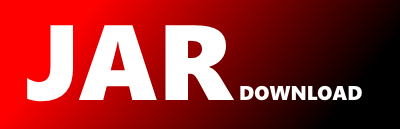
com.okta.sdk.resource.client.BaseApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
/*
* Okta Admin Management
* Allows customers to easily access the Okta Management APIs
*
* The version of the OpenAPI document: 2024.08.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.okta.sdk.resource.client;
import com.fasterxml.jackson.core.type.TypeReference;
import java.util.Collections;
import java.util.Map;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public abstract class BaseApi {
protected ApiClient apiClient;
public BaseApi() {
this(Configuration.getDefaultApiClient());
}
public BaseApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Directly invoke the API for the given URL. Useful if the API returns direct links/URLs for subsequent requests.
*
* @param url
* The URL for the request, either full URL or only the path.
* @param method
* The HTTP method for the request.
*
* @throws ApiException
* if fails to make API call.
*/
public void invokeAPI(String url, String method) throws ApiException {
invokeAPI(url, method, null, null, Collections.emptyMap());
}
/**
* Directly invoke the API for the given URL. Useful if the API returns direct links/URLs for subsequent requests.
*
* @param url
* The URL for the request, either full URL or only the path.
* @param method
* The HTTP method for the request.
* @param additionalHeaders
* Additional headers for the request.
*
* @throws ApiException
* if fails to make API call.
*/
public void invokeAPI(String url, String method, Map additionalHeaders) throws ApiException {
invokeAPI(url, method, null, null, additionalHeaders);
}
/**
* Directly invoke the API for the given URL. Useful if the API returns direct links/URLs for subsequent requests.
*
* @param url
* The URL for the request, either full URL or only the path.
* @param method
* The HTTP method for the request.
* @param request
* The request object.
*
* @throws ApiException
* if fails to make API call.
*/
public void invokeAPI(String url, String method, Object request) throws ApiException {
invokeAPI(url, method, request, null, Collections.emptyMap());
}
/**
* Directly invoke the API for the given URL. Useful if the API returns direct links/URLs for subsequent requests.
*
* @param url
* The URL for the request, either full URL or only the path.
* @param method
* The HTTP method for the request.
* @param request
* The request object.
* @param additionalHeaders
* Additional headers for the request.
*
* @throws ApiException
* if fails to make API call.
*/
public void invokeAPI(String url, String method, Object request, Map additionalHeaders)
throws ApiException {
invokeAPI(url, method, request, null, additionalHeaders);
}
/**
* Directly invoke the API for the given URL. Useful if the API returns direct links/URLs for subsequent requests.
*
* @param url
* The URL for the request, either full URL or only the path.
* @param method
* The HTTP method for the request.
* @param returnType
* The return type.
*
* @return The API response in the specified type.
*
* @throws ApiException
* if fails to make API call.
*/
public T invokeAPI(String url, String method, TypeReference returnType) throws ApiException {
return invokeAPI(url, method, null, returnType, Collections.emptyMap());
}
/**
* Directly invoke the API for the given URL. Useful if the API returns direct links/URLs for subsequent requests.
*
* @param url
* The URL for the request, either full URL or only the path.
* @param method
* The HTTP method for the request.
* @param request
* The request object.
* @param returnType
* The return type.
*
* @return The API response in the specified type.
*
* @throws ApiException
* if fails to make API call.
*/
public T invokeAPI(String url, String method, Object request, TypeReference returnType) throws ApiException {
return invokeAPI(url, method, request, returnType, Collections.emptyMap());
}
/**
* Directly invoke the API for the given URL. Useful if the API returns direct links/URLs for subsequent requests.
*
* @param url
* The URL for the request, either full URL or only the path.
* @param method
* The HTTP method for the request.
* @param request
* The request object.
* @param returnType
* The return type.
* @param additionalHeaders
* Additional headers for the request.
*
* @return The API response in the specified type.
*
* @throws ApiException
* if fails to make API call.
*/
public abstract T invokeAPI(String url, String method, Object request, TypeReference returnType,
Map additionalHeaders) throws ApiException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy