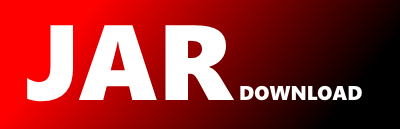
com.okta.sdk.resource.model.AgentPoolUpdateSetting Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.AgentType;
import com.okta.sdk.resource.model.ReleaseChannel;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Setting for auto-update
*/
@ApiModel(description = "Setting for auto-update")
@JsonPropertyOrder({ AgentPoolUpdateSetting.JSON_PROPERTY_AGENT_TYPE,
AgentPoolUpdateSetting.JSON_PROPERTY_CONTINUE_ON_ERROR, AgentPoolUpdateSetting.JSON_PROPERTY_LATEST_VERSION,
AgentPoolUpdateSetting.JSON_PROPERTY_MINIMAL_SUPPORTED_VERSION, AgentPoolUpdateSetting.JSON_PROPERTY_POOL_ID,
AgentPoolUpdateSetting.JSON_PROPERTY_POOL_NAME, AgentPoolUpdateSetting.JSON_PROPERTY_RELEASE_CHANNEL })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class AgentPoolUpdateSetting implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_AGENT_TYPE = "agentType";
private AgentType agentType;
public static final String JSON_PROPERTY_CONTINUE_ON_ERROR = "continueOnError";
private Boolean continueOnError;
public static final String JSON_PROPERTY_LATEST_VERSION = "latestVersion";
private String latestVersion;
public static final String JSON_PROPERTY_MINIMAL_SUPPORTED_VERSION = "minimalSupportedVersion";
private String minimalSupportedVersion;
public static final String JSON_PROPERTY_POOL_ID = "poolId";
private String poolId;
public static final String JSON_PROPERTY_POOL_NAME = "poolName";
private String poolName;
public static final String JSON_PROPERTY_RELEASE_CHANNEL = "releaseChannel";
private ReleaseChannel releaseChannel;
public AgentPoolUpdateSetting() {
}
/*
* @JsonCreator public AgentPoolUpdateSetting(
*
* @JsonProperty(JSON_PROPERTY_POOL_ID) String poolId ) { this(); this.poolId = poolId; }
*/
public AgentPoolUpdateSetting agentType(AgentType agentType) {
this.agentType = agentType;
return this;
}
/**
* Get agentType
*
* @return agentType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AGENT_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AgentType getAgentType() {
return agentType;
}
@JsonProperty(JSON_PROPERTY_AGENT_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAgentType(AgentType agentType) {
this.agentType = agentType;
}
public AgentPoolUpdateSetting continueOnError(Boolean continueOnError) {
this.continueOnError = continueOnError;
return this;
}
/**
* Get continueOnError
*
* @return continueOnError
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CONTINUE_ON_ERROR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getContinueOnError() {
return continueOnError;
}
@JsonProperty(JSON_PROPERTY_CONTINUE_ON_ERROR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setContinueOnError(Boolean continueOnError) {
this.continueOnError = continueOnError;
}
public AgentPoolUpdateSetting latestVersion(String latestVersion) {
this.latestVersion = latestVersion;
return this;
}
/**
* Get latestVersion
*
* @return latestVersion
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LATEST_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getLatestVersion() {
return latestVersion;
}
@JsonProperty(JSON_PROPERTY_LATEST_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLatestVersion(String latestVersion) {
this.latestVersion = latestVersion;
}
public AgentPoolUpdateSetting minimalSupportedVersion(String minimalSupportedVersion) {
this.minimalSupportedVersion = minimalSupportedVersion;
return this;
}
/**
* Get minimalSupportedVersion
*
* @return minimalSupportedVersion
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_MINIMAL_SUPPORTED_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMinimalSupportedVersion() {
return minimalSupportedVersion;
}
@JsonProperty(JSON_PROPERTY_MINIMAL_SUPPORTED_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMinimalSupportedVersion(String minimalSupportedVersion) {
this.minimalSupportedVersion = minimalSupportedVersion;
}
/**
* Get poolId
*
* @return poolId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_POOL_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPoolId() {
return poolId;
}
public AgentPoolUpdateSetting poolName(String poolName) {
this.poolName = poolName;
return this;
}
/**
* Get poolName
*
* @return poolName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_POOL_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPoolName() {
return poolName;
}
@JsonProperty(JSON_PROPERTY_POOL_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPoolName(String poolName) {
this.poolName = poolName;
}
public AgentPoolUpdateSetting releaseChannel(ReleaseChannel releaseChannel) {
this.releaseChannel = releaseChannel;
return this;
}
/**
* Get releaseChannel
*
* @return releaseChannel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_RELEASE_CHANNEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ReleaseChannel getReleaseChannel() {
return releaseChannel;
}
@JsonProperty(JSON_PROPERTY_RELEASE_CHANNEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReleaseChannel(ReleaseChannel releaseChannel) {
this.releaseChannel = releaseChannel;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AgentPoolUpdateSetting agentPoolUpdateSetting = (AgentPoolUpdateSetting) o;
return Objects.equals(this.agentType, agentPoolUpdateSetting.agentType)
&& Objects.equals(this.continueOnError, agentPoolUpdateSetting.continueOnError)
&& Objects.equals(this.latestVersion, agentPoolUpdateSetting.latestVersion)
&& Objects.equals(this.minimalSupportedVersion, agentPoolUpdateSetting.minimalSupportedVersion)
&& Objects.equals(this.poolId, agentPoolUpdateSetting.poolId)
&& Objects.equals(this.poolName, agentPoolUpdateSetting.poolName)
&& Objects.equals(this.releaseChannel, agentPoolUpdateSetting.releaseChannel);
// ;
}
@Override
public int hashCode() {
return Objects.hash(agentType, continueOnError, latestVersion, minimalSupportedVersion, poolId, poolName,
releaseChannel);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AgentPoolUpdateSetting {\n");
sb.append(" agentType: ").append(toIndentedString(agentType)).append("\n");
sb.append(" continueOnError: ").append(toIndentedString(continueOnError)).append("\n");
sb.append(" latestVersion: ").append(toIndentedString(latestVersion)).append("\n");
sb.append(" minimalSupportedVersion: ").append(toIndentedString(minimalSupportedVersion)).append("\n");
sb.append(" poolId: ").append(toIndentedString(poolId)).append("\n");
sb.append(" poolName: ").append(toIndentedString(poolName)).append("\n");
sb.append(" releaseChannel: ").append(toIndentedString(releaseChannel)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy