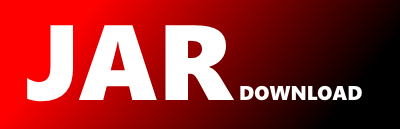
com.okta.sdk.resource.model.AgentPoolUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.Agent;
import com.okta.sdk.resource.model.AgentType;
import com.okta.sdk.resource.model.AgentUpdateJobStatus;
import com.okta.sdk.resource.model.AutoUpdateSchedule;
import com.okta.sdk.resource.model.LinksSelf;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Various information about agent auto update configuration
*/
@ApiModel(description = "Various information about agent auto update configuration")
@JsonPropertyOrder({ AgentPoolUpdate.JSON_PROPERTY_AGENTS, AgentPoolUpdate.JSON_PROPERTY_AGENT_TYPE,
AgentPoolUpdate.JSON_PROPERTY_ENABLED, AgentPoolUpdate.JSON_PROPERTY_ID, AgentPoolUpdate.JSON_PROPERTY_NAME,
AgentPoolUpdate.JSON_PROPERTY_NOTIFY_ADMIN, AgentPoolUpdate.JSON_PROPERTY_REASON,
AgentPoolUpdate.JSON_PROPERTY_SCHEDULE, AgentPoolUpdate.JSON_PROPERTY_SORT_ORDER,
AgentPoolUpdate.JSON_PROPERTY_STATUS, AgentPoolUpdate.JSON_PROPERTY_TARGET_VERSION,
AgentPoolUpdate.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class AgentPoolUpdate implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_AGENTS = "agents";
private List agents = null;
public static final String JSON_PROPERTY_AGENT_TYPE = "agentType";
private AgentType agentType;
public static final String JSON_PROPERTY_ENABLED = "enabled";
private Boolean enabled;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_NOTIFY_ADMIN = "notifyAdmin";
private Boolean notifyAdmin;
public static final String JSON_PROPERTY_REASON = "reason";
private String reason;
public static final String JSON_PROPERTY_SCHEDULE = "schedule";
private AutoUpdateSchedule schedule;
public static final String JSON_PROPERTY_SORT_ORDER = "sortOrder";
private Integer sortOrder;
public static final String JSON_PROPERTY_STATUS = "status";
private AgentUpdateJobStatus status;
public static final String JSON_PROPERTY_TARGET_VERSION = "targetVersion";
private String targetVersion;
public static final String JSON_PROPERTY_LINKS = "_links";
private LinksSelf links;
public AgentPoolUpdate() {
}
/*
* @JsonCreator public AgentPoolUpdate(
*
* @JsonProperty(JSON_PROPERTY_ID) String id ) { this(); this.id = id; }
*/
public AgentPoolUpdate agents(List agents) {
this.agents = agents;
return this;
}
public AgentPoolUpdate addagentsItem(Agent agentsItem) {
if (this.agents == null) {
this.agents = new ArrayList<>();
}
this.agents.add(agentsItem);
return this;
}
/**
* Get agents
*
* @return agents
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AGENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAgents() {
return agents;
}
@JsonProperty(JSON_PROPERTY_AGENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAgents(List agents) {
this.agents = agents;
}
public AgentPoolUpdate agentType(AgentType agentType) {
this.agentType = agentType;
return this;
}
/**
* Get agentType
*
* @return agentType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AGENT_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AgentType getAgentType() {
return agentType;
}
@JsonProperty(JSON_PROPERTY_AGENT_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAgentType(AgentType agentType) {
this.agentType = agentType;
}
public AgentPoolUpdate enabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Get enabled
*
* @return enabled
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENABLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getEnabled() {
return enabled;
}
@JsonProperty(JSON_PROPERTY_ENABLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
/**
* Get id
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
public AgentPoolUpdate name(String name) {
this.name = name;
return this;
}
/**
* Get name
*
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public AgentPoolUpdate notifyAdmin(Boolean notifyAdmin) {
this.notifyAdmin = notifyAdmin;
return this;
}
/**
* Get notifyAdmin
*
* @return notifyAdmin
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_NOTIFY_ADMIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getNotifyAdmin() {
return notifyAdmin;
}
@JsonProperty(JSON_PROPERTY_NOTIFY_ADMIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNotifyAdmin(Boolean notifyAdmin) {
this.notifyAdmin = notifyAdmin;
}
public AgentPoolUpdate reason(String reason) {
this.reason = reason;
return this;
}
/**
* Get reason
*
* @return reason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_REASON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getReason() {
return reason;
}
@JsonProperty(JSON_PROPERTY_REASON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReason(String reason) {
this.reason = reason;
}
public AgentPoolUpdate schedule(AutoUpdateSchedule schedule) {
this.schedule = schedule;
return this;
}
/**
* Get schedule
*
* @return schedule
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SCHEDULE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AutoUpdateSchedule getSchedule() {
return schedule;
}
@JsonProperty(JSON_PROPERTY_SCHEDULE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSchedule(AutoUpdateSchedule schedule) {
this.schedule = schedule;
}
public AgentPoolUpdate sortOrder(Integer sortOrder) {
this.sortOrder = sortOrder;
return this;
}
/**
* Get sortOrder
*
* @return sortOrder
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SORT_ORDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getSortOrder() {
return sortOrder;
}
@JsonProperty(JSON_PROPERTY_SORT_ORDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSortOrder(Integer sortOrder) {
this.sortOrder = sortOrder;
}
public AgentPoolUpdate status(AgentUpdateJobStatus status) {
this.status = status;
return this;
}
/**
* Get status
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AgentUpdateJobStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatus(AgentUpdateJobStatus status) {
this.status = status;
}
public AgentPoolUpdate targetVersion(String targetVersion) {
this.targetVersion = targetVersion;
return this;
}
/**
* Get targetVersion
*
* @return targetVersion
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TARGET_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTargetVersion() {
return targetVersion;
}
@JsonProperty(JSON_PROPERTY_TARGET_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTargetVersion(String targetVersion) {
this.targetVersion = targetVersion;
}
public AgentPoolUpdate links(LinksSelf links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksSelf getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(LinksSelf links) {
this.links = links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AgentPoolUpdate agentPoolUpdate = (AgentPoolUpdate) o;
return Objects.equals(this.agents, agentPoolUpdate.agents)
&& Objects.equals(this.agentType, agentPoolUpdate.agentType)
&& Objects.equals(this.enabled, agentPoolUpdate.enabled) && Objects.equals(this.id, agentPoolUpdate.id)
&& Objects.equals(this.name, agentPoolUpdate.name)
&& Objects.equals(this.notifyAdmin, agentPoolUpdate.notifyAdmin)
&& Objects.equals(this.reason, agentPoolUpdate.reason)
&& Objects.equals(this.schedule, agentPoolUpdate.schedule)
&& Objects.equals(this.sortOrder, agentPoolUpdate.sortOrder)
&& Objects.equals(this.status, agentPoolUpdate.status)
&& Objects.equals(this.targetVersion, agentPoolUpdate.targetVersion)
&& Objects.equals(this.links, agentPoolUpdate.links);
// ;
}
@Override
public int hashCode() {
return Objects.hash(agents, agentType, enabled, id, name, notifyAdmin, reason, schedule, sortOrder, status,
targetVersion, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AgentPoolUpdate {\n");
sb.append(" agents: ").append(toIndentedString(agents)).append("\n");
sb.append(" agentType: ").append(toIndentedString(agentType)).append("\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" notifyAdmin: ").append(toIndentedString(notifyAdmin)).append("\n");
sb.append(" reason: ").append(toIndentedString(reason)).append("\n");
sb.append(" schedule: ").append(toIndentedString(schedule)).append("\n");
sb.append(" sortOrder: ").append(toIndentedString(sortOrder)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" targetVersion: ").append(toIndentedString(targetVersion)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy