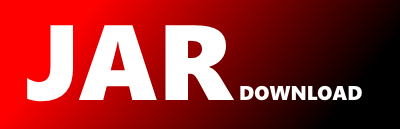
com.okta.sdk.resource.model.Application Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.ApplicationAccessibility;
import com.okta.sdk.resource.model.ApplicationEmbedded;
import com.okta.sdk.resource.model.ApplicationLicensing;
import com.okta.sdk.resource.model.ApplicationLifecycleStatus;
import com.okta.sdk.resource.model.ApplicationLinks;
import com.okta.sdk.resource.model.ApplicationSignOnMode;
import com.okta.sdk.resource.model.ApplicationVisibility;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Application
*/
@JsonPropertyOrder({ Application.JSON_PROPERTY_ACCESSIBILITY, Application.JSON_PROPERTY_CREATED,
Application.JSON_PROPERTY_FEATURES, Application.JSON_PROPERTY_ID, Application.JSON_PROPERTY_LABEL,
Application.JSON_PROPERTY_LAST_UPDATED, Application.JSON_PROPERTY_LICENSING, Application.JSON_PROPERTY_ORN,
Application.JSON_PROPERTY_PROFILE, Application.JSON_PROPERTY_SIGN_ON_MODE, Application.JSON_PROPERTY_STATUS,
Application.JSON_PROPERTY_VISIBILITY, Application.JSON_PROPERTY_EMBEDDED, Application.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.EXISTING_PROPERTY, property = "signOnMode", visible = true, defaultImpl = Application.class)
@JsonSubTypes({ @JsonSubTypes.Type(value = AutoLoginApplication.class, name = "AUTO_LOGIN"),
@JsonSubTypes.Type(value = BasicAuthApplication.class, name = "BASIC_AUTH"),
@JsonSubTypes.Type(value = BookmarkApplication.class, name = "BOOKMARK"),
@JsonSubTypes.Type(value = BrowserPluginApplication.class, name = "BROWSER_PLUGIN"),
@JsonSubTypes.Type(value = OpenIdConnectApplication.class, name = "OPENID_CONNECT"),
@JsonSubTypes.Type(value = Saml11Application.class, name = "SAML_1_1"),
@JsonSubTypes.Type(value = SamlApplication.class, name = "SAML_2_0"),
@JsonSubTypes.Type(value = SecurePasswordStoreApplication.class, name = "SECURE_PASSWORD_STORE"),
@JsonSubTypes.Type(value = WsFederationApplication.class, name = "WS_FEDERATION"), })
public class Application implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACCESSIBILITY = "accessibility";
private ApplicationAccessibility accessibility;
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
/**
* Gets or Sets features
*/
public enum FeaturesEnum {
GROUP_PUSH("GROUP_PUSH"),
IMPORT_NEW_USERS("IMPORT_NEW_USERS"),
IMPORT_PROFILE_UPDATES("IMPORT_PROFILE_UPDATES"),
IMPORT_USER_SCHEMA("IMPORT_USER_SCHEMA"),
PROFILE_MASTERING("PROFILE_MASTERING"),
PUSH_NEW_USERS("PUSH_NEW_USERS"),
PUSH_PASSWORD_UPDATES("PUSH_PASSWORD_UPDATES"),
PUSH_PROFILE_UPDATES("PUSH_PROFILE_UPDATES"),
PUSH_USER_DEACTIVATION("PUSH_USER_DEACTIVATION"),
REACTIVATE_USERS("REACTIVATE_USERS"),
OUTBOUND_DEL_AUTH("OUTBOUND_DEL_AUTH"),
DESKTOP_SSO("DESKTOP_SSO"),
FEDERATED_PROFILE("FEDERATED_PROFILE"),
SUPPRESS_ACTIVATION_EMAIL("SUPPRESS_ACTIVATION_EMAIL"),
PUSH_PENDING_USERS("PUSH_PENDING_USERS"),
MFA("MFA"),
UPDATE_EXISTING_USERNAME("UPDATE_EXISTING_USERNAME"),
EXCLUDE_USERNAME_UPDATE_ON_PROFILE_PUSH("EXCLUDE_USERNAME_UPDATE_ON_PROFILE_PUSH"),
EXCHANGE_ACTIVE_SYNC("EXCHANGE_ACTIVE_SYNC"),
IMPORT_SYNC("IMPORT_SYNC"),
IMPORT_SYNC_CONTACTS("IMPORT_SYNC_CONTACTS"),
DEVICE_COMPLIANCE("DEVICE_COMPLIANCE"),
VPN_CONFIG("VPN_CONFIG"),
IMPORT_SCHEMA_ENUM_VALUES("IMPORT_SCHEMA_ENUM_VALUES"),
SCIM_PROVISIONING("SCIM_PROVISIONING"),
DEVICE_FILTER_IN_SIGN_ON_RULES("DEVICE_FILTER_IN_SIGN_ON_RULES"),
PROFILE_TEMPLATE_UPGRADE("PROFILE_TEMPLATE_UPGRADE"),
DEFAULT_PUSH_STATUS_TO_PUSH("DEFAULT_PUSH_STATUS_TO_PUSH"),
REAL_TIME_SYNC("REAL_TIME_SYNC"),
SSO("SSO"),
AUTHN_CONTEXT("AUTHN_CONTEXT"),
JIT_PROVISIONING("JIT_PROVISIONING"),
GROUP_SYNC("GROUP_SYNC"),
OPP_SCIM_INCREMENTAL_IMPORTS("OPP_SCIM_INCREMENTAL_IMPORTS"),
IN_MEMORY_APP_USER("IN_MEMORY_APP_USER"),
LOG_STREAMING("LOG_STREAMING"),
OAUTH_INTEGRATION("OAUTH_INTEGRATION"),
IDP("IDP"),
PUSH_NEW_USERS_WITHOUT_PASSWORD("PUSH_NEW_USERS_WITHOUT_PASSWORD"),
SKYHOOK_SERVICE("SKYHOOK_SERVICE"),
ENTITLEMENT_MANAGEMENT("ENTITLEMENT_MANAGEMENT"),
PUSH_NEW_USERS_WITH_HASHED_PASSWORD("PUSH_NEW_USERS_WITH_HASHED_PASSWORD"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
FeaturesEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static FeaturesEnum fromValue(String value) {
for (FeaturesEnum b : FeaturesEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_FEATURES = "features";
private List features = null;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_LABEL = "label";
private String label;
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private OffsetDateTime lastUpdated;
public static final String JSON_PROPERTY_LICENSING = "licensing";
private ApplicationLicensing licensing;
public static final String JSON_PROPERTY_ORN = "orn";
private String orn;
public static final String JSON_PROPERTY_PROFILE = "profile";
private Map profile = null;
public static final String JSON_PROPERTY_SIGN_ON_MODE = "signOnMode";
protected ApplicationSignOnMode signOnMode;
public static final String JSON_PROPERTY_STATUS = "status";
private ApplicationLifecycleStatus status;
public static final String JSON_PROPERTY_VISIBILITY = "visibility";
private ApplicationVisibility visibility;
public static final String JSON_PROPERTY_EMBEDDED = "_embedded";
private ApplicationEmbedded embedded;
public static final String JSON_PROPERTY_LINKS = "_links";
private ApplicationLinks links;
public Application() {
}
/*
* @JsonCreator public Application(
*
* @JsonProperty(JSON_PROPERTY_CREATED) OffsetDateTime created,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED) OffsetDateTime lastUpdated,
*
* @JsonProperty(JSON_PROPERTY_ORN) String orn ) { this(); this.created = created; this.id = id; this.lastUpdated =
* lastUpdated; this.orn = orn; }
*/
public Application accessibility(ApplicationAccessibility accessibility) {
this.accessibility = accessibility;
return this;
}
/**
* Get accessibility
*
* @return accessibility
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACCESSIBILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationAccessibility getAccessibility() {
return accessibility;
}
@JsonProperty(JSON_PROPERTY_ACCESSIBILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccessibility(ApplicationAccessibility accessibility) {
this.accessibility = accessibility;
}
/**
* Timestamp when the Application object was created
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Timestamp when the Application object was created")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
public Application features(List features) {
this.features = features;
return this;
}
public Application addfeaturesItem(FeaturesEnum featuresItem) {
if (this.features == null) {
this.features = new ArrayList<>();
}
this.features.add(featuresItem);
return this;
}
/**
* Enabled app features > **Note:** Some apps can support optional provisioning features. See [Application
* Features](/openapi/okta-management/management/tag/ApplicationFeatures/)
*
* @return features
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Enabled app features > **Note:** Some apps can support optional provisioning features. See [Application Features](/openapi/okta-management/management/tag/ApplicationFeatures/) ")
@JsonProperty(JSON_PROPERTY_FEATURES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getFeatures() {
return features;
}
@JsonProperty(JSON_PROPERTY_FEATURES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFeatures(List features) {
this.features = features;
}
/**
* Unique ID for the app instance
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique ID for the app instance")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
public Application label(String label) {
this.label = label;
return this;
}
/**
* User-defined display name for app
*
* @return label
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "User-defined display name for app")
@JsonProperty(JSON_PROPERTY_LABEL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getLabel() {
return label;
}
@JsonProperty(JSON_PROPERTY_LABEL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLabel(String label) {
this.label = label;
}
/**
* Timestamp when the Application object was last updated
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Timestamp when the Application object was last updated")
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
public Application licensing(ApplicationLicensing licensing) {
this.licensing = licensing;
return this;
}
/**
* Get licensing
*
* @return licensing
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LICENSING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationLicensing getLicensing() {
return licensing;
}
@JsonProperty(JSON_PROPERTY_LICENSING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLicensing(ApplicationLicensing licensing) {
this.licensing = licensing;
}
/**
* The Okta resource name (ORN) for the current app instance
*
* @return orn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The Okta resource name (ORN) for the current app instance")
@JsonProperty(JSON_PROPERTY_ORN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOrn() {
return orn;
}
public Application profile(Map profile) {
this.profile = profile;
return this;
}
public Application putprofileItem(String key, Object profileItem) {
if (this.profile == null) {
this.profile = new HashMap<>();
}
this.profile.put(key, profileItem);
return this;
}
/**
* Contains any valid JSON schema for specifying properties that can be referenced from a request (only available to
* OAuth 2.0 client apps). For example, add an app manager contact email address or define an allowlist of groups
* that you can then reference using the Okta Expression Language `getFilteredGroups` function. >
* **Notes:** > * `profile` isn't encrypted, so don't store sensitive data in it. > *
* `profile` doesn't limit the level of nesting in the JSON schema you created, but there is a
* practical size limit. Okta recommends a JSON schema size of 1 MB or less for best performance.
*
* @return profile
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Contains any valid JSON schema for specifying properties that can be referenced from a request (only available to OAuth 2.0 client apps). For example, add an app manager contact email address or define an allowlist of groups that you can then reference using the Okta Expression Language `getFilteredGroups` function. > **Notes:** > * `profile` isn't encrypted, so don't store sensitive data in it. > * `profile` doesn't limit the level of nesting in the JSON schema you created, but there is a practical size limit. Okta recommends a JSON schema size of 1 MB or less for best performance.")
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getProfile() {
return profile;
}
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public void setProfile(Map profile) {
this.profile = profile;
}
public Application signOnMode(ApplicationSignOnMode signOnMode) {
this.signOnMode = signOnMode;
return this;
}
/**
* Get signOnMode
*
* @return signOnMode
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_SIGN_ON_MODE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public ApplicationSignOnMode getSignOnMode() {
return signOnMode;
}
@JsonProperty(JSON_PROPERTY_SIGN_ON_MODE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSignOnMode(ApplicationSignOnMode signOnMode) {
this.signOnMode = signOnMode;
}
public Application status(ApplicationLifecycleStatus status) {
this.status = status;
return this;
}
/**
* Get status
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationLifecycleStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatus(ApplicationLifecycleStatus status) {
this.status = status;
}
public Application visibility(ApplicationVisibility visibility) {
this.visibility = visibility;
return this;
}
/**
* Get visibility
*
* @return visibility
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationVisibility getVisibility() {
return visibility;
}
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVisibility(ApplicationVisibility visibility) {
this.visibility = visibility;
}
public Application embedded(ApplicationEmbedded embedded) {
this.embedded = embedded;
return this;
}
/**
* Get embedded
*
* @return embedded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_EMBEDDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationEmbedded getEmbedded() {
return embedded;
}
@JsonProperty(JSON_PROPERTY_EMBEDDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEmbedded(ApplicationEmbedded embedded) {
this.embedded = embedded;
}
public Application links(ApplicationLinks links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationLinks getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(ApplicationLinks links) {
this.links = links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Application application = (Application) o;
return Objects.equals(this.accessibility, application.accessibility)
&& Objects.equals(this.created, application.created)
&& Objects.equals(this.features, application.features) && Objects.equals(this.id, application.id)
&& Objects.equals(this.label, application.label)
&& Objects.equals(this.lastUpdated, application.lastUpdated)
&& Objects.equals(this.licensing, application.licensing) && Objects.equals(this.orn, application.orn)
&& Objects.equals(this.profile, application.profile)
&& Objects.equals(this.signOnMode, application.signOnMode)
&& Objects.equals(this.status, application.status)
&& Objects.equals(this.visibility, application.visibility)
&& Objects.equals(this.embedded, application.embedded) && Objects.equals(this.links, application.links);
// ;
}
@Override
public int hashCode() {
return Objects.hash(accessibility, created, features, id, label, lastUpdated, licensing, orn, profile,
signOnMode, status, visibility, embedded, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Application {\n");
sb.append(" accessibility: ").append(toIndentedString(accessibility)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" features: ").append(toIndentedString(features)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" label: ").append(toIndentedString(label)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" licensing: ").append(toIndentedString(licensing)).append("\n");
sb.append(" orn: ").append(toIndentedString(orn)).append("\n");
sb.append(" profile: ").append(toIndentedString(profile)).append("\n");
sb.append(" signOnMode: ").append(toIndentedString(signOnMode)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" visibility: ").append(toIndentedString(visibility)).append("\n");
sb.append(" embedded: ").append(toIndentedString(embedded)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy