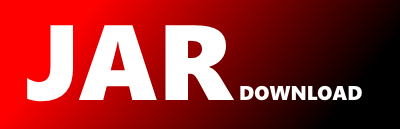
com.okta.sdk.resource.api.UserCredApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.ChangePasswordRequest;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.ForgotPasswordResponse;
import com.okta.sdk.resource.model.ResetPasswordToken;
import com.okta.sdk.resource.model.User;
import com.okta.sdk.resource.model.UserCredentials;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class UserCredApi {
private ApiClient apiClient;
public UserCredApi() {
this(Configuration.getDefaultApiClient());
}
public UserCredApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Update Password Updates a User's password by validating the User's current Password. This operation
* provides an option to delete all the sessions of the specified User. However, if the request is made in the
* context of a session owned by the specified User, that session isn't cleared. You can only perform this
* operation on Users in `STAGED`, `ACTIVE`, `PASSWORD_EXPIRED`, or
* `RECOVERY` status that have a valid [Password
* credential](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/User/#tag/User/operation/createUser!path=credentials/password&t=request).
* The User transitions to `ACTIVE` status when successfully invoked in `RECOVERY` status.
*
* @param userId
* ID of an existing Okta user (required)
* @param changePasswordRequest
* (required)
* @param strict
* If true, validates against password minimum age policy (optional, default to false)
*
* @return UserCredentials
*
* @throws ApiException
* if fails to make API call
*/
public UserCredentials changePassword(String userId, ChangePasswordRequest changePasswordRequest, Boolean strict)
throws ApiException {
return this.changePassword(userId, changePasswordRequest, strict, Collections.emptyMap());
}
/**
* Update Password Updates a User's password by validating the User's current Password. This operation
* provides an option to delete all the sessions of the specified User. However, if the request is made in the
* context of a session owned by the specified User, that session isn't cleared. You can only perform this
* operation on Users in `STAGED`, `ACTIVE`, `PASSWORD_EXPIRED`, or
* `RECOVERY` status that have a valid [Password
* credential](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/User/#tag/User/operation/createUser!path=credentials/password&t=request).
* The User transitions to `ACTIVE` status when successfully invoked in `RECOVERY` status.
*
* @param userId
* ID of an existing Okta user (required)
* @param changePasswordRequest
* (required)
* @param strict
* If true, validates against password minimum age policy (optional, default to false)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserCredentials
*
* @throws ApiException
* if fails to make API call
*/
public UserCredentials changePassword(String userId, ChangePasswordRequest changePasswordRequest, Boolean strict,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = changePasswordRequest;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling changePassword");
}
// verify the required parameter 'changePasswordRequest' is set
if (changePasswordRequest == null) {
throw new ApiException(400,
"Missing the required parameter 'changePasswordRequest' when calling changePassword");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/credentials/change_password".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("strict", strict));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Update Recovery Question Updates a User's Recovery Question and answer credential by validating the
* User's current Password. You can only perform this operation on Users in `STAGED`,
* `ACTIVE`, or `RECOVERY` status that have a valid [Password
* credential](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/User/#tag/User/operation/createUser!path=credentials/password&t=request).
*
* @param userId
* ID of an existing Okta user (required)
* @param userCredentials
* (required)
*
* @return UserCredentials
*
* @throws ApiException
* if fails to make API call
*/
public UserCredentials changeRecoveryQuestion(String userId, UserCredentials userCredentials) throws ApiException {
return this.changeRecoveryQuestion(userId, userCredentials, Collections.emptyMap());
}
/**
* Update Recovery Question Updates a User's Recovery Question and answer credential by validating the
* User's current Password. You can only perform this operation on Users in `STAGED`,
* `ACTIVE`, or `RECOVERY` status that have a valid [Password
* credential](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/User/#tag/User/operation/createUser!path=credentials/password&t=request).
*
* @param userId
* ID of an existing Okta user (required)
* @param userCredentials
* (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserCredentials
*
* @throws ApiException
* if fails to make API call
*/
public UserCredentials changeRecoveryQuestion(String userId, UserCredentials userCredentials,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = userCredentials;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling changeRecoveryQuestion");
}
// verify the required parameter 'userCredentials' is set
if (userCredentials == null) {
throw new ApiException(400,
"Missing the required parameter 'userCredentials' when calling changeRecoveryQuestion");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/credentials/change_recovery_question"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Expire Password Expires the password. This operation transitions the User status to `PASSWORD_EXPIRED`
* so that the User must change their password the next time that they sign in. <br> If you have integrated
* Okta with your on-premises Active Directory (AD), then setting a User's Password as expired in Okta also
* expires the Password in AD. When the User tries to sign in to Okta, delegated authentication finds the
* password-expired status in AD, and the User is presented with the password-expired page where they can change
* their Password.
*
* @param userId
* ID of an existing Okta user (required)
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User expirePassword(String userId) throws ApiException {
return this.expirePassword(userId, Collections.emptyMap());
}
/**
* Expire Password Expires the password. This operation transitions the User status to `PASSWORD_EXPIRED`
* so that the User must change their password the next time that they sign in. <br> If you have integrated
* Okta with your on-premises Active Directory (AD), then setting a User's Password as expired in Okta also
* expires the Password in AD. When the User tries to sign in to Okta, delegated authentication finds the
* password-expired status in AD, and the User is presented with the password-expired page where they can change
* their Password.
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User expirePassword(String userId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling expirePassword");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/expire_password".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Expire Password with temporary password Expires the password and resets the User's Password to a temporary
* password. This operation transitions the User status to `PASSWORD_EXPIRED` so that the User must change
* their password the next time that they sign in. User's Password is reset to a temporary password that is
* returned, and then the User's Password is expired. If `revokeSessions` is included in the request
* with a value of `true`, the User's current outstanding sessions are revoked and require
* re-authentication. <br> If you have integrated Okta with your on-premises Active Directory (AD), then
* setting a User's Password as expired in Okta also expires the Password in AD. When the User tries to sign in
* to Okta, delegated authentication finds the password-expired status in AD, and the User is presented with the
* password-expired page where they can change their Password.
*
* @param userId
* ID of an existing Okta user (required)
* @param revokeSessions
* Revokes the User's existing sessions if `true` (optional, default to false)
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User expirePasswordWithTempPassword(String userId, Boolean revokeSessions) throws ApiException {
return this.expirePasswordWithTempPassword(userId, revokeSessions, Collections.emptyMap());
}
/**
* Expire Password with temporary password Expires the password and resets the User's Password to a temporary
* password. This operation transitions the User status to `PASSWORD_EXPIRED` so that the User must change
* their password the next time that they sign in. User's Password is reset to a temporary password that is
* returned, and then the User's Password is expired. If `revokeSessions` is included in the request
* with a value of `true`, the User's current outstanding sessions are revoked and require
* re-authentication. <br> If you have integrated Okta with your on-premises Active Directory (AD), then
* setting a User's Password as expired in Okta also expires the Password in AD. When the User tries to sign in
* to Okta, delegated authentication finds the password-expired status in AD, and the User is presented with the
* password-expired page where they can change their Password.
*
* @param userId
* ID of an existing Okta user (required)
* @param revokeSessions
* Revokes the User's existing sessions if `true` (optional, default to false)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return User
*
* @throws ApiException
* if fails to make API call
*/
public User expirePasswordWithTempPassword(String userId, Boolean revokeSessions,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400,
"Missing the required parameter 'userId' when calling expirePasswordWithTempPassword");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/expire_password_with_temp_password"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("revokeSessions", revokeSessions));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Start forgot password flow Starts the forgot password flow. Generates a one-time token (OTT) that you can use to
* reset a User's Password. The User must validate their security question's answer when visiting the reset
* link. This operation can only be performed on Users with an ACTIVE status and a valid [Recovery Question
* credential](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/User/#tag/User/operation/createUser!path=credentials/recovery_question&t=request).
* > **Note:** If you have migrated to Identity Engine, you can allow Users to recover passwords with any
* enrolled MFA authenticator. See [Self-service account
* recovery](https://help.okta.com/oie/en-us/content/topics/identity-engine/authenticators/configure-sspr.htm?cshid=ext-config-sspr)
* If an email address is associated with multiple Users, keep in mind the following to ensure a successful password
* recovery lookup: * Okta no longer includes deactivated Users in the lookup. * The lookup searches sign-in IDs
* first, then primary email addresses, and then secondary email addresses. If `sendEmail` is
* `false`, returns a link for the User to reset their Password. This operation doesn't affect the
* status of the User.
*
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* Sends a forgot password email to the User if `true` (optional, default to true)
*
* @return ForgotPasswordResponse
*
* @throws ApiException
* if fails to make API call
*/
public ForgotPasswordResponse forgotPassword(String userId, Boolean sendEmail) throws ApiException {
return this.forgotPassword(userId, sendEmail, Collections.emptyMap());
}
/**
* Start forgot password flow Starts the forgot password flow. Generates a one-time token (OTT) that you can use to
* reset a User's Password. The User must validate their security question's answer when visiting the reset
* link. This operation can only be performed on Users with an ACTIVE status and a valid [Recovery Question
* credential](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/User/#tag/User/operation/createUser!path=credentials/recovery_question&t=request).
* > **Note:** If you have migrated to Identity Engine, you can allow Users to recover passwords with any
* enrolled MFA authenticator. See [Self-service account
* recovery](https://help.okta.com/oie/en-us/content/topics/identity-engine/authenticators/configure-sspr.htm?cshid=ext-config-sspr)
* If an email address is associated with multiple Users, keep in mind the following to ensure a successful password
* recovery lookup: * Okta no longer includes deactivated Users in the lookup. * The lookup searches sign-in IDs
* first, then primary email addresses, and then secondary email addresses. If `sendEmail` is
* `false`, returns a link for the User to reset their Password. This operation doesn't affect the
* status of the User.
*
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* Sends a forgot password email to the User if `true` (optional, default to true)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return ForgotPasswordResponse
*
* @throws ApiException
* if fails to make API call
*/
public ForgotPasswordResponse forgotPassword(String userId, Boolean sendEmail,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling forgotPassword");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/credentials/forgot_password".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("sendEmail", sendEmail));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Reset Password with Recovery Question Resets the User's password to the specified password if the provided
* answer to the recovery question is correct. You must include the Recovery Question answer with the submission.
*
* @param userId
* ID of an existing Okta user (required)
* @param userCredentials
* (required)
* @param sendEmail
* (optional, default to true)
*
* @return UserCredentials
*
* @throws ApiException
* if fails to make API call
*/
public UserCredentials forgotPasswordSetNewPassword(String userId, UserCredentials userCredentials,
Boolean sendEmail) throws ApiException {
return this.forgotPasswordSetNewPassword(userId, userCredentials, sendEmail, Collections.emptyMap());
}
/**
* Reset Password with Recovery Question Resets the User's password to the specified password if the provided
* answer to the recovery question is correct. You must include the Recovery Question answer with the submission.
*
* @param userId
* ID of an existing Okta user (required)
* @param userCredentials
* (required)
* @param sendEmail
* (optional, default to true)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserCredentials
*
* @throws ApiException
* if fails to make API call
*/
public UserCredentials forgotPasswordSetNewPassword(String userId, UserCredentials userCredentials,
Boolean sendEmail, Map additionalHeaders) throws ApiException {
Object localVarPostBody = userCredentials;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400,
"Missing the required parameter 'userId' when calling forgotPasswordSetNewPassword");
}
// verify the required parameter 'userCredentials' is set
if (userCredentials == null) {
throw new ApiException(400,
"Missing the required parameter 'userCredentials' when calling forgotPasswordSetNewPassword");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/credentials/forgot_password_recovery_question"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("sendEmail", sendEmail));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Reset password Resets password. Generates a one-time token (OTT) that you can use to reset a User's password.
* You can automatically email the OTT link to the User or return the OTT to the API caller and distribute using a
* custom flow. This operation transitions the User to the `RECOVERY` status. The User is then not able to
* sign in or initiate a forgot password flow until they complete the reset flow. This operation provides an option
* to delete all the User's sessions. However, if the request is made in the context of a session owned by the
* specified User, that session isn't cleared. > **Note:** You can also use this API to convert a User with
* the Okta Credential Provider to use a Federated Provider. After this conversion, the User can't directly sign
* in with a password. > To convert a federated User back to an Okta User, use the default API call. If an email
* address is associated with multiple Users, keep in mind the following to ensure a successful password recovery
* lookup: * Okta no longer includes deactivated Users in the lookup. * The lookup searches sign-in IDs first, then
* primary email addresses, and then secondary email addresses. If `sendEmail` is `false`,
* returns a link for the User to reset their password.
*
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* (required)
* @param revokeSessions
* Revokes all User sessions, except for the current session, if set to `true` (optional,
* default to false)
*
* @return ResetPasswordToken
*
* @throws ApiException
* if fails to make API call
*/
public ResetPasswordToken resetPassword(String userId, Boolean sendEmail, Boolean revokeSessions)
throws ApiException {
return this.resetPassword(userId, sendEmail, revokeSessions, Collections.emptyMap());
}
/**
* Reset password Resets password. Generates a one-time token (OTT) that you can use to reset a User's password.
* You can automatically email the OTT link to the User or return the OTT to the API caller and distribute using a
* custom flow. This operation transitions the User to the `RECOVERY` status. The User is then not able to
* sign in or initiate a forgot password flow until they complete the reset flow. This operation provides an option
* to delete all the User's sessions. However, if the request is made in the context of a session owned by the
* specified User, that session isn't cleared. > **Note:** You can also use this API to convert a User with
* the Okta Credential Provider to use a Federated Provider. After this conversion, the User can't directly sign
* in with a password. > To convert a federated User back to an Okta User, use the default API call. If an email
* address is associated with multiple Users, keep in mind the following to ensure a successful password recovery
* lookup: * Okta no longer includes deactivated Users in the lookup. * The lookup searches sign-in IDs first, then
* primary email addresses, and then secondary email addresses. If `sendEmail` is `false`,
* returns a link for the User to reset their password.
*
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* (required)
* @param revokeSessions
* Revokes all User sessions, except for the current session, if set to `true` (optional,
* default to false)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return ResetPasswordToken
*
* @throws ApiException
* if fails to make API call
*/
public ResetPasswordToken resetPassword(String userId, Boolean sendEmail, Boolean revokeSessions,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling resetPassword");
}
// verify the required parameter 'sendEmail' is set
if (sendEmail == null) {
throw new ApiException(400, "Missing the required parameter 'sendEmail' when calling resetPassword");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/reset_password".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("sendEmail", sendEmail));
localVarQueryParams.addAll(apiClient.parameterToPair("revokeSessions", revokeSessions));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy