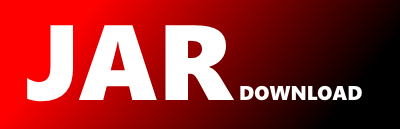
com.okta.sdk.resource.api.UserFactorApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.ResendUserFactor;
import com.okta.sdk.resource.model.UploadYubikeyOtpTokenSeedRequest;
import com.okta.sdk.resource.model.UserFactor;
import com.okta.sdk.resource.model.UserFactorActivateRequest;
import com.okta.sdk.resource.model.UserFactorActivateResponse;
import com.okta.sdk.resource.model.UserFactorPushTransaction;
import com.okta.sdk.resource.model.UserFactorSecurityQuestionProfile;
import com.okta.sdk.resource.model.UserFactorSupported;
import com.okta.sdk.resource.model.UserFactorVerifyRequest;
import com.okta.sdk.resource.model.UserFactorVerifyResponse;
import com.okta.sdk.resource.model.UserFactorYubikeyOtpToken;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class UserFactorApi {
private ApiClient apiClient;
public UserFactorApi() {
this(Configuration.getDefaultApiClient());
}
public UserFactorApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Activate a Factor Activates a Factor. Some Factors (`call`, `email`, `push`,
* `sms`, `token:software:totp`, `u2f`, and `webauthn`) require activation
* to complete the enrollment process. Okta enforces a rate limit of five activation attempts within five minutes.
* After a user exceeds the rate limit, Okta returns an error message. > **Note**: If the user exceeds their SMS,
* call, or email factor activate rate limit, then an OTP resend request
* (`/api/v1/users/${userId}}/factors/${factorId}/resend`) isn't allowed for the same Factor.
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param body
* (optional)
*
* @return UserFactorActivateResponse
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorActivateResponse activateFactor(String userId, String factorId, UserFactorActivateRequest body)
throws ApiException {
return this.activateFactor(userId, factorId, body, Collections.emptyMap());
}
/**
* Activate a Factor Activates a Factor. Some Factors (`call`, `email`, `push`,
* `sms`, `token:software:totp`, `u2f`, and `webauthn`) require activation
* to complete the enrollment process. Okta enforces a rate limit of five activation attempts within five minutes.
* After a user exceeds the rate limit, Okta returns an error message. > **Note**: If the user exceeds their SMS,
* call, or email factor activate rate limit, then an OTP resend request
* (`/api/v1/users/${userId}}/factors/${factorId}/resend`) isn't allowed for the same Factor.
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param body
* (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserFactorActivateResponse
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorActivateResponse activateFactor(String userId, String factorId, UserFactorActivateRequest body,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling activateFactor");
}
// verify the required parameter 'factorId' is set
if (factorId == null) {
throw new ApiException(400, "Missing the required parameter 'factorId' when calling activateFactor");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors/{factorId}/lifecycle/activate"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()))
.replaceAll("\\{" + "factorId" + "\\}", apiClient.escapeString(factorId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Enroll a Factor Enrolls a supported Factor for the specified user > **Note:** All responses return the
* enrolled Factor with a status of either `PENDING_ACTIVATION`` or `ACTIVE`. ####
* Additional SMS/Call Factor information * **Rate limits**: Okta may return a `429 Too Many Requests`
* status code if you attempt to resend an SMS or a voice call challenge (OTP) within the same time window. The
* current [rate limit](https://developer.okta.com/docs/reference/rate-limits/) is one SMS/CALL challenge per phone
* number every 30 seconds. * **Existing phone numbers**: Okta may return a `400 Bad Request` status code
* if a user attempts to enroll with a different phone number when the user has an existing mobile phone or has an
* existing phone with voice call capability. A user can enroll only one mobile phone for `sms` and enroll
* only one voice call capable phone for `call` factor. #### Additional WebAuthn Factor information
* **Enroll WebAuthn response parameters** * For detailed information on the Webauthn standard, including an
* up-to-date list of supported browsers, see [webauthn.me](https://a0.to/webauthnme-okta-docs). * In the enroll API
* response, the `response._embedded.activation` object contains properties used to help the client to
* create a new WebAuthn credential for use with Okta. See the [WebAuthn spec for
* PublicKeyCredentialCreationOptions](https://www.w3.org/TR/webauthn/#dictionary-makecredentialoptions). ####
* Additional Custom TOTP Factor information **Enroll Custom TOTP Factor** * The enrollment process involves passing
* both the `factorProfileId` and `sharedSecret` properties for a token. * A Factor Profile
* represents a particular configuration of the Custom TOTP factor. It includes certain properties that match the
* hardware token that end users possess, such as the HMAC algorithm, passcode length, and time interval. There can
* be multiple Custom TOTP factor profiles per org, but users can only enroll in one Custom TOTP factor. Admins can
* [create Custom TOTP factor profiles](https://help.okta.com/okta_help.htm?id=ext-mfa-totp) in the Admin
* Console. Then, copy the `factorProfileId` from the Admin Console into the API request. *
* <x-lifecycle class=\"oie\"></x-lifecycle> For Custom TOTP enrollment, Okta
* automaticaly enrolls a user with a `token:software:totp` factor and the `push` factor if the
* user isn't currently enrolled with these factors.
*
* @param userId
* ID of an existing Okta user (required)
* @param body
* Factor (required)
* @param updatePhone
* If `true`, indicates that you are replacing the currently registered phone number for the
* specified user. This parameter is ignored if the existing phone number is used by an activated Factor.
* (optional, default to false)
* @param templateId
* ID of an existing custom SMS template. See the [SMS Templates API](../Template). This parameter is
* only used by `sms` Factors. If the provided ID doesn't exist, the default template is
* used instead. (optional)
* @param tokenLifetimeSeconds
* Defines how long the token remains valid (optional, default to 300)
* @param activate
* If `true`, the factor is immediately activated as part of the enrollment. An activation
* process isn't required. Currently auto-activation is supported by `sms`,
* `call`, `email` and `token:hotp` (Custom TOTP) Factor. (optional,
* default to false)
* @param acceptLanguage
* An ISO 639-1 two-letter language code that defines a localized message to send. This parameter is only
* used by `sms` Factors. If a localized message doesn't exist or the
* `templateId` is incorrect, the default template is used instead. (optional)
*
* @return UserFactor
*
* @throws ApiException
* if fails to make API call
*/
public UserFactor enrollFactor(String userId, UserFactor body, Boolean updatePhone, String templateId,
Integer tokenLifetimeSeconds, Boolean activate, String acceptLanguage) throws ApiException {
return this.enrollFactor(userId, body, updatePhone, templateId, tokenLifetimeSeconds, activate, acceptLanguage,
Collections.emptyMap());
}
/**
* Enroll a Factor Enrolls a supported Factor for the specified user > **Note:** All responses return the
* enrolled Factor with a status of either `PENDING_ACTIVATION`` or `ACTIVE`. ####
* Additional SMS/Call Factor information * **Rate limits**: Okta may return a `429 Too Many Requests`
* status code if you attempt to resend an SMS or a voice call challenge (OTP) within the same time window. The
* current [rate limit](https://developer.okta.com/docs/reference/rate-limits/) is one SMS/CALL challenge per phone
* number every 30 seconds. * **Existing phone numbers**: Okta may return a `400 Bad Request` status code
* if a user attempts to enroll with a different phone number when the user has an existing mobile phone or has an
* existing phone with voice call capability. A user can enroll only one mobile phone for `sms` and enroll
* only one voice call capable phone for `call` factor. #### Additional WebAuthn Factor information
* **Enroll WebAuthn response parameters** * For detailed information on the Webauthn standard, including an
* up-to-date list of supported browsers, see [webauthn.me](https://a0.to/webauthnme-okta-docs). * In the enroll API
* response, the `response._embedded.activation` object contains properties used to help the client to
* create a new WebAuthn credential for use with Okta. See the [WebAuthn spec for
* PublicKeyCredentialCreationOptions](https://www.w3.org/TR/webauthn/#dictionary-makecredentialoptions). ####
* Additional Custom TOTP Factor information **Enroll Custom TOTP Factor** * The enrollment process involves passing
* both the `factorProfileId` and `sharedSecret` properties for a token. * A Factor Profile
* represents a particular configuration of the Custom TOTP factor. It includes certain properties that match the
* hardware token that end users possess, such as the HMAC algorithm, passcode length, and time interval. There can
* be multiple Custom TOTP factor profiles per org, but users can only enroll in one Custom TOTP factor. Admins can
* [create Custom TOTP factor profiles](https://help.okta.com/okta_help.htm?id=ext-mfa-totp) in the Admin
* Console. Then, copy the `factorProfileId` from the Admin Console into the API request. *
* <x-lifecycle class=\"oie\"></x-lifecycle> For Custom TOTP enrollment, Okta
* automaticaly enrolls a user with a `token:software:totp` factor and the `push` factor if the
* user isn't currently enrolled with these factors.
*
* @param userId
* ID of an existing Okta user (required)
* @param body
* Factor (required)
* @param updatePhone
* If `true`, indicates that you are replacing the currently registered phone number for the
* specified user. This parameter is ignored if the existing phone number is used by an activated Factor.
* (optional, default to false)
* @param templateId
* ID of an existing custom SMS template. See the [SMS Templates API](../Template). This parameter is
* only used by `sms` Factors. If the provided ID doesn't exist, the default template is
* used instead. (optional)
* @param tokenLifetimeSeconds
* Defines how long the token remains valid (optional, default to 300)
* @param activate
* If `true`, the factor is immediately activated as part of the enrollment. An activation
* process isn't required. Currently auto-activation is supported by `sms`,
* `call`, `email` and `token:hotp` (Custom TOTP) Factor. (optional,
* default to false)
* @param acceptLanguage
* An ISO 639-1 two-letter language code that defines a localized message to send. This parameter is only
* used by `sms` Factors. If a localized message doesn't exist or the
* `templateId` is incorrect, the default template is used instead. (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserFactor
*
* @throws ApiException
* if fails to make API call
*/
public UserFactor enrollFactor(String userId, UserFactor body, Boolean updatePhone, String templateId,
Integer tokenLifetimeSeconds, Boolean activate, String acceptLanguage,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling enrollFactor");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling enrollFactor");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("updatePhone", updatePhone));
localVarQueryParams.addAll(apiClient.parameterToPair("templateId", templateId));
localVarQueryParams.addAll(apiClient.parameterToPair("tokenLifetimeSeconds", tokenLifetimeSeconds));
localVarQueryParams.addAll(apiClient.parameterToPair("activate", activate));
if (acceptLanguage != null)
localVarHeaderParams.put("Accept-Language", apiClient.parameterToString(acceptLanguage));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve a Factor Retrieves an existing Factor for the specified user
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
*
* @return UserFactor
*
* @throws ApiException
* if fails to make API call
*/
public UserFactor getFactor(String userId, String factorId) throws ApiException {
return this.getFactor(userId, factorId, Collections.emptyMap());
}
/**
* Retrieve a Factor Retrieves an existing Factor for the specified user
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserFactor
*
* @throws ApiException
* if fails to make API call
*/
public UserFactor getFactor(String userId, String factorId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling getFactor");
}
// verify the required parameter 'factorId' is set
if (factorId == null) {
throw new ApiException(400, "Missing the required parameter 'factorId' when calling getFactor");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors/{factorId}"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()))
.replaceAll("\\{" + "factorId" + "\\}", apiClient.escapeString(factorId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve a Factor transaction status Retrieves the status of a `push` Factor verification transaction
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param transactionId
* ID of an existing Factor verification transaction (required)
*
* @return UserFactorPushTransaction
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorPushTransaction getFactorTransactionStatus(String userId, String factorId, String transactionId)
throws ApiException {
return this.getFactorTransactionStatus(userId, factorId, transactionId, Collections.emptyMap());
}
/**
* Retrieve a Factor transaction status Retrieves the status of a `push` Factor verification transaction
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param transactionId
* ID of an existing Factor verification transaction (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserFactorPushTransaction
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorPushTransaction getFactorTransactionStatus(String userId, String factorId, String transactionId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400,
"Missing the required parameter 'userId' when calling getFactorTransactionStatus");
}
// verify the required parameter 'factorId' is set
if (factorId == null) {
throw new ApiException(400,
"Missing the required parameter 'factorId' when calling getFactorTransactionStatus");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new ApiException(400,
"Missing the required parameter 'transactionId' when calling getFactorTransactionStatus");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors/{factorId}/transactions/{transactionId}"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()))
.replaceAll("\\{" + "factorId" + "\\}", apiClient.escapeString(factorId.toString()))
.replaceAll("\\{" + "transactionId" + "\\}", apiClient.escapeString(transactionId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve a YubiKey OTP Token Retrieves the specified YubiKey OTP Token by `id`
*
* @param tokenId
* ID of a Yubikey token (required)
*
* @return UserFactorYubikeyOtpToken
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorYubikeyOtpToken getYubikeyOtpTokenById(String tokenId) throws ApiException {
return this.getYubikeyOtpTokenById(tokenId, Collections.emptyMap());
}
/**
* Retrieve a YubiKey OTP Token Retrieves the specified YubiKey OTP Token by `id`
*
* @param tokenId
* ID of a Yubikey token (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserFactorYubikeyOtpToken
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorYubikeyOtpToken getYubikeyOtpTokenById(String tokenId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'tokenId' is set
if (tokenId == null) {
throw new ApiException(400, "Missing the required parameter 'tokenId' when calling getYubikeyOtpTokenById");
}
// create path and map variables
String localVarPath = "/api/v1/org/factors/yubikey_token/tokens/{tokenId}".replaceAll("\\{" + "tokenId" + "\\}",
apiClient.escapeString(tokenId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all enrolled Factors Lists all enrolled Factors for the specified user
*
* @param userId
* ID of an existing Okta user (required)
*
* @return List<UserFactor>
*
* @throws ApiException
* if fails to make API call
*/
public List listFactors(String userId) throws ApiException {
return this.listFactors(userId, Collections.emptyMap());
}
/**
* List all enrolled Factors Lists all enrolled Factors for the specified user
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<UserFactor>
*
* @throws ApiException
* if fails to make API call
*/
public List listFactors(String userId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling listFactors");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all supported Factors Lists all the supported Factors that can be enrolled for the specified user
*
* @param userId
* ID of an existing Okta user (required)
*
* @return List<UserFactorSupported>
*
* @throws ApiException
* if fails to make API call
*/
public List listSupportedFactors(String userId) throws ApiException {
return this.listSupportedFactors(userId, Collections.emptyMap());
}
/**
* List all supported Factors Lists all the supported Factors that can be enrolled for the specified user
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<UserFactorSupported>
*
* @throws ApiException
* if fails to make API call
*/
public List listSupportedFactors(String userId, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling listSupportedFactors");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors/catalog".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all supported Security Questions Lists all available Security Questions for the specified user
*
* @param userId
* ID of an existing Okta user (required)
*
* @return List<UserFactorSecurityQuestionProfile>
*
* @throws ApiException
* if fails to make API call
*/
public List listSupportedSecurityQuestions(String userId) throws ApiException {
return this.listSupportedSecurityQuestions(userId, Collections.emptyMap());
}
/**
* List all supported Security Questions Lists all available Security Questions for the specified user
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<UserFactorSecurityQuestionProfile>
*
* @throws ApiException
* if fails to make API call
*/
public List listSupportedSecurityQuestions(String userId,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400,
"Missing the required parameter 'userId' when calling listSupportedSecurityQuestions");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors/questions".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* List all YubiKey OTP Tokens Lists all YubiKey OTP Tokens
*
* @param after
* Specifies the pagination cursor for the next page of tokens (optional)
* @param expand
* Embeds the [User](/openapi/okta-management/management/tag/User/) resource if the YubiKey Token is
* assigned to a user and `expand` is set to `user` (optional)
* @param filter
* The expression used to filter tokens (optional)
* @param forDownload
* Returns tokens in a CSV to download instead of in the response. When you use this query parameter, the
* `limit` default changes to 1000. (optional, default to false)
* @param limit
* Specifies the number of results per page (optional, default to 20)
* @param sortBy
* The value of how the tokens are sorted (optional)
* @param sortOrder
* Specifies the sort order, either `ASC` or `DESC` (optional)
*
* @return List<UserFactorYubikeyOtpToken>
*
* @throws ApiException
* if fails to make API call
*/
public List listYubikeyOtpTokens(String after, String expand, String filter,
Boolean forDownload, Integer limit, String sortBy, String sortOrder) throws ApiException {
return this.listYubikeyOtpTokens(after, expand, filter, forDownload, limit, sortBy, sortOrder,
Collections.emptyMap());
}
/**
* List all YubiKey OTP Tokens Lists all YubiKey OTP Tokens
*
* @param after
* Specifies the pagination cursor for the next page of tokens (optional)
* @param expand
* Embeds the [User](/openapi/okta-management/management/tag/User/) resource if the YubiKey Token is
* assigned to a user and `expand` is set to `user` (optional)
* @param filter
* The expression used to filter tokens (optional)
* @param forDownload
* Returns tokens in a CSV to download instead of in the response. When you use this query parameter, the
* `limit` default changes to 1000. (optional, default to false)
* @param limit
* Specifies the number of results per page (optional, default to 20)
* @param sortBy
* The value of how the tokens are sorted (optional)
* @param sortOrder
* Specifies the sort order, either `ASC` or `DESC` (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return List<UserFactorYubikeyOtpToken>
*
* @throws ApiException
* if fails to make API call
*/
public List listYubikeyOtpTokens(String after, String expand, String filter,
Boolean forDownload, Integer limit, String sortBy, String sortOrder, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/org/factors/yubikey_token/tokens";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarQueryParams.addAll(apiClient.parameterToPair("expand", expand));
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
localVarQueryParams.addAll(apiClient.parameterToPair("forDownload", forDownload));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPair("sortBy", sortBy));
localVarQueryParams.addAll(apiClient.parameterToPair("sortOrder", sortOrder));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference> localVarReturnType = new TypeReference>() {
};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Resend a Factor enrollment Resends an `sms`, `call`, or `email` factor challenge as
* part of an enrollment flow For `call` and `sms` factors, Okta enforces a rate limit of one
* OTP challenge per device every 30 seconds. You can configure your `sms` and `call` factors to
* use a third-party telephony provider. See the [Telephony inline hook
* reference](https://developer.okta.com/docs/reference/telephony-hook/). Okta round-robins between SMS providers
* with every resend request to help ensure delivery of an SMS and Call OTPs across different carriers. >
* **Note**: Resend operations aren't allowed after a factor exceeds the activation rate limit. See [Activate a
* Factor](./#tag/UserFactor/operation/activateFactor).
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param resendUserFactor
* (required)
* @param templateId
* ID of an existing custom SMS template. See the [SMS Templates API](../Template). This parameter is
* only used by `sms` Factors. (optional)
*
* @return ResendUserFactor
*
* @throws ApiException
* if fails to make API call
*/
public ResendUserFactor resendEnrollFactor(String userId, String factorId, ResendUserFactor resendUserFactor,
String templateId) throws ApiException {
return this.resendEnrollFactor(userId, factorId, resendUserFactor, templateId, Collections.emptyMap());
}
/**
* Resend a Factor enrollment Resends an `sms`, `call`, or `email` factor challenge as
* part of an enrollment flow For `call` and `sms` factors, Okta enforces a rate limit of one
* OTP challenge per device every 30 seconds. You can configure your `sms` and `call` factors to
* use a third-party telephony provider. See the [Telephony inline hook
* reference](https://developer.okta.com/docs/reference/telephony-hook/). Okta round-robins between SMS providers
* with every resend request to help ensure delivery of an SMS and Call OTPs across different carriers. >
* **Note**: Resend operations aren't allowed after a factor exceeds the activation rate limit. See [Activate a
* Factor](./#tag/UserFactor/operation/activateFactor).
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param resendUserFactor
* (required)
* @param templateId
* ID of an existing custom SMS template. See the [SMS Templates API](../Template). This parameter is
* only used by `sms` Factors. (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return ResendUserFactor
*
* @throws ApiException
* if fails to make API call
*/
public ResendUserFactor resendEnrollFactor(String userId, String factorId, ResendUserFactor resendUserFactor,
String templateId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = resendUserFactor;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling resendEnrollFactor");
}
// verify the required parameter 'factorId' is set
if (factorId == null) {
throw new ApiException(400, "Missing the required parameter 'factorId' when calling resendEnrollFactor");
}
// verify the required parameter 'resendUserFactor' is set
if (resendUserFactor == null) {
throw new ApiException(400,
"Missing the required parameter 'resendUserFactor' when calling resendEnrollFactor");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors/{factorId}/resend"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()))
.replaceAll("\\{" + "factorId" + "\\}", apiClient.escapeString(factorId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("templateId", templateId));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Unenroll a Factor Unenrolls an existing Factor for the specified user. This allows the user to enroll a new
* Factor. > **Note**: If you unenroll the `push` or the `signed_nonce` Factors, Okta also
* unenrolls any other `totp`, `signed_nonce`, or Okta Verify `push` Factors
* associated with the user.
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param removeRecoveryEnrollment
* If `true`, removes the phone number as both a recovery method and a Factor. This parameter
* is only used for the `sms` and `call` Factors. (optional, default to false)
*
* @throws ApiException
* if fails to make API call
*/
public void unenrollFactor(String userId, String factorId, Boolean removeRecoveryEnrollment) throws ApiException {
this.unenrollFactor(userId, factorId, removeRecoveryEnrollment, Collections.emptyMap());
}
/**
* Unenroll a Factor Unenrolls an existing Factor for the specified user. This allows the user to enroll a new
* Factor. > **Note**: If you unenroll the `push` or the `signed_nonce` Factors, Okta also
* unenrolls any other `totp`, `signed_nonce`, or Okta Verify `push` Factors
* associated with the user.
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param removeRecoveryEnrollment
* If `true`, removes the phone number as both a recovery method and a Factor. This parameter
* is only used for the `sms` and `call` Factors. (optional, default to false)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void unenrollFactor(String userId, String factorId, Boolean removeRecoveryEnrollment,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling unenrollFactor");
}
// verify the required parameter 'factorId' is set
if (factorId == null) {
throw new ApiException(400, "Missing the required parameter 'factorId' when calling unenrollFactor");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors/{factorId}"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()))
.replaceAll("\\{" + "factorId" + "\\}", apiClient.escapeString(factorId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("removeRecoveryEnrollment", removeRecoveryEnrollment));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Upload a YubiKey OTP Seed Uploads a seed for a user to enroll a YubiKey OTP
*
* @param uploadYubikeyOtpTokenSeedRequest
* (required)
* @param after
* Specifies the pagination cursor for the next page of tokens (optional)
* @param expand
* Embeds the [User](/openapi/okta-management/management/tag/User/) resource if the YubiKey Token is
* assigned to a user and `expand` is set to `user` (optional)
* @param filter
* The expression used to filter tokens (optional)
* @param forDownload
* Returns tokens in a CSV to download instead of in the response. When you use this query parameter, the
* `limit` default changes to 1000. (optional, default to false)
* @param limit
* Specifies the number of results per page (optional, default to 20)
* @param sortBy
* The value of how the tokens are sorted (optional)
* @param sortOrder
* Specifies the sort order, either `ASC` or `DESC` (optional)
*
* @return UserFactorYubikeyOtpToken
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorYubikeyOtpToken uploadYubikeyOtpTokenSeed(
UploadYubikeyOtpTokenSeedRequest uploadYubikeyOtpTokenSeedRequest, String after, String expand,
String filter, Boolean forDownload, Integer limit, String sortBy, String sortOrder) throws ApiException {
return this.uploadYubikeyOtpTokenSeed(uploadYubikeyOtpTokenSeedRequest, after, expand, filter, forDownload,
limit, sortBy, sortOrder, Collections.emptyMap());
}
/**
* Upload a YubiKey OTP Seed Uploads a seed for a user to enroll a YubiKey OTP
*
* @param uploadYubikeyOtpTokenSeedRequest
* (required)
* @param after
* Specifies the pagination cursor for the next page of tokens (optional)
* @param expand
* Embeds the [User](/openapi/okta-management/management/tag/User/) resource if the YubiKey Token is
* assigned to a user and `expand` is set to `user` (optional)
* @param filter
* The expression used to filter tokens (optional)
* @param forDownload
* Returns tokens in a CSV to download instead of in the response. When you use this query parameter, the
* `limit` default changes to 1000. (optional, default to false)
* @param limit
* Specifies the number of results per page (optional, default to 20)
* @param sortBy
* The value of how the tokens are sorted (optional)
* @param sortOrder
* Specifies the sort order, either `ASC` or `DESC` (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserFactorYubikeyOtpToken
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorYubikeyOtpToken uploadYubikeyOtpTokenSeed(
UploadYubikeyOtpTokenSeedRequest uploadYubikeyOtpTokenSeedRequest, String after, String expand,
String filter, Boolean forDownload, Integer limit, String sortBy, String sortOrder,
Map additionalHeaders) throws ApiException {
Object localVarPostBody = uploadYubikeyOtpTokenSeedRequest;
// verify the required parameter 'uploadYubikeyOtpTokenSeedRequest' is set
if (uploadYubikeyOtpTokenSeedRequest == null) {
throw new ApiException(400,
"Missing the required parameter 'uploadYubikeyOtpTokenSeedRequest' when calling uploadYubikeyOtpTokenSeed");
}
// create path and map variables
String localVarPath = "/api/v1/org/factors/yubikey_token/tokens";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("after", after));
localVarQueryParams.addAll(apiClient.parameterToPair("expand", expand));
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
localVarQueryParams.addAll(apiClient.parameterToPair("forDownload", forDownload));
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPair("sortBy", sortBy));
localVarQueryParams.addAll(apiClient.parameterToPair("sortOrder", sortOrder));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Verify a Factor Verifies an OTP for a Factor. Some Factors (`call`, `email`,
* `push`, `sms`, `u2f`, and `webauthn`) must first issue a challenge before
* you can verify the Factor. Do this by making a request without a body. After a challenge is issued, make another
* request to verify the Factor. **Note**: To verify a `push` factor, use the **poll** link returned when
* you issue the challenge. See [Retrieve a Factor Transaction
* Status](/openapi/okta-management/management/tag/UserFactor/#tag/UserFactor/operation/getFactorTransactionStatus).
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param templateId
* ID of an existing custom SMS template. See the [SMS Templates API](../Template). This parameter is
* only used by `sms` Factors. (optional)
* @param tokenLifetimeSeconds
* Defines how long the token remains valid (optional, default to 300)
* @param xForwardedFor
* Public IP address for the user agent (optional)
* @param userAgent
* Type of user agent detected when the request is made. Required to verify `push` Factors.
* (optional)
* @param acceptLanguage
* An ISO 639-1 two-letter language code that defines a localized message to send. This parameter is only
* used by `sms` Factors. If a localized message doesn't exist or the
* `templateId` is incorrect, the default template is used instead. (optional)
* @param body
* Some Factors (`call`, `email`, `push`, `sms`, `u2f`,
* and `webauthn`) must first issue a challenge before you can verify the Factor. Do this by
* making a request without a body. After a challenge is issued, make another request to verify the
* Factor. (optional)
*
* @return UserFactorVerifyResponse
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorVerifyResponse verifyFactor(String userId, String factorId, String templateId,
Integer tokenLifetimeSeconds, String xForwardedFor, String userAgent, String acceptLanguage,
UserFactorVerifyRequest body) throws ApiException {
return this.verifyFactor(userId, factorId, templateId, tokenLifetimeSeconds, xForwardedFor, userAgent,
acceptLanguage, body, Collections.emptyMap());
}
/**
* Verify a Factor Verifies an OTP for a Factor. Some Factors (`call`, `email`,
* `push`, `sms`, `u2f`, and `webauthn`) must first issue a challenge before
* you can verify the Factor. Do this by making a request without a body. After a challenge is issued, make another
* request to verify the Factor. **Note**: To verify a `push` factor, use the **poll** link returned when
* you issue the challenge. See [Retrieve a Factor Transaction
* Status](/openapi/okta-management/management/tag/UserFactor/#tag/UserFactor/operation/getFactorTransactionStatus).
*
* @param userId
* ID of an existing Okta user (required)
* @param factorId
* ID of an existing user Factor (required)
* @param templateId
* ID of an existing custom SMS template. See the [SMS Templates API](../Template). This parameter is
* only used by `sms` Factors. (optional)
* @param tokenLifetimeSeconds
* Defines how long the token remains valid (optional, default to 300)
* @param xForwardedFor
* Public IP address for the user agent (optional)
* @param userAgent
* Type of user agent detected when the request is made. Required to verify `push` Factors.
* (optional)
* @param acceptLanguage
* An ISO 639-1 two-letter language code that defines a localized message to send. This parameter is only
* used by `sms` Factors. If a localized message doesn't exist or the
* `templateId` is incorrect, the default template is used instead. (optional)
* @param body
* Some Factors (`call`, `email`, `push`, `sms`, `u2f`,
* and `webauthn`) must first issue a challenge before you can verify the Factor. Do this by
* making a request without a body. After a challenge is issued, make another request to verify the
* Factor. (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserFactorVerifyResponse
*
* @throws ApiException
* if fails to make API call
*/
public UserFactorVerifyResponse verifyFactor(String userId, String factorId, String templateId,
Integer tokenLifetimeSeconds, String xForwardedFor, String userAgent, String acceptLanguage,
UserFactorVerifyRequest body, Map additionalHeaders) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling verifyFactor");
}
// verify the required parameter 'factorId' is set
if (factorId == null) {
throw new ApiException(400, "Missing the required parameter 'factorId' when calling verifyFactor");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/factors/{factorId}/verify"
.replaceAll("\\{" + "userId" + "\\}", apiClient.escapeString(userId.toString()))
.replaceAll("\\{" + "factorId" + "\\}", apiClient.escapeString(factorId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("templateId", templateId));
localVarQueryParams.addAll(apiClient.parameterToPair("tokenLifetimeSeconds", tokenLifetimeSeconds));
if (xForwardedFor != null)
localVarHeaderParams.put("X-Forwarded-For", apiClient.parameterToString(xForwardedFor));
if (userAgent != null)
localVarHeaderParams.put("User-Agent", apiClient.parameterToString(userAgent));
if (acceptLanguage != null)
localVarHeaderParams.put("Accept-Language", apiClient.parameterToString(acceptLanguage));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { "application/json" };
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy