId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException(400,
"Missing the required parameter 'groupId' when calling unassignGroupTargetFromGroupAdminRole");
}
// verify the required parameter 'roleAssignmentId' is set
if (roleAssignmentId == null) {
throw new ApiException(400,
"Missing the required parameter 'roleAssignmentId' when calling unassignGroupTargetFromGroupAdminRole");
}
// verify the required parameter 'targetGroupId' is set
if (targetGroupId == null) {
throw new ApiException(400,
"Missing the required parameter 'targetGroupId' when calling unassignGroupTargetFromGroupAdminRole");
}
// create path and map variables
String localVarPath = "/api/v1/groups/{groupId}/roles/{roleAssignmentId}/targets/groups/{targetGroupId}"
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "roleAssignmentId" + "\\}", apiClient.escapeString(roleAssignmentId.toString()))
.replaceAll("\\{" + "targetGroupId" + "\\}", apiClient.escapeString(targetGroupId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy