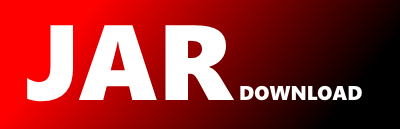
com.okta.sdk.resource.api.UserLifecycleApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.model.*;
import com.okta.sdk.resource.client.Pair;
import com.okta.sdk.resource.model.Error;
import com.okta.sdk.resource.model.UserActivationToken;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.DeserializationFeature;
import org.openapitools.jackson.nullable.JsonNullableModule;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class UserLifecycleApi {
private ApiClient apiClient;
public UserLifecycleApi() {
this(Configuration.getDefaultApiClient());
}
public UserLifecycleApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Activate a User Activates a User. This operation can only be performed on Users with a `STAGED` or
* `DEPROVISIONED` status. Activation of a User is an asynchronous operation. * The User will have the
* `transitioningToStatus` property with an `ACTIVE` value during activation to indicate that
* the user hasn't completed the asynchronous operation. * The User will have an `ACTIVE` status when
* the activation process completes. Users who don't have a password must complete the welcome flow by visiting
* the activation link to complete the transition to `ACTIVE` status. > **Note:** If you want to send a
* branded User Activation email, change the subdomain of your request to the custom domain that's associated
* with the brand. > For example, change `subdomain.okta.com` to `custom.domain.one`. See
* [Multibrand and custom domains](https://developer.okta.com/docs/concepts/brands/#multibrand-and-custom-domains).
* > **Note:** If you have Optional Password enabled, visiting the activation link is optional for users who
* aren't required to enroll a password. > See [Create user with Optional Password
* enabled](https://developer.okta.com/docs/reference/api/users/#create-user-with-optional-password-enabled). >
* **Legal disclaimer** > After a user is added to the Okta directory, they receive an activation email. As part
* of signing up for this service, > you agreed not to use Okta's service/product to spam and/or send
* unsolicited messages. > Please refrain from adding unrelated accounts to the directory as Okta is not
* responsible for, and disclaims any and all > liability associated with, the activation email's content.
* You, and you alone, bear responsibility for the emails sent to any recipients.
*
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* Sends an activation email to the user if `true` (optional, default to true)
*
* @return UserActivationToken
*
* @throws ApiException
* if fails to make API call
*/
public UserActivationToken activateUser(String userId, Boolean sendEmail) throws ApiException {
return this.activateUser(userId, sendEmail, Collections.emptyMap());
}
/**
* Activate a User Activates a User. This operation can only be performed on Users with a `STAGED` or
* `DEPROVISIONED` status. Activation of a User is an asynchronous operation. * The User will have the
* `transitioningToStatus` property with an `ACTIVE` value during activation to indicate that
* the user hasn't completed the asynchronous operation. * The User will have an `ACTIVE` status when
* the activation process completes. Users who don't have a password must complete the welcome flow by visiting
* the activation link to complete the transition to `ACTIVE` status. > **Note:** If you want to send a
* branded User Activation email, change the subdomain of your request to the custom domain that's associated
* with the brand. > For example, change `subdomain.okta.com` to `custom.domain.one`. See
* [Multibrand and custom domains](https://developer.okta.com/docs/concepts/brands/#multibrand-and-custom-domains).
* > **Note:** If you have Optional Password enabled, visiting the activation link is optional for users who
* aren't required to enroll a password. > See [Create user with Optional Password
* enabled](https://developer.okta.com/docs/reference/api/users/#create-user-with-optional-password-enabled). >
* **Legal disclaimer** > After a user is added to the Okta directory, they receive an activation email. As part
* of signing up for this service, > you agreed not to use Okta's service/product to spam and/or send
* unsolicited messages. > Please refrain from adding unrelated accounts to the directory as Okta is not
* responsible for, and disclaims any and all > liability associated with, the activation email's content.
* You, and you alone, bear responsibility for the emails sent to any recipients.
*
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* Sends an activation email to the user if `true` (optional, default to true)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserActivationToken
*
* @throws ApiException
* if fails to make API call
*/
public UserActivationToken activateUser(String userId, Boolean sendEmail, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling activateUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/activate".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("sendEmail", sendEmail));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Deactivate a User Deactivates a User. This operation can only be performed on Users that do not have a
* `DEPROVISIONED` status. * The User's `transitioningToStatus` property is
* `DEPROVISIONED` during deactivation to indicate that the user hasn't completed the asynchronous
* operation. * The User's status is `DEPROVISIONED` when the deactivation process is complete. >
* **Important:** Deactivating a User is a **destructive** operation. The User is deprovisioned from all assigned
* apps, which might destroy their data such as email or files. **This action cannot be recovered!** You can also
* perform user deactivation asynchronously. To invoke asynchronous user deactivation, pass an HTTP header
* `Prefer: respond-async` with the request.
*
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* Sends a deactivation email to the admin if `true` (optional, default to false)
* @param prefer
* Request asynchronous processing (optional)
*
* @throws ApiException
* if fails to make API call
*/
public void deactivateUser(String userId, Boolean sendEmail, String prefer) throws ApiException {
this.deactivateUser(userId, sendEmail, prefer, Collections.emptyMap());
}
/**
* Deactivate a User Deactivates a User. This operation can only be performed on Users that do not have a
* `DEPROVISIONED` status. * The User's `transitioningToStatus` property is
* `DEPROVISIONED` during deactivation to indicate that the user hasn't completed the asynchronous
* operation. * The User's status is `DEPROVISIONED` when the deactivation process is complete. >
* **Important:** Deactivating a User is a **destructive** operation. The User is deprovisioned from all assigned
* apps, which might destroy their data such as email or files. **This action cannot be recovered!** You can also
* perform user deactivation asynchronously. To invoke asynchronous user deactivation, pass an HTTP header
* `Prefer: respond-async` with the request.
*
* @param userId
* ID of an existing Okta user (required)
* @param sendEmail
* Sends a deactivation email to the admin if `true` (optional, default to false)
* @param prefer
* Request asynchronous processing (optional)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void deactivateUser(String userId, Boolean sendEmail, String prefer, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling deactivateUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/deactivate".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("sendEmail", sendEmail));
if (prefer != null)
localVarHeaderParams.put("Prefer", apiClient.parameterToString(prefer));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Reactivate a User Reactivates a user. This operation can only be performed on Users with a
* `PROVISIONED` or `RECOVERY`
* [status](/openapi/okta-management/management/tag/User/#tag/User/operation/listUsers!c=200&path=status&t=response).
* This operation restarts the activation workflow if for some reason the user activation wasn't completed when
* using the `activationToken` from [Activate
* User](/openapi/okta-management/management/tag/UserLifecycle/#tag/UserLifecycle/operation/activateUser). Users
* that don't have a password must complete the flow by completing [Reset
* Password](/openapi/okta-management/management/tag/UserCred/#tag/UserCred/operation/resetPassword) and MFA
* enrollment steps to transition the user to `ACTIVE` status. If `sendEmail` is
* `false`, returns an activation link for the user to set up their account. The activation token can be
* used to create a custom activation link.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param sendEmail
* Sends an activation email to the user if `true` (optional, default to false)
*
* @return UserActivationToken
*
* @throws ApiException
* if fails to make API call
*/
public UserActivationToken reactivateUser(String id, Boolean sendEmail) throws ApiException {
return this.reactivateUser(id, sendEmail, Collections.emptyMap());
}
/**
* Reactivate a User Reactivates a user. This operation can only be performed on Users with a
* `PROVISIONED` or `RECOVERY`
* [status](/openapi/okta-management/management/tag/User/#tag/User/operation/listUsers!c=200&path=status&t=response).
* This operation restarts the activation workflow if for some reason the user activation wasn't completed when
* using the `activationToken` from [Activate
* User](/openapi/okta-management/management/tag/UserLifecycle/#tag/UserLifecycle/operation/activateUser). Users
* that don't have a password must complete the flow by completing [Reset
* Password](/openapi/okta-management/management/tag/UserCred/#tag/UserCred/operation/resetPassword) and MFA
* enrollment steps to transition the user to `ACTIVE` status. If `sendEmail` is
* `false`, returns an activation link for the user to set up their account. The activation token can be
* used to create a custom activation link.
*
* @param id
* `id`, `login`, or `login shortname` (as long as it is unambiguous) of
* user (required)
* @param sendEmail
* Sends an activation email to the user if `true` (optional, default to false)
* @param additionalHeaders
* additionalHeaders for this call
*
* @return UserActivationToken
*
* @throws ApiException
* if fails to make API call
*/
public UserActivationToken reactivateUser(String id, Boolean sendEmail, Map additionalHeaders)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling reactivateUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{id}/lifecycle/reactivate".replaceAll("\\{" + "id" + "\\}",
apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("sendEmail", sendEmail));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
TypeReference localVarReturnType = new TypeReference() {
};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Reset Factors Resets all factors for the specified User. All MFA factor enrollments return to the unenrolled
* state. The User's status remains `ACTIVE`. This link is present only if the User is currently
* enrolled in one or more MFA factors.
*
* @param userId
* ID of an existing Okta user (required)
*
* @throws ApiException
* if fails to make API call
*/
public void resetFactors(String userId) throws ApiException {
this.resetFactors(userId, Collections.emptyMap());
}
/**
* Reset Factors Resets all factors for the specified User. All MFA factor enrollments return to the unenrolled
* state. The User's status remains `ACTIVE`. This link is present only if the User is currently
* enrolled in one or more MFA factors.
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void resetFactors(String userId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling resetFactors");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/reset_factors".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Suspend a User Suspends a user. This operation can only be performed on Users with an `ACTIVE` status.
* The User has a `SUSPENDED` status when the process completes. Suspended users can't sign in to
* Okta. They can only be unsuspended or deactivated. Their group and app assignments are retained.
*
* @param userId
* ID of an existing Okta user (required)
*
* @throws ApiException
* if fails to make API call
*/
public void suspendUser(String userId) throws ApiException {
this.suspendUser(userId, Collections.emptyMap());
}
/**
* Suspend a User Suspends a user. This operation can only be performed on Users with an `ACTIVE` status.
* The User has a `SUSPENDED` status when the process completes. Suspended users can't sign in to
* Okta. They can only be unsuspended or deactivated. Their group and app assignments are retained.
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void suspendUser(String userId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling suspendUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/suspend".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Unlock a User Unlocks a User with a `LOCKED_OUT` status or unlocks a User with an `ACTIVE`
* status that is blocked from unknown devices. Unlocked Users have an `ACTIVE` status and can sign in
* with their current password. > **Note:** This operation works with Okta-sourced users. It doesn't support
* directory-sourced accounts such as Active Directory.
*
* @param userId
* ID of an existing Okta user (required)
*
* @throws ApiException
* if fails to make API call
*/
public void unlockUser(String userId) throws ApiException {
this.unlockUser(userId, Collections.emptyMap());
}
/**
* Unlock a User Unlocks a User with a `LOCKED_OUT` status or unlocks a User with an `ACTIVE`
* status that is blocked from unknown devices. Unlocked Users have an `ACTIVE` status and can sign in
* with their current password. > **Note:** This operation works with Okta-sourced users. It doesn't support
* directory-sourced accounts such as Active Directory.
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void unlockUser(String userId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling unlockUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/unlock".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Unsuspend a User Unsuspends a user and returns them to the `ACTIVE` state. This operation can only be
* performed on users that have a `SUSPENDED` status.
*
* @param userId
* ID of an existing Okta user (required)
*
* @throws ApiException
* if fails to make API call
*/
public void unsuspendUser(String userId) throws ApiException {
this.unsuspendUser(userId, Collections.emptyMap());
}
/**
* Unsuspend a User Unsuspends a user and returns them to the `ACTIVE` state. This operation can only be
* performed on users that have a `SUSPENDED` status.
*
* @param userId
* ID of an existing Okta user (required)
* @param additionalHeaders
* additionalHeaders for this call
*
* @throws ApiException
* if fails to make API call
*/
public void unsuspendUser(String userId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'userId' is set
if (userId == null) {
throw new ApiException(400, "Missing the required parameter 'userId' when calling unsuspendUser");
}
// create path and map variables
String localVarPath = "/api/v1/users/{userId}/lifecycle/unsuspend".replaceAll("\\{" + "userId" + "\\}",
apiClient.escapeString(userId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = { "application/json" };
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiToken", "oauth2" };
apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(), localVarPostBody, localVarHeaderParams, localVarCookieParams,
localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
protected static ObjectMapper getObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
objectMapper.registerModule(new JsonNullableModule());
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_AS_NULL, true);
return objectMapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy