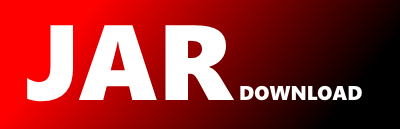
com.okta.sdk.resource.model.AppUserAssignRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.AppUserCredentials;
import com.okta.sdk.resource.model.AppUserStatus;
import com.okta.sdk.resource.model.AppUserSyncState;
import com.okta.sdk.resource.model.LinksAppAndUser;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.Map;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* AppUserAssignRequest
*/
@JsonPropertyOrder({ AppUserAssignRequest.JSON_PROPERTY_CREATED, AppUserAssignRequest.JSON_PROPERTY_CREDENTIALS,
AppUserAssignRequest.JSON_PROPERTY_EXTERNAL_ID, AppUserAssignRequest.JSON_PROPERTY_ID,
AppUserAssignRequest.JSON_PROPERTY_LAST_SYNC, AppUserAssignRequest.JSON_PROPERTY_LAST_UPDATED,
AppUserAssignRequest.JSON_PROPERTY_PASSWORD_CHANGED, AppUserAssignRequest.JSON_PROPERTY_PROFILE,
AppUserAssignRequest.JSON_PROPERTY_SCOPE, AppUserAssignRequest.JSON_PROPERTY_STATUS,
AppUserAssignRequest.JSON_PROPERTY_STATUS_CHANGED, AppUserAssignRequest.JSON_PROPERTY_SYNC_STATE,
AppUserAssignRequest.JSON_PROPERTY_EMBEDDED, AppUserAssignRequest.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class AppUserAssignRequest implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_CREDENTIALS = "credentials";
private AppUserCredentials credentials;
public static final String JSON_PROPERTY_EXTERNAL_ID = "externalId";
private String externalId;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_LAST_SYNC = "lastSync";
private OffsetDateTime lastSync;
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private OffsetDateTime lastUpdated;
public static final String JSON_PROPERTY_PASSWORD_CHANGED = "passwordChanged";
private JsonNullable passwordChanged = JsonNullable. undefined();
public static final String JSON_PROPERTY_PROFILE = "profile";
private Map profile = null;
/**
* Indicates if the assignment is direct (`USER`) or by group membership (`GROUP`).
*/
public enum ScopeEnum {
USER("USER"),
GROUP("GROUP"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ScopeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ScopeEnum fromValue(String value) {
for (ScopeEnum b : ScopeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_SCOPE = "scope";
private ScopeEnum scope;
public static final String JSON_PROPERTY_STATUS = "status";
private AppUserStatus status;
public static final String JSON_PROPERTY_STATUS_CHANGED = "statusChanged";
private OffsetDateTime statusChanged;
public static final String JSON_PROPERTY_SYNC_STATE = "syncState";
private AppUserSyncState syncState;
public static final String JSON_PROPERTY_EMBEDDED = "_embedded";
private Map embedded = null;
public static final String JSON_PROPERTY_LINKS = "_links";
private LinksAppAndUser links;
public AppUserAssignRequest() {
}
/*
* @JsonCreator public AppUserAssignRequest(
*
* @JsonProperty(JSON_PROPERTY_EXTERNAL_ID) String externalId,
*
* @JsonProperty(JSON_PROPERTY_LAST_SYNC) OffsetDateTime lastSync,
*
* @JsonProperty(JSON_PROPERTY_PASSWORD_CHANGED) OffsetDateTime passwordChanged,
*
* @JsonProperty(JSON_PROPERTY_STATUS_CHANGED) OffsetDateTime statusChanged,
*
* @JsonProperty(JSON_PROPERTY_EMBEDDED) Map embedded ) { this(); this.externalId = externalId;
* this.lastSync = lastSync; this.passwordChanged = passwordChanged; this.statusChanged = statusChanged;
* this.embedded = embedded; }
*/
public AppUserAssignRequest created(OffsetDateTime created) {
this.created = created;
return this;
}
/**
* Get created
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCreated(OffsetDateTime created) {
this.created = created;
}
public AppUserAssignRequest credentials(AppUserCredentials credentials) {
this.credentials = credentials;
return this;
}
/**
* Get credentials
*
* @return credentials
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CREDENTIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AppUserCredentials getCredentials() {
return credentials;
}
@JsonProperty(JSON_PROPERTY_CREDENTIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCredentials(AppUserCredentials credentials) {
this.credentials = credentials;
}
/**
* The ID of the user in the target app that's linked to the Okta Application User object. This value is the
* native app-specific identifier or primary key for the user in the target app. The `externalId` is set
* during import when the user is confirmed (reconciled) or during provisioning when the user is created in the
* target app. This value isn't populated for SSO app assignments (for example, SAML or SWA) because it
* isn't synchronized with a target app.
*
* @return externalId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "70c14cc17d3745e8a9f98d599a68329c", value = "The ID of the user in the target app that's linked to the Okta Application User object. This value is the native app-specific identifier or primary key for the user in the target app. The `externalId` is set during import when the user is confirmed (reconciled) or during provisioning when the user is created in the target app. This value isn't populated for SSO app assignments (for example, SAML or SWA) because it isn't synchronized with a target app.")
@JsonProperty(JSON_PROPERTY_EXTERNAL_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExternalId() {
return externalId;
}
public AppUserAssignRequest id(String id) {
this.id = id;
return this;
}
/**
* Unique identifier for the Okta User
*
* @return id
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "00u11z6WHMYCGPCHCRFK", required = true, value = "Unique identifier for the Okta User")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
/**
* Timestamp of the last synchronization operation. This value is only updated for apps with the
* `IMPORT_PROFILE_UPDATES` or `PUSH PROFILE_UPDATES` feature.
*
* @return lastSync
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2014-06-24T15:27:59Z", value = "Timestamp of the last synchronization operation. This value is only updated for apps with the `IMPORT_PROFILE_UPDATES` or `PUSH PROFILE_UPDATES` feature.")
@JsonProperty(JSON_PROPERTY_LAST_SYNC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastSync() {
return lastSync;
}
public AppUserAssignRequest lastUpdated(OffsetDateTime lastUpdated) {
this.lastUpdated = lastUpdated;
return this;
}
/**
* Get lastUpdated
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLastUpdated(OffsetDateTime lastUpdated) {
this.lastUpdated = lastUpdated;
}
/**
* Timestamp when the Application User password was last changed
*
* @return passwordChanged
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2014-06-24T15:27:59Z", value = "Timestamp when the Application User password was last changed")
@JsonIgnore
public OffsetDateTime getPasswordChanged() {
if (passwordChanged == null) {
passwordChanged = JsonNullable. undefined();
}
return passwordChanged.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PASSWORD_CHANGED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getPasswordChanged_JsonNullable() {
return passwordChanged;
}
@JsonProperty(JSON_PROPERTY_PASSWORD_CHANGED)
private void setPasswordChanged_JsonNullable(JsonNullable passwordChanged) {
this.passwordChanged = passwordChanged;
}
public AppUserAssignRequest profile(Map profile) {
this.profile = profile;
return this;
}
public AppUserAssignRequest putprofileItem(String key, Object profileItem) {
if (this.profile == null) {
this.profile = new HashMap<>();
}
this.profile.put(key, profileItem);
return this;
}
/**
* Specifies the default and custom profile properties for a user. Properties that are visible in the Admin Console
* for an app assignment can also be assigned through the API. Some properties are reference properties that are
* imported from the target app and can't be configured. See
* [profile](/openapi/okta-management/management/tag/User/#tag/User/operation/getUser!c=200&path=profile&t=response).
*
* @return profile
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the default and custom profile properties for a user. Properties that are visible in the Admin Console for an app assignment can also be assigned through the API. Some properties are reference properties that are imported from the target app and can't be configured. See [profile](/openapi/okta-management/management/tag/User/#tag/User/operation/getUser!c=200&path=profile&t=response). ")
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getProfile() {
return profile;
}
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public void setProfile(Map profile) {
this.profile = profile;
}
public AppUserAssignRequest scope(ScopeEnum scope) {
this.scope = scope;
return this;
}
/**
* Indicates if the assignment is direct (`USER`) or by group membership (`GROUP`).
*
* @return scope
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USER", value = "Indicates if the assignment is direct (`USER`) or by group membership (`GROUP`).")
@JsonProperty(JSON_PROPERTY_SCOPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ScopeEnum getScope() {
return scope;
}
@JsonProperty(JSON_PROPERTY_SCOPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setScope(ScopeEnum scope) {
this.scope = scope;
}
public AppUserAssignRequest status(AppUserStatus status) {
this.status = status;
return this;
}
/**
* Get status
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AppUserStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatus(AppUserStatus status) {
this.status = status;
}
/**
* Timestamp when the Application User status was last changed
*
* @return statusChanged
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2014-06-24T15:28:14Z", value = "Timestamp when the Application User status was last changed")
@JsonProperty(JSON_PROPERTY_STATUS_CHANGED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getStatusChanged() {
return statusChanged;
}
public AppUserAssignRequest syncState(AppUserSyncState syncState) {
this.syncState = syncState;
return this;
}
/**
* Get syncState
*
* @return syncState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SYNC_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AppUserSyncState getSyncState() {
return syncState;
}
@JsonProperty(JSON_PROPERTY_SYNC_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSyncState(AppUserSyncState syncState) {
this.syncState = syncState;
}
/**
* Embedded resources related to the Application User using the [JSON Hypertext Application
* Language](https://datatracker.ietf.org/doc/html/draft-kelly-json-hal-06) specification
*
* @return embedded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Embedded resources related to the Application User using the [JSON Hypertext Application Language](https://datatracker.ietf.org/doc/html/draft-kelly-json-hal-06) specification")
@JsonProperty(JSON_PROPERTY_EMBEDDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getEmbedded() {
return embedded;
}
public AppUserAssignRequest links(LinksAppAndUser links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksAppAndUser getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(LinksAppAndUser links) {
this.links = links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AppUserAssignRequest appUserAssignRequest = (AppUserAssignRequest) o;
return Objects.equals(this.created, appUserAssignRequest.created)
&& Objects.equals(this.credentials, appUserAssignRequest.credentials)
&& Objects.equals(this.externalId, appUserAssignRequest.externalId)
&& Objects.equals(this.id, appUserAssignRequest.id)
&& Objects.equals(this.lastSync, appUserAssignRequest.lastSync)
&& Objects.equals(this.lastUpdated, appUserAssignRequest.lastUpdated)
&& equalsNullable(this.passwordChanged, appUserAssignRequest.passwordChanged)
&& Objects.equals(this.profile, appUserAssignRequest.profile)
&& Objects.equals(this.scope, appUserAssignRequest.scope)
&& Objects.equals(this.status, appUserAssignRequest.status)
&& Objects.equals(this.statusChanged, appUserAssignRequest.statusChanged)
&& Objects.equals(this.syncState, appUserAssignRequest.syncState)
&& Objects.equals(this.embedded, appUserAssignRequest.embedded)
&& Objects.equals(this.links, appUserAssignRequest.links);
// ;
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(created, credentials, externalId, id, lastSync, lastUpdated,
hashCodeNullable(passwordChanged), profile, scope, status, statusChanged, syncState, embedded, links);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] { a.get() }) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AppUserAssignRequest {\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" credentials: ").append(toIndentedString(credentials)).append("\n");
sb.append(" externalId: ").append(toIndentedString(externalId)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" lastSync: ").append(toIndentedString(lastSync)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" passwordChanged: ").append(toIndentedString(passwordChanged)).append("\n");
sb.append(" profile: ").append(toIndentedString(profile)).append("\n");
sb.append(" scope: ").append(toIndentedString(scope)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" statusChanged: ").append(toIndentedString(statusChanged)).append("\n");
sb.append(" syncState: ").append(toIndentedString(syncState)).append("\n");
sb.append(" embedded: ").append(toIndentedString(embedded)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy