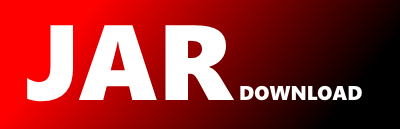
com.okta.sdk.resource.api.UserApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.CreateUserRequest;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.UpdateUserRequest;
import com.okta.sdk.resource.model.User;
import com.okta.sdk.resource.model.UserBlock;
import com.okta.sdk.resource.model.UserGetSingleton;
import com.okta.sdk.resource.model.UserNextLogin;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class UserApi {
private ApiClient apiClient;
public UserApi() {
this(Configuration.getDefaultApiClient());
}
public UserApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Create a User Creates a new User in your Okta org with or without credentials.<br> > **Legal
* Disclaimer** > > After a User is added to the Okta directory, they receive an activation email. As part of
* signing up for this service, > you agreed not to use Okta's service/product to spam and/or send
* unsolicited messages. > Please refrain from adding unrelated accounts to the directory as Okta is not
* responsible for, and disclaims any and all > liability associated with, the activation email's content.
* You, and you alone, bear responsibility for the emails sent to any recipients. All responses return the created
* User. Activation of a User is an asynchronous operation. The system performs group reconciliation during
* activation and assigns the User to all apps via direct or indirect relationships (group memberships). * The
* user's `transitioningToStatus` property is `ACTIVE` during activation to indicate that
* the User hasn't completed the asynchronous operation. * The user's `status` is
* `ACTIVE` when the activation process is complete. The User is emailed a one-time activation token if
* activated without a password. > **Note:** If the User is assigned to an app that is configured for
* provisioning, the activation process triggers downstream provisioning to the app. It is possible for a User to
* sign in before these apps have been successfully provisioned for the User. > **Important:** Do not generate or
* send a one-time activation token when activating Users with an assigned password. Users should sign in with their
* assigned password. For more information about the various scenarios of creating a user listed in the examples,
* see User Scenario Creations section in the [Users API](/openapi/okta-management/management/tag/User) description.
*
* @param body
* (required)
* @param activate
* Executes an [activation
* lifecycle](/openapi/okta-management/management/tag/UserLifecycle/#tag/UserLifecycle/operation/activateUser)
* operation when creating the User (optional, default to true)
* @param provider
* Indicates whether to create a User with a specified authentication provider (optional, default to
* false)
* @param nextLogin
* With `activate=true`, if `nextLogin=changePassword`, a User is created,
* activated, and the password is set to `EXPIRED`. The User must change it the next time they
* sign in. (optional)
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User createUser(CreateUserRequest body, Boolean activate, Boolean provider, UserNextLogin nextLogin)
throws ApiException {
return this.createUser(body, activate, provider, nextLogin, Collections.emptyMap());
}
/**
* Create a User Creates a new User in your Okta org with or without credentials.<br> > **Legal
* Disclaimer** > > After a User is added to the Okta directory, they receive an activation email. As part of
* signing up for this service, > you agreed not to use Okta's service/product to spam and/or send
* unsolicited messages. > Please refrain from adding unrelated accounts to the directory as Okta is not
* responsible for, and disclaims any and all > liability associated with, the activation email's content.
* You, and you alone, bear responsibility for the emails sent to any recipients. All responses return the created
* User. Activation of a User is an asynchronous operation. The system performs group reconciliation during
* activation and assigns the User to all apps via direct or indirect relationships (group memberships). * The
* user's `transitioningToStatus` property is `ACTIVE` during activation to indicate that
* the User hasn't completed the asynchronous operation. * The user's `status` is
* `ACTIVE` when the activation process is complete. The User is emailed a one-time activation token if
* activated without a password. > **Note:** If the User is assigned to an app that is configured for
* provisioning, the activation process triggers downstream provisioning to the app. It is possible for a User to
* sign in before these apps have been successfully provisioned for the User. > **Important:** Do not generate or
* send a one-time activation token when activating Users with an assigned password. Users should sign in with their
* assigned password. For more information about the various scenarios of creating a user listed in the examples,
* see User Scenario Creations section in the [Users API](/openapi/okta-management/management/tag/User) description.
*
* @param body
* (required)
* @param activate
* Executes an [activation
* lifecycle](/openapi/okta-management/management/tag/UserLifecycle/#tag/UserLifecycle/operation/activateUser)
* operation when creating the User (optional, default to true)
* @param provider
* Indicates whether to create a User with a specified authentication provider (optional, default to
* false)
* @param nextLogin
* With `activate=true`, if `nextLogin=changePassword`, a User is created,
* activated, and the password is set to `EXPIRED`. The User must change it the next time they
* sign in. (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User createUser(CreateUserRequest body, Boolean activate, Boolean provider, UserNextLogin nextLogin,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling createUser");
}
// create path and map variables
String localVarPath = "/api/v1/users";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("activate", activate));
localVarQueryParams.addAll(apiClient.parameterToPair("provider", provider));
localVarQueryParams.addAll(apiClient.parameterToPair("nextLogin", nextLogin));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Delete a User Deletes a User permanently. This operation can only be performed on Users that have a
* `DEPROVISIONED` status. **This action can't be recovered!** This operation on a User that
* hasn't been deactivated causes that User to be deactivated. A second delete operation is required to delete
* the User. > **Note:** You can also perform user deletion asynchronously. To invoke asynchronous user deletion,
* pass an HTTP header `Prefer: respond-async` with the request. This header is also supported by user
* deactivation, which is performed if the delete endpoint is invoked on a User that hasn't been deactivated.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param sendEmail
* Sends a deactivation email to the admin if `true` (optional, default to false)
* @param prefer
* (optional)
*
* @throws ApiException
* if fails to make API call
*/
public void deleteUser(String id, Boolean sendEmail, String prefer) throws ApiException {
this.deleteUser(id, sendEmail, prefer, Collections.emptyMap());
}
/**
* Delete a User Deletes a User permanently. This operation can only be performed on Users that have a
* `DEPROVISIONED` status. **This action can't be recovered!** This operation on a User that
* hasn't been deactivated causes that User to be deactivated. A second delete operation is required to delete
* the User. > **Note:** You can also perform user deletion asynchronously. To invoke asynchronous user deletion,
* pass an HTTP header `Prefer: respond-async` with the request. This header is also supported by user
* deactivation, which is performed if the delete endpoint is invoked on a User that hasn't been deactivated.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param sendEmail
* Sends a deactivation email to the admin if `true` (optional, default to false)
* @param prefer
* (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deleteUser(String id, Boolean sendEmail, String prefer, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling deleteUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{id}".replaceAll("\\{" + "id" + "\\}",
apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("sendEmail", sendEmail));
if (prefer != null)
localVarHeaderParams.put("Prefer", apiClient.parameterToString(prefer));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Retrieve a User Retrieves a User from your Okta org. > **Note:** You can substitute `me` for the
* `id` to fetch the current User linked to an API token or session cookie. > * The request returns the
* User linked to the API token that is specified in the Authorization header, not the User linked to the active
* session. Details of the Admin User who granted the API token is returned. > * When the end User has an active
* Okta session, it is typically a CORS request from the browser. Therefore, it's possible to retrieve the
* current User without the Authorization header. > **Note:** Some browsers block third-party cookies by default,
* which disrupts Okta functionality in certain flows. See [Mitigate the impact of third-party cookie
* deprecation](https://help.okta.com/okta_help.htm?type=oie&id=ext-third-party-cookies). >
* **Note:** When fetching a User by `login` or `login shortname`, [URL
* encode](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding) the request parameter to ensure that
* special characters are escaped properly. Logins with a `/` character can only be fetched by
* `id` due to URL issues with escaping the `/` character.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param contentType
* Specifies the media type of the resource. Optional `okta-response` value can be included for
* performance optimization. Complex DelAuth configurations may degrade performance when fetching
* specific parts of the response, and passing this parameter can omit these parts, bypassing the
* bottleneck. Enum values for `okta-response`: * `omitCredentials`: Omits the
* credentials subobject from the response. * `omitCredentialsLinks`: Omits the following HAL
* links from the response: Change Password, Change Recovery Question, Forgot Password, Reset Password,
* Reset Factors, Unlock. * `omitTransitioningToStatus`: Omits the
* `transitioningToStatus` field from the response. (optional)
* @param expand
* An optional parameter to include metadata in the `_embedded` attribute. Valid value:
* `blocks` (optional)
*
* @return UserGetSingleton
*
* @throws ApiException
* if fails to make API call
*/
public UserGetSingleton getUser(String id, String contentType, String expand) throws ApiException {
return this.getUser(id, contentType, expand, Collections.emptyMap());
}
/**
* Retrieve a User Retrieves a User from your Okta org. > **Note:** You can substitute `me` for the
* `id` to fetch the current User linked to an API token or session cookie. > * The request returns the
* User linked to the API token that is specified in the Authorization header, not the User linked to the active
* session. Details of the Admin User who granted the API token is returned. > * When the end User has an active
* Okta session, it is typically a CORS request from the browser. Therefore, it's possible to retrieve the
* current User without the Authorization header. > **Note:** Some browsers block third-party cookies by default,
* which disrupts Okta functionality in certain flows. See [Mitigate the impact of third-party cookie
* deprecation](https://help.okta.com/okta_help.htm?type=oie&id=ext-third-party-cookies). >
* **Note:** When fetching a User by `login` or `login shortname`, [URL
* encode](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding) the request parameter to ensure that
* special characters are escaped properly. Logins with a `/` character can only be fetched by
* `id` due to URL issues with escaping the `/` character.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param contentType
* Specifies the media type of the resource. Optional `okta-response` value can be included for
* performance optimization. Complex DelAuth configurations may degrade performance when fetching
* specific parts of the response, and passing this parameter can omit these parts, bypassing the
* bottleneck. Enum values for `okta-response`: * `omitCredentials`: Omits the
* credentials subobject from the response. * `omitCredentialsLinks`: Omits the following HAL
* links from the response: Change Password, Change Recovery Question, Forgot Password, Reset Password,
* Reset Factors, Unlock. * `omitTransitioningToStatus`: Omits the
* `transitioningToStatus` field from the response. (optional)
* @param expand
* An optional parameter to include metadata in the `_embedded` attribute. Valid value:
* `blocks` (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserGetSingleton
*
* @throws ApiException
* if fails to make API call
*/
public UserGetSingleton getUser(String id, String contentType, String expand, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{id}".replaceAll("\\{" + "id" + "\\}",
apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("expand", expand));
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all User Blocks Lists information about how the User is blocked from accessing their account
*
* @param userId
* ID of an existing Okta user (required)
*
* @return List<UserBlock>
*
* @throws ApiException
* if fails to make API call
*/
public List listUserBlocks(String userId) throws ApiException {
return this.listUserBlocks(userId, Collections.emptyMap());
}
/**
* List all User Blocks Lists information about how the User is blocked from accessing their account
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<UserBlock>
*
* @throws ApiException
* if fails to make API call
*/
public List listUserBlocks(String userId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling listUserBlocks");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/blocks".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all Users Lists Users in your org, with pagination in most cases. A subset of Users can be returned that
* match a supported filter expression or search criteria.
*
* @param contentType
* Specifies the media type of the resource. Optional `okta-response` value can be included for
* performance optimization. Complex DelAuth configurations may degrade performance when fetching
* specific parts of the response, and passing this parameter can omit these parts, bypassing the
* bottleneck. Enum values for `okta-response`: * `omitCredentials`: Omits the
* credentials subobject from the response. * `omitCredentialsLinks`: Omits the following HAL
* links from the response: Change Password, Change Recovery Question, Forgot Password, Reset Password,
* Reset Factors, Unlock. * `omitTransitioningToStatus`: Omits the
* `transitioningToStatus` field from the response. (optional)
* @param q
* Finds users who match the specified query. This doesn't support pagination. This might not deliver
* optimal performance for large orgs, and is deprecated for such use cases. To ensure optimal
* performance, use a [`search`
* parameter](/openapi/okta-management/management/tag/User/#tag/User/operation/listUsers!in=query&path=search&t=request)
* instead. Use the `q` parameter for a simple lookup of users by name, for example when
* creating a people picker. The value of `q` is matched against `firstName`,
* `lastName`, or `email`. This performs a `startsWith` match, but this is
* an implementation detail and can change without notice. You don't need to specify
* `firstName`, `lastName`, or `email`. (optional)
* @param after
* The cursor to use for pagination. It is an opaque string that specifies your current location in the
* list and is obtained from the `Link` response header. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param limit
* Specifies the number of results returned. Defaults to 10 if `q` is provided. (optional,
* default to 200)
* @param filter
* Filters users with a supported expression for a subset of properties. This requires [URL
* encoding](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding). For example,
* `filter=lastUpdated gt \"2013-06-01T00:00:00.000Z\"` is encoded as
* `filter=lastUpdated%20gt%20%222013-06-01T00:00:00.000Z%22`. Filtering is case-sensitive
* for attribute names and query values, while attribute operators are case-insensitive. Filtering
* supports the following limited number of properties: `status`, `lastUpdated`,
* `id`, `profile.login`, `profile.email`, `profile.firstName`,
* and `profile.lastName`. Additionally, filtering supports only the equal `eq`
* operator from the standard Okta API filtering semantics, except in the case of the
* `lastUpdated` property. This property can also use the inequality operators (`gt`,
* `ge`, `lt`, and `le`). For logical operators, only the logical operators
* `and` and `or` are supported. The `not` operator isn't supported.
* (optional)
* @param search
* Searches for users with a supported filtering expression for most properties. Okta recommends using
* this parameter for search for best performance. This operation supports
* [pagination](https://developer.okta.com/docs/api/#pagination). Use an ID lookup for records that you
* update to ensure your results contain the latest data. Property names in the search parameter are case
* sensitive, whereas operators (`eq`, `sw`, and so on) and string values are case
* insensitive. Unlike with user logins, diacritical marks are significant in search string values: a
* search for `isaac.brock` finds `Isaac.Brock`, but doesn't find a property
* whose value is `isáàc.bröck`. This operation requires [URL
* encoding](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding). For example,
* `search=profile.department eq \"Engineering\"` is encoded as
* `search=profile.department%20eq%20%22Engineering%22`. > **Note:** If you use the
* special character `\"` within a quoted string, it must also be escaped `\\`
* and encoded. For example, `search=profile.lastName eq \"bob\"smith\"` is
* encoded as `search=profile.lastName%20eq%20%22bob%5C%22smith%22`. This operation
* searches many properties: * Any user profile property, including custom-defined properties * The
* top-level properties `id`, `status`, `created`, `activated`,
* `statusChanged`, and `lastUpdated` * The [User
* Type](https://developer.okta.com/docs/reference/api/user-types/) accessed as `type.id` You
* can also use `sortBy` and `sortOrder` parameters. The `ne` (not equal)
* operator isn't supported, but you can obtain the same result by using `lt ... or ...
* gt`. For example, to see all users except those that have a status of `STAGED`, use
* `(status lt \"STAGED\" or status gt \"STAGED\")`. You can search
* properties that are arrays. If any element matches the search term, the entire array (object) is
* returned. Okta follows the [SCIM Protocol
* Specification](https://tools.ietf.org/html/rfc7644#section-3.4.2.2) for searching arrays. You can
* search multiple arrays, multiple values in an array, as well as using the standard logical and
* filtering operators. See [Filter](https://developer.okta.com/docs/reference/core-okta-api/#filter).
* (optional)
* @param sortBy
* Specifies field to sort by (for search queries only). This can be any single property, for example
* `sortBy=profile.lastName`. Users with the same value for the `sortBy`
* property will be ordered by `id`. (optional)
* @param sortOrder
* Specifies sort order asc or desc (for search queries only). Sorting is done in ASCII sort order (that
* is, by ASCII character value), but isn't case sensitive. `sortOrder` is ignored if
* `sortBy` is not present. (optional)
*
* @return List<User>
*
* @throws ApiException
* if fails to make API call
*/
public List listUsers(String contentType, String q, String after, Integer limit, String filter, String search,
String sortBy, String sortOrder) throws ApiException {
return this.listUsers(contentType, q, after, limit, filter, search, sortBy, sortOrder, Collections.emptyMap());
}
/**
* List all Users Lists Users in your org, with pagination in most cases. A subset of Users can be returned that
* match a supported filter expression or search criteria.
*
* @param contentType
* Specifies the media type of the resource. Optional `okta-response` value can be included for
* performance optimization. Complex DelAuth configurations may degrade performance when fetching
* specific parts of the response, and passing this parameter can omit these parts, bypassing the
* bottleneck. Enum values for `okta-response`: * `omitCredentials`: Omits the
* credentials subobject from the response. * `omitCredentialsLinks`: Omits the following HAL
* links from the response: Change Password, Change Recovery Question, Forgot Password, Reset Password,
* Reset Factors, Unlock. * `omitTransitioningToStatus`: Omits the
* `transitioningToStatus` field from the response. (optional)
* @param q
* Finds users who match the specified query. This doesn't support pagination. This might not deliver
* optimal performance for large orgs, and is deprecated for such use cases. To ensure optimal
* performance, use a [`search`
* parameter](/openapi/okta-management/management/tag/User/#tag/User/operation/listUsers!in=query&path=search&t=request)
* instead. Use the `q` parameter for a simple lookup of users by name, for example when
* creating a people picker. The value of `q` is matched against `firstName`,
* `lastName`, or `email`. This performs a `startsWith` match, but this is
* an implementation detail and can change without notice. You don't need to specify
* `firstName`, `lastName`, or `email`. (optional)
* @param after
* The cursor to use for pagination. It is an opaque string that specifies your current location in the
* list and is obtained from the `Link` response header. See
* [Pagination](https://developer.okta.com/docs/api/#pagination). (optional)
* @param limit
* Specifies the number of results returned. Defaults to 10 if `q` is provided. (optional,
* default to 200)
* @param filter
* Filters users with a supported expression for a subset of properties. This requires [URL
* encoding](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding). For example,
* `filter=lastUpdated gt \"2013-06-01T00:00:00.000Z\"` is encoded as
* `filter=lastUpdated%20gt%20%222013-06-01T00:00:00.000Z%22`. Filtering is case-sensitive
* for attribute names and query values, while attribute operators are case-insensitive. Filtering
* supports the following limited number of properties: `status`, `lastUpdated`,
* `id`, `profile.login`, `profile.email`, `profile.firstName`,
* and `profile.lastName`. Additionally, filtering supports only the equal `eq`
* operator from the standard Okta API filtering semantics, except in the case of the
* `lastUpdated` property. This property can also use the inequality operators (`gt`,
* `ge`, `lt`, and `le`). For logical operators, only the logical operators
* `and` and `or` are supported. The `not` operator isn't supported.
* (optional)
* @param search
* Searches for users with a supported filtering expression for most properties. Okta recommends using
* this parameter for search for best performance. This operation supports
* [pagination](https://developer.okta.com/docs/api/#pagination). Use an ID lookup for records that you
* update to ensure your results contain the latest data. Property names in the search parameter are case
* sensitive, whereas operators (`eq`, `sw`, and so on) and string values are case
* insensitive. Unlike with user logins, diacritical marks are significant in search string values: a
* search for `isaac.brock` finds `Isaac.Brock`, but doesn't find a property
* whose value is `isáàc.bröck`. This operation requires [URL
* encoding](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding). For example,
* `search=profile.department eq \"Engineering\"` is encoded as
* `search=profile.department%20eq%20%22Engineering%22`. > **Note:** If you use the
* special character `\"` within a quoted string, it must also be escaped `\\`
* and encoded. For example, `search=profile.lastName eq \"bob\"smith\"` is
* encoded as `search=profile.lastName%20eq%20%22bob%5C%22smith%22`. This operation
* searches many properties: * Any user profile property, including custom-defined properties * The
* top-level properties `id`, `status`, `created`, `activated`,
* `statusChanged`, and `lastUpdated` * The [User
* Type](https://developer.okta.com/docs/reference/api/user-types/) accessed as `type.id` You
* can also use `sortBy` and `sortOrder` parameters. The `ne` (not equal)
* operator isn't supported, but you can obtain the same result by using `lt ... or ...
* gt`. For example, to see all users except those that have a status of `STAGED`, use
* `(status lt \"STAGED\" or status gt \"STAGED\")`. You can search
* properties that are arrays. If any element matches the search term, the entire array (object) is
* returned. Okta follows the [SCIM Protocol
* Specification](https://tools.ietf.org/html/rfc7644#section-3.4.2.2) for searching arrays. You can
* search multiple arrays, multiple values in an array, as well as using the standard logical and
* filtering operators. See [Filter](https://developer.okta.com/docs/reference/core-okta-api/#filter).
* (optional)
* @param sortBy
* Specifies field to sort by (for search queries only). This can be any single property, for example
* `sortBy=profile.lastName`. Users with the same value for the `sortBy`
* property will be ordered by `id`. (optional)
* @param sortOrder
* Specifies sort order asc or desc (for search queries only). Sorting is done in ASCII sort order (that
* is, by ASCII character value), but isn't case sensitive. `sortOrder` is ignored if
* `sortBy` is not present. (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<User>
*
* @throws ApiException
* if fails to make API call
*/
public List listUsers(String contentType, String q, String after, Integer limit, String filter, String search,
String sortBy, String sortOrder, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/users";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("q", q));
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
localVarQueryParams.addAll(apiClient.parameterToPair("search", search));
localVarQueryParams.addAll(apiClient.parameterToPair("sortBy", sortBy));
localVarQueryParams.addAll(apiClient.parameterToPair("sortOrder", sortOrder));
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Replace a User Replaces a User's profile, credentials, or both using strict-update semantics. All profile
* properties must be specified when updating a User's profile with a `PUT` method. Any property not
* specified in the request is deleted. > **Important:** Don't use a `PUT` method for partial
* updates.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param user
* (required)
* @param strict
* If `true`, validates against minimum age and history password policy (optional)
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User replaceUser(String id, UpdateUserRequest user, Boolean strict) throws ApiException {
return this.replaceUser(id, user, strict, Collections.emptyMap());
}
/**
* Replace a User Replaces a User's profile, credentials, or both using strict-update semantics. All profile
* properties must be specified when updating a User's profile with a `PUT` method. Any property not
* specified in the request is deleted. > **Important:** Don't use a `PUT` method for partial
* updates.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param user
* (required)
* @param strict
* If `true`, validates against minimum age and history password policy (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User replaceUser(String id, UpdateUserRequest user, Boolean strict, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = user;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling replaceUser");
}
// verify the required parameter 'user' is set
if (user == null) {
throw new ApiException(400, "Missing the required parameter 'user' when calling replaceUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{id}".replaceAll("\\{" + "id" + "\\}",
apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("strict", strict));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Update a User Updates a user's profile or credentials with partial update semantics. > **Important:** Use
* the `POST` method for partial updates. Unspecified properties are set to null with `PUT`.
* `profile` and `credentials` can be updated independently or together with a single request.
* > **Note**: Currently, the User Type of a User can only be changed via a full replacement PUT operation. If
* the request parameters of a partial update include the type element from the User object, the value must match
* the existing type of the User. Only admins are permitted to change the User type of a User; end users are not
* allowed to change their own User type. > **Note**: To update a current user's profile with partial
* semantics, the `/api/v1/users/me` endpoint can be invoked. > > A User can only update profile
* properties for which the User has write access. Within the profile, if the User tries to update the primary or
* the secondary email IDs, verification emails are sent to those email IDs, and the fields are updated only upon
* verification. If you are using this endpoint to set a password, it sets a password without validating existing
* user credentials. This is an administrative operation. For operations that validate credentials, refer to the
* `Reset Password`, `Forgot Password`, and `Change Password` endpoints.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param user
* (required)
* @param strict
* If true, validates against minimum age and history password policy (optional)
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User updateUser(String id, UpdateUserRequest user, Boolean strict) throws ApiException {
return this.updateUser(id, user, strict, Collections.emptyMap());
}
/**
* Update a User Updates a user's profile or credentials with partial update semantics. > **Important:** Use
* the `POST` method for partial updates. Unspecified properties are set to null with `PUT`.
* `profile` and `credentials` can be updated independently or together with a single request.
* > **Note**: Currently, the User Type of a User can only be changed via a full replacement PUT operation. If
* the request parameters of a partial update include the type element from the User object, the value must match
* the existing type of the User. Only admins are permitted to change the User type of a User; end users are not
* allowed to change their own User type. > **Note**: To update a current user's profile with partial
* semantics, the `/api/v1/users/me` endpoint can be invoked. > > A User can only update profile
* properties for which the User has write access. Within the profile, if the User tries to update the primary or
* the secondary email IDs, verification emails are sent to those email IDs, and the fields are updated only upon
* verification. If you are using this endpoint to set a password, it sets a password without validating existing
* user credentials. This is an administrative operation. For operations that validate credentials, refer to the
* `Reset Password`, `Forgot Password`, and `Change Password` endpoints.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param user
* (required)
* @param strict
* If true, validates against minimum age and history password policy (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User updateUser(String id, UpdateUserRequest user, Boolean strict, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = user;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling updateUser");
}
// verify the required parameter 'user' is set
if (user == null) {
throw new ApiException(400, "Missing the required parameter 'user' when calling updateUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{id}".replaceAll("\\{" + "id" + "\\}",
apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("strict", strict));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy